Using Cloudinary for eCommerce
Nothing improves sales on the web than imagery and video. You can describe an item a dozen ways but one picture can suck the user right in, multiple photos (including a nice zoom feature) can clinch the sale. What does this mean? It's important to provide users with performant, detailed, and quality images for both desktop and mobile. Luckily Cloudinary provides a variety of optimization methods and media features so you can showcase your products in an optimized, customizable fashion.
Let's have a look at some of the features and methods Cloudinary provides for optimizing, creating, and delivering images in eCommerce sites!
Background Removal
Unless you're using a professional photographer, you're likely going to want touching up of your photos, but doing so takes time and expertise. Instead of throwing loads of time or money at background removal, upload the image to Cloudinary for background removal:
// npm install cloudinary var cloudinary = require('cloudinary'); // Set your API information cloudinary.config({ cloud_name: 'david-walsh-blog', api_key: '############', api_secret: '############' }); // Upload an image, cloudinary.uploader.upload('sample-photos/my-photo.jpg', function(result) { // Log out the result to get the URL of the image console.log(result); // Image url is: result.url / result.secure_url }, { public_id: "my-photo", background_removal: "remove_the_background" });
![]() |
![]() |
Taking the step to remove the photo background, if necessary, sets the stage for more transformations. Cleanup!
Easy, Dynamic Image Sizing by URL
The simplest image transformation feature is dynamic image sizing which can be done by slightly modifying the URL to an image:
<!-- Size image to 300x300 --> <img src="https://res.cloudinary.com/david-wash-blog/image/upload/w_300,h_300/usa">
If you're using Cloudinary's awesome React.js API, you can simply add the width
and height
attributes to the Image
and the image will be dynamically transformed to that size:
<Image publicId="ringo" width="300" height="300">
Customized image sizing optimizes both load time and rendering time!
Content Aware Cropping
Most sites prefer all product images to be cut to certain sizes, making displaying product images in templates easy and predictable. There will be times, however, that thumbnails need to be used and it would be nice if just the image was cropped to the main focal point. Cloudinary can do this with content aware cropping!
<img src="https://res.cloudinary.com/david-wash-blog/image/upload/g_auto/diana">
![]() |
![]() |
With Cloudinary's content aware cropping, you can look for a face or other focal point while cropping the image to size!
Layers
The layering transformation feature is one of the most amazing features from Cloudinary. Using the Cloudinary API, you can upload images which can overlay other images! Think of a price tag image on top of your product image, along with some price text:
<Image publicId="diana" width="400"> <Transformation raw_transformation="200" /> <Transformation angle="20" crop="scale" gravity="north_west" overlay="price-tag" width="100" /> <Transformation angle="20" color="rgb:fff" gravity="north_west" overlay="text:fira%20mono_16_bold:$$(price)" x="45" y="27" /> </Image>
The resulting <img>
and URL looks is:
https://res.cloudinary.com/david-wash-blog/image/upload/$price_!200!/a_20,c_scale,g_north_west,l_price-tag,w_100/a_20,co_rgb:fff,g_north_west,l_text:fira%20mono_16_bold:$$(price),x_45,y_27/diana
You can complete this layering with any other transformation, so you can overlay image and text on a content aware cropped image of a dynamic size. That's one of the really power functions of Cloudinary!
Client Hints
A few months back I showed you the newly supported client hints feature in browsers. Client hints allow your browser to share your viewport dimensions in requests so that servers can dynamically generate and return an optimized image:
<meta http-equiv="Accept-CH" content="DPR, Width">
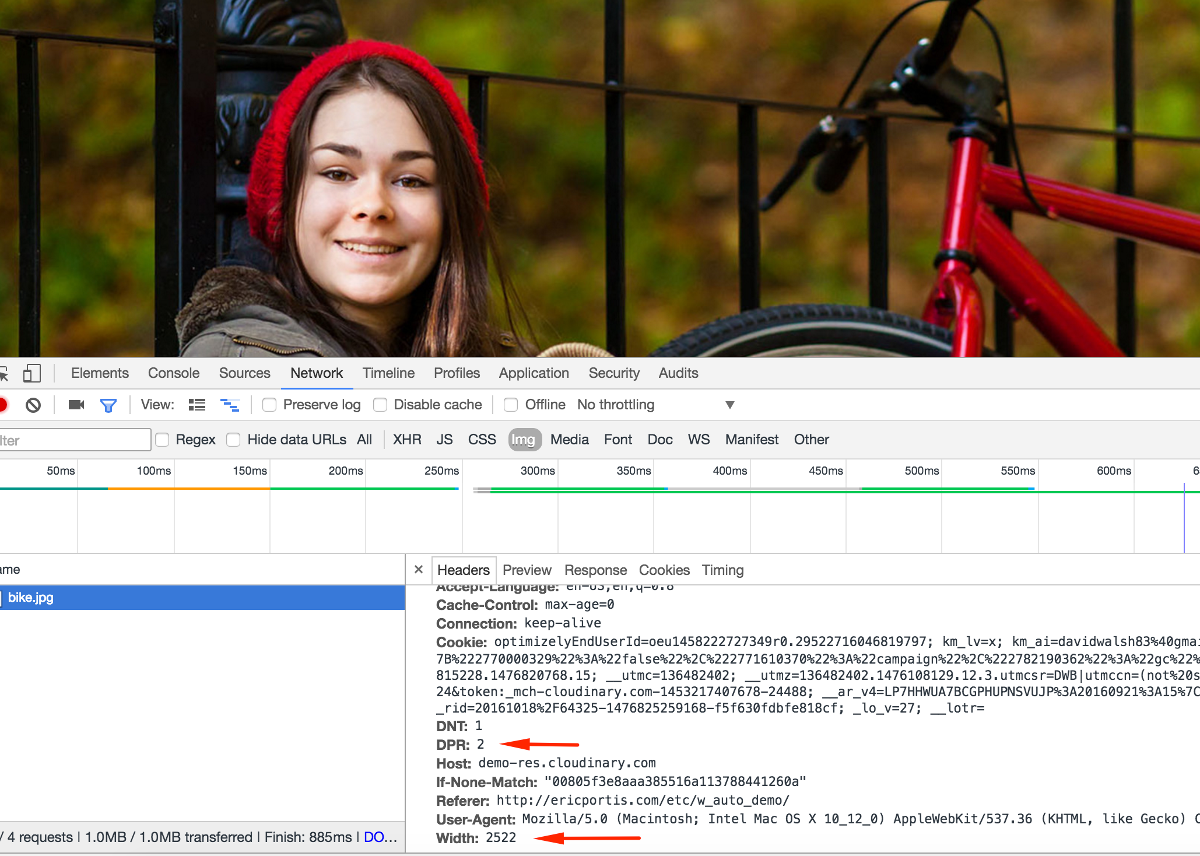
Cloudinary supports client hints so be sure to add the <meta>
tag to enable them!
Pulling it all Together!
Using the techniques cited above, along with a few others, I've created a crude single page product display and shopping cart using cloudinary-react:
import React, { Component } from 'react'; import './App.css'; import { Image, Transformation, CloudinaryContext } from 'cloudinary-react'; let products = [ { id: 'diana', title: 'Pricess Di', price: '200' }, { id: 'obama', title: 'President Obama', price: '150' }, { id: 'usa', title: 'Innauguration', price: '75' } ]; class App extends Component { state = { currentProduct: products[0], cart: [] }; addToCart = (publicId) => { this.state.cart.push(this.state.currentProduct); this.setState({ cart: this.state.cart }); } render() { return ( <div> <CloudinaryContext cloudName="david-wash-blog"> <div className="detail"> <h2>Product Detail</h2> <Image publicId={this.state.currentProduct.id} width="400"> <Transformation raw_transformation={`$price_!${this.state.currentProduct.price}!`} /> <Transformation angle="20" crop="scale" gravity="north_west" overlay="price-tag" width="100" /> <Transformation angle="20" color="rgb:fff" gravity="north_west" overlay="text:fira%20mono_16_bold:$$(price)" x="45" y="27" /> </Image> <button onClick={this.addToCart}>Add to Cart</button> </div> <div className="available"> <h2>Products in Stock</h2> <p>Click the thumbnail below to view a larger image.</p> {products.map(product => { return <Image key={product.id} onClick={() =>this.setState({ currentProduct: product })} publicId={product.id} width="200"/>; })} </div> <div className="cart"> <h2>Your Cart</h2> {this.state.cart.length ? this.state.cart.map(product => { return <Image key={product.id} gravity="auto" crop="crop" publicId={product.id} width="100"/>; }) : 'Cart is empty'} </div> </CloudinaryContext></div> ); } } export default App;
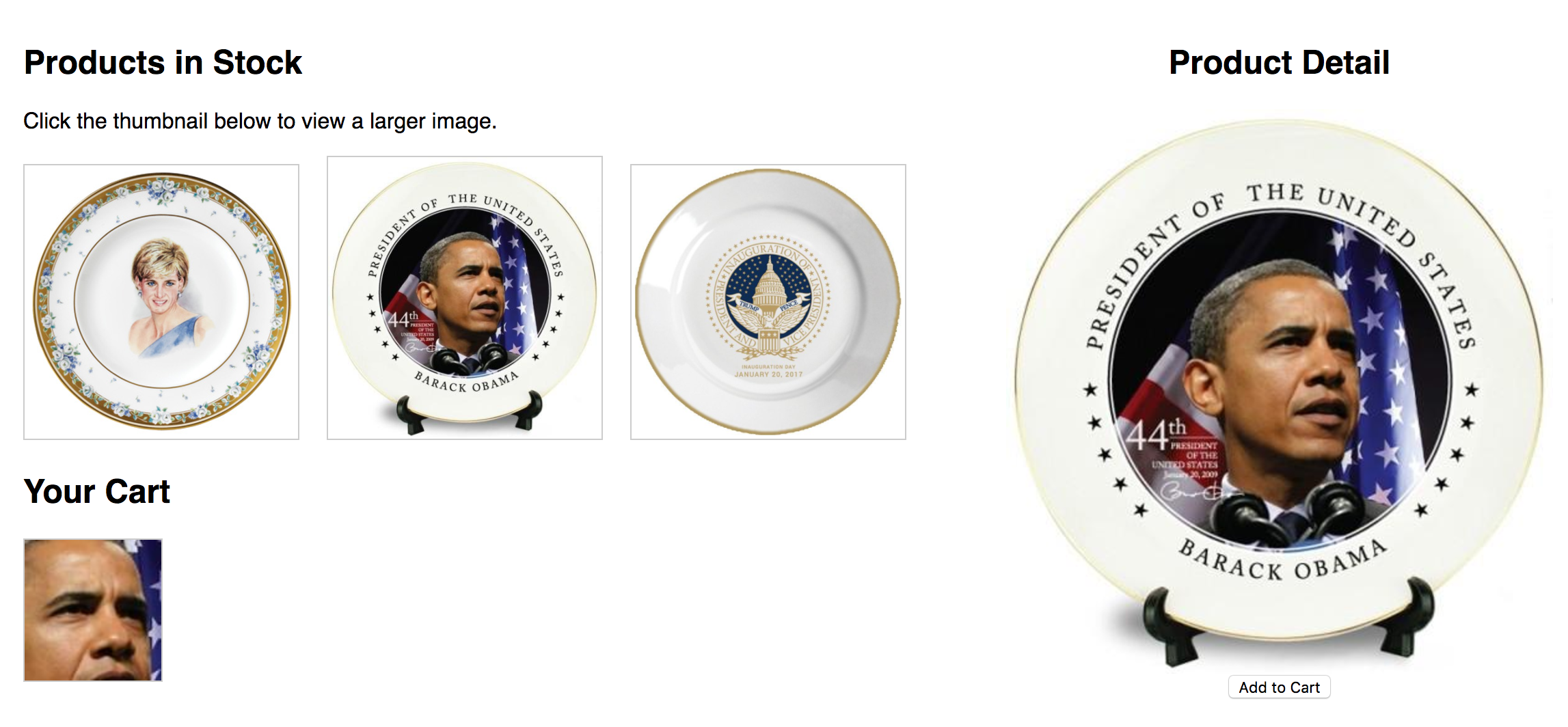
All images are sized dynamically, price overlays are added to product detail images, and any product added to cart will provide content aware images as thumbnails so that the thumbnail can be small but the user knows exactly the detail needed to make out what the item is.
While these techniques have been presented as awesome eCommerce feature usages, the truth is that each of these features can be used in any situation to improve performance and visibility. Don't forget that Cloudinary provides PHP, Node.js, Ruby, Java, Python, and other APIs to upload, modify, and display your images. Give Cloudinary a shot -- you wont be disappointed!
Wow, I will definitely have to do more with Cloudinary moving forward. Looks like it can do some really useful stuff. Thanks for the information!