Cloudinary React Components
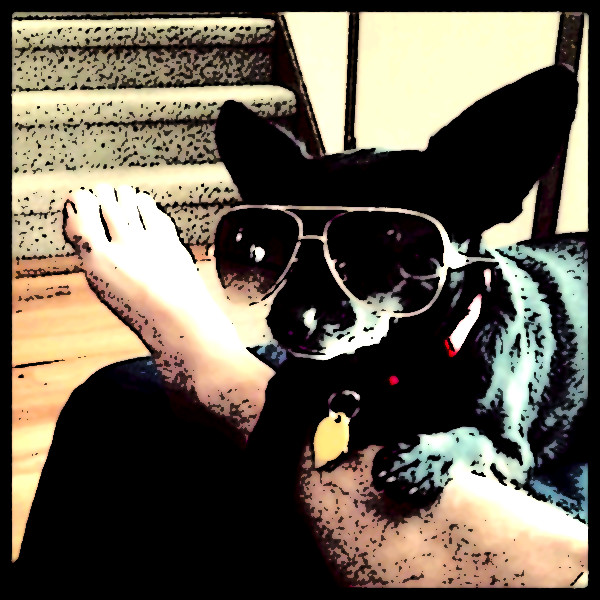
I've been experimenting a lot with both React and Cloudinary over the past six months and it's been a blast -- I'm learning a ton while also recovering the ambition and thirst I had as a young developer. React has been a revelation: an advanced JavaScript framework that doesn't overcomplicate things and has encouraged me to learn more ESNext and Babel. Cloudinary lets me do amazing stuff with images and media, like creating Instagram-Like Filters, transform images, and more, all by modifying the image URL. Cloudinary provides APIs for a number of different languages (Node.js, Python, PHP, etc.) but they've gone a step further and now offer a set of React components to use in your React app!
Installing cloudinary-react
Cloudinary's React component set is available via the cloudinary-react
name:
yarn add cloudinary-react # or `npm install cloudinary-react`
Use npm install
or yarn add
to get these components.
Cloudinary Components
Cloudinary provides CloudinaryContext
, Image
, Video
, and Transformation
components. They are available within your app using require
or import
:
import { Image, Video, Transformation, CloudinaryContext } from 'cloudinary-react';
Let's have a look at each component type!
Image
The Image
component is the simplest of components, allowing all types of transformations:
<!-- basic usage --> <Image cloudName="david-wash-blog" publicId="ringo" width="600" crop="scale" alt="Ringo" /> <!-- with a simple effect --> <Image publicId="ringo" effect="cartoonify:25:50" />
The code above transpiles to:
<img alt="Ringo" width="600" src="http://res.cloudinary.com/david-wash-blog/image/upload/c_scale,w_600/ringo">
Note that you can add all of the usual attributes for each image, like alt
, title
, and so on.
Video
The Video
component is also very simple, working as you think it would:
<Video cloudName="david-wash-blog" publicId="sample-video" width="800" controls />
All transformations can also be applied to videos as well!
Transformation
Image
components can contain any number of Transformation
components to modify the outgoing image:
<!-- Rotate and trim the image, then add text --> <Image cloudName="david-wash-blog" publicId="ringo"> <Transformation angle="-45"/> <Transformation effect="trim" angle="45" crop="scale" width="600"> <Transformation overlay="text:Arial_100:Hello" /> </Transformation> </Image>
Cloudinary's Transformation documentation is an excellent reference for the amazing breadth of transformations. If you have any question about how the transformation should be added as an attribute, click the Node.js
tab in the Cloudinary documentation examples to see what your keys and values should be.
Cloudinary Context
The CloudinaryContext
component allows for intelligent grouping of media and effects to be applied to its child content, be it Image
, Video
, Transformation
components:
<CloudinaryContext cloudName="david-wash-blog" effect="art:aurora" width="300"> <Image publicId="ringo"></Image> <Image publicId="coffee"></Image> <!-- ... --> </CloudinaryContext>
With the example above, all Image
components have the effect designated by its parent CloudinaryContext
, an awesome way to cut down on repeated code and keep your JSX tight and organized! You can even stack CloudinaryContext
components:
<CloudinaryContext cloudName="david-wash-blog"> <Image publicId="ringo" /> <Image publicId="coffee" /> <CloudinaryContext fetchFormat="auto" quality="auto"> <Image publicId="ringo" /> <Image publicId="coffee" /> </CloudinaryContext> </CloudinaryContext>
Creating a Quick Instagram-style Experiment
One of the reasons I love React (and more specifically create-react-app
) is that it lets me put together a dynamic app really quickly. Since Cloudinary provides a few dozen artistic filters, I thought it would be fun to create a very simple Instagram-like app using the Cloudinary's React library. Then minutes later I had something:
class App extends Component { state = { width: 600, filter: null }; filters = [ 'al_dente', 'audrey', 'aurora', 'daguerre', 'eucalyptus', 'fes', 'frost', 'hairspray', 'hokusai', 'incognito', 'linen', 'peacock', 'primavera', 'quartz', 'red_rock', 'refresh', 'sizzle', 'sonnet', 'ukulele', 'zorro' ]; onPreviewClick(event) { this.setState({ filter: event.target.src }); } render() { return ( <div> <CloudinaryContext cloudName="david-wash-blog"> <div className="wrapper"> <div className="left"> <Image publicId="ringo" width="{this.state.width}"> { this.state.filter && (<Transformation effect={`art:${this.state.filter}`} />) } </Image> </div> <div className="right"> {this.filters.map(filter => ( <div className="preview" key={filter}> <Image publicId="ringo" width="{this.state.width}" onClick={this.onPreviewClick}> <Transformation effect={`art:${filter}`} /> </Image> <span>{filter}</span> </div> ))} </div> </div> </CloudinaryContext> </div> ); } }
The result looks like:
Cloudinary provides APIs and helpers for every major programming language and now provides jQuery and React library resources to make coding your media-rich applications with ease. Especially useful are the Transformation
and CloudinaryContext
components which allow your code to stay clean and brief. cloudinary-react
is just another awesome reason to look to Cloudinary for all of your media needs!
“I’ve been experimenting a lot with both React and Cloudinary over the past six months and it’s been a blast — I’m learning a ton while also recovering the ambition and thirst I had as a young developer. React has been a revelation: an advanced JavaScript framework that doesn’t overcomplicate things and has encouraged me to learn more ESNext and Babel. ”
So crazy how this is the exact same experience I am going through – even the part about experimenting a lot with React and Cloudinary over the past SIX months. Lol. Nice article Dave. But more importantly I wanted to thank you for the article about being a dev dad. About to be one. Really needed that.
how to upload this its not mentioned