Sass Color Variables That Don’t Suck
One of the best reasons to use Sass is variables. They help keep your code DRY, which makes it easy to maintain and change. However, with colors, it's easy for your variables to get out of hand. In this article, I'll show you an quick, easy method to wrangle your color variables.
The Problem
Let's say you have the following CSS:
h1 { background-color: #b0eb00; } a { color: #b0eb00; } button { border-color: #b0eb00; box-shadow: 0 0 1rem #b0eb00; }
If you decide to use a different color than #b0eb00
, you have to change it in multiple places. This quickly becomes aggravating in a large project. But, with Sass, you can use a variable!
$yellow-green: #b0eb00; h1 { background-color: #b0eb00; } a { color: #b0eb00; } button { border-color: #b0eb00; box-shadow: 0 0 1rem #b0eb00; }
Now, it's easy to change the value of $yellow-green
everywhere. It's also simple to locate the places where $yellow-green
is used by searching for it.
As you accumulate color variables, you may decide to stick them in in their own file.
$yellow-green: #b0eb00; $gray: #595959; $dark-gray: #363636; $light-gray: #a6a6a6;
Over time, this file grows until you end up with a mess.
$yellow-green: #b0eb00; $red: #b30015; $blue: #2a00b3; $other-blue: #0077b3; $cyan: #00b0b3; $transparent-cyan: #24fbff; $periwinkle: #8a7dff; $light-gray: #a6a6a6; $gray: #595959; $darkish-gray: #444; $dark-gray: #363636; $darker-gray: #303030; $darkest-gray: #292929; $darkester-gray: #111;
Every time you add a new color, it becomes more difficult to find a name for it. Should #0c0c0c
be $darkesterest-gray
? How about $almost-black
? When using these variables, it's difficult to remember the names without looking them up.
A Bad Solution That Does Not Work
You've felt the pain of your color variables and decided to refactor. Instead of naming the variables after colors, now you're going to name them after how they're used.
$background-color: #b0eb00; $error-color: #b30015; $anchor-color: #2a00b3; $anchor-hover-color: #0077b3; $anchor-visited-color: #00b0b3; $anchor-visited-blending-color: #24fbff; $tooltip-color: #8a7dff; $emphasis-color: #a6a6a6; $text-color: #595959; $strong-color: #444; $username-color: #363636; $shadow-color: #303030; $border-color: #292929; $marquee-color: #111;
At least now it's obvious what the variables are by looking at them. It's also really easy to change your colors. The problem is the variables aren't reusable. What happens when decide to use #292929
as the background color for your buttons? Do you rename $border-color
to $border-and-button-color
? Do you add another variable and duplicate the value?
Let's say you want to change the anchor color. Rather than changing it in the anchor styles, where it's used, you have to search for $anchor-color
in the big list of color variables. Gross!
Another Solution That Doesn't Work
You've given up naming colors and decided to use numbers instead.
$color1: #b0eb00; $color2: #b30015; $color3: #2a00b3; $color4: #0077b3; $color5: #00b0b3; $color6: #24fbff; $color7: #8a7dff; $color8: #a6a6a6; $color9: #595959; $color10: #444; $color11: #363636; $color12: #303030; $color13: #292929; $color14: #111;
Now your variables are unique, but they're difficult to remember. Was the border color $color12
or $color13
? If you see $color4
in your code, can you tell what color it is without looking it up? What happens when you want to remove $color8
? Do you rename the subsequent colors or let your ordering get messed up?
A New Approach
Wouldn't it be nice if you could name your variables after colors like you were trying to do at the start, but without all the naming difficulty? You can using Name That Color! Using this tool, rename your color variables.
$lime: #b0eb00; $bright-red: #b30015; $dark-blue: #2a00b3; $deep-cerulean: #0077b3; $bondi-blue: #00b0b3; $cyan: #24fbff; $heliotrope: #8a7dff; $silver-chalice: #a6a6a6; $scorpion: #595959; $tundora: #444; $mine-shaft: #363636; $cod-gray: #111;
Now your variables are named after colors, but they're unique and memorable.
My favorite feature of Name That Color is it shows you the closest color when the hex code doesn't match a color exactly. This tool has more than 1500 color names, so it's easy to find names for all of your variables.
What about colors that are really close? For example, #303030
and #292929
would both be named $mine-shaft
. To keep your color variables from becoming too granular, if a color name is duplicated, then it's not worth having its own variable. Instead, use the built in Sass color functions. In the above example, you would use darken($mine-shaft, 2.5%)
instead of #303030
.
That's It!
You've mastered the art of simple color variable naming and you can move on with your life. Enjoy!
Edit: A few people have asked how to change a color using this methodology. For example, how would you swap $crimson
for $royal-blue
? Because your colors are uniquely named, it's easy to do a quick find and replace to swap them out. Every modern code editor supports this feature, so change away!
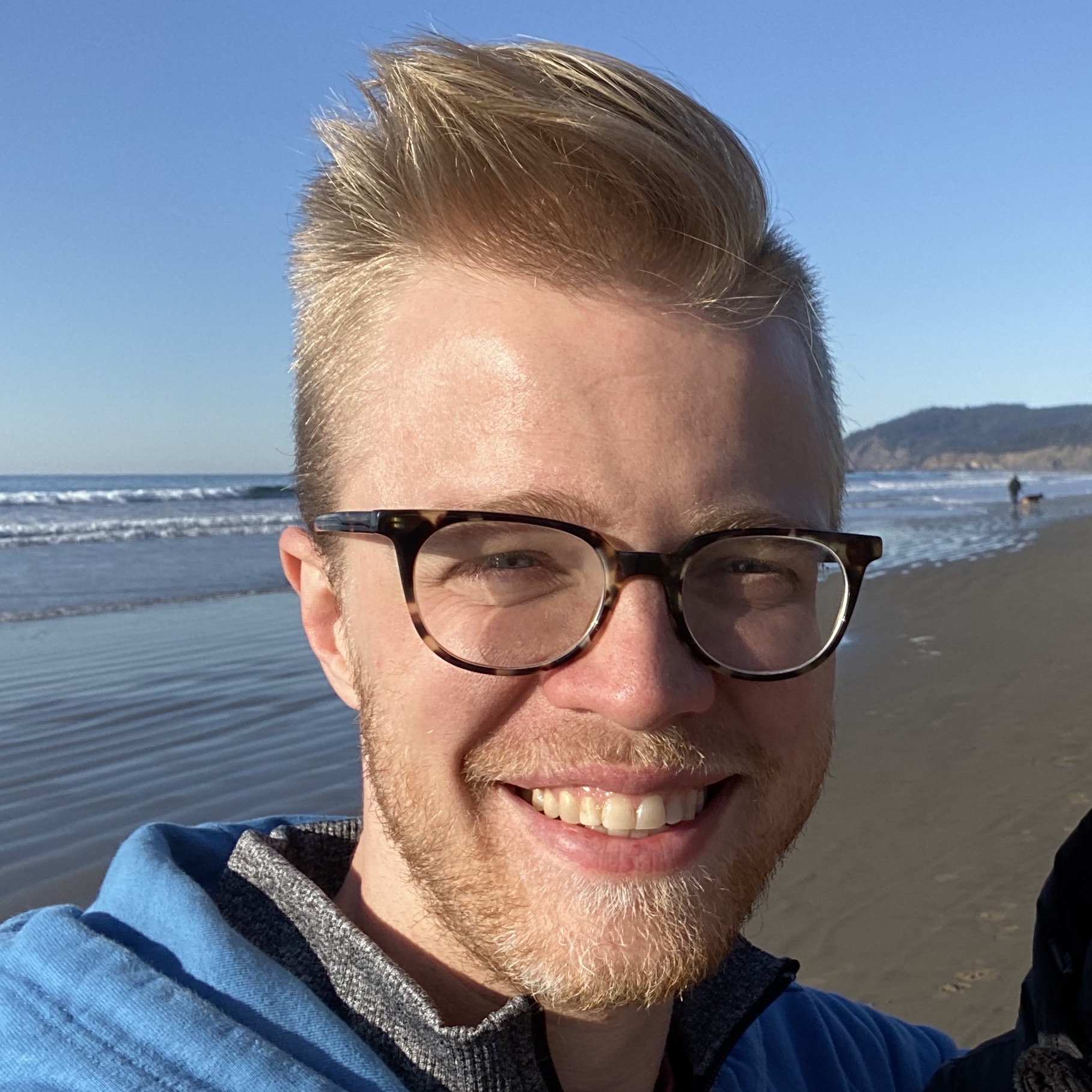
About Landon Schropp
Landon is a developer, designer and entrepreneur based in Kansas City. He's the author of the Unraveling Flexbox. He's passionate about building simple apps people love to use.
I love it! I worked at a place that retailed tons of lighting, so sometimes I would ask the product developers to name the colors for me! Grellow was one of the best names they gave me!
I just started using SASS and I was wondering how people deal with lots of colors, and this is by far the best solution as well as the simplest.
Grellow made me laugh. I’m glad you liked my solution!
Seems like you followed down the same path I ended up, finding that using named variables gets messy. Especially if you’re using a proper autocomplete.
Working in a project and typing
$somecolor $othercolor $thirdcolor
trying to get the autocomplete to help me out with the name I’m looking for.As a good convention, try grouping your scss variables. For example…
You can then use this to autocomplete and find your color, by typing
$color-
and having all your color variables pop up. Then, you can extend this for other variable groupings, such as:This has been a huge QoL improvement in my own workflow. Hope it helps someone else.
This is a great tip. Thanks for sharing!
Woops, forgot a point. To the idea of naming for purpose, versus color. You should do both.
For color:
Then for purpose:
I came here to post this but you beat me to it!
After 20-30 projects I have found this the easiest maintainable way of working with colours.
Sacha Greif described this approach 2 years ago: http://sachagreif.com/sass-color-variables/
This would have been where I read it first, as a follow up, I suggest reading this: http://engineering.appfolio.com/2012/11/16/css-architecture/
Paul, you nailed it. Use both! I was going to post the same exact 2 points as you, and as Rory said, you beat me to it!
But, I do have a 3rd point to add!
When your project involves multiple shades of a color, as most projects do, I think the best pattern is
$color-(color name)-(luminance)
. For example,$color-blue-42: hsl(201, 100%, 42%);
.This gives plenty of room for growth (even though hopefully there will be none), and is way better than “darkerest-medium-lightish-blue”.
This is the way that’s best.
1. Define your palette.
2. Apply the palette to semantic variables.
3. Apply your variables to the places they are used.
You can also save yourself friction by naming your classes / id’s carefully, and then you can just use them to name your variables.
ie:
$id-selector-path-property: $my-gorgeous-colour;
and so on…
IMO solution proposed by jason is by far the best one.
Using css variables aliases coupled with semantic context allow setting up color themes easily.
Using directly color variables in your css definitions is a real problem when one day you don’t want anymore a black background for your header and want to use a blue one, but your variable is named $black.
Landon, thanks for the article, and for more that I like your proposal, I’m not sure this would work for me, because if I name the variable after color name, but in the future I need to change that color to a complete difference, I would have a color name variable with a different color… for instance… lets say I have a border that has the color
#b0eb00
and per your approach, I would give the variable name “lime”… But later on, my customer decide that the border color should be red… what do I do?? change the color on my variable or create another variable called “border-color” and set red and go back to the property and set the new variable?? Well… I`m back to square one…I would use separate variables in those cases:
Now you can easily change the border color to red in a single place.
This is a great point, and one I’ll address in an edit shortly. The short answer is if there’s one thing in your project you want to change, like a border color, then it makes sense to change it where the border color is styled. If you want to change a color across your entire project, say from $lime to $red, then you can change your color variable’s name and value, and then do a quick find and replace in your project to get the rest of them.
Good one ..
This is amazing how CSS is being more advanced and gives the UX devs more and more power to keep it more maintainable …
Love it ..
I find lists quick and handy for grayscales or other monochromatic shades. I use the nth selector directly but you can also use semantic variables.
https://gist.github.com/GeorgeHastings/a31752f8fee67894edce#file-gistfile1-txt
Sorry, been there, done that – doesn’t help either. You end up with a bunch of names that don’t mean anything. The name that colour names are a bit whacky, and even if they weren’t, are you really going to remember the difference between $heliotrope, $tundora and $silver-chalice? In the end, they might as well be lorem ipsum strings.
I think the problem simply cannot be resolved. There is simply no easy way for the human brain to catalog a long list of colours.
Still, I’ll keep on trying. next time I am going to try names of cartoons or comic characters with some sort of a colour association, for example $hulk, $kermit, $raphael, $green-lantern for greens etc, maybe they will be more memorable. They can’t be worse than $mine-shaft.
I was going to make the same point here, while having real color names may help some people who are super-duper color fanatics – the rest of use who have trouble telling say magenta from fuchsia or teal and turquoise – it won’t be that helpful.
I think any way you go about it using your own names for colors, using real color names, associating it with characters, plants, or whatever it may work fine if you are the only developer, but when working with a team it could get very tricky. For example, I name a variable $raphael thinking of the 80s/90s TMNT series where the turtles were a dark shade of green. That’s my association – but new intern Joey fresh outta high school isn’t a 90’s baby and he associated $raphael’s color of a more limey green from the current mutation of the TMNT series. And then he may try to create a new variable for a color that already exists.
I don’t have a solution really. I tend to work as Paul Morrison explained above.
For those that use Sublime Text, this package shows you what colours your variables/hex codes are: https://github.com/Monnoroch/ColorHighlighter
It’s pretty handy!
Exactly! And guess what, I think of red when I hear Raphael, because he’s the red ninja turtle.
This is really great, thanks for sharing this valuable tips.
Thanks for sharing your ideas.
I usually group my colors by components. We rely heavily on reusable controls that’s why we relate our variable scheme to our JavaScript namespacing eg rk_header_buttons_color.
This is bulky at first but helps you later in the project when working in (distributed) teams. Refactoring can be done later. Less code doesn’t always mean better maintainability.
In my experience this is the best approach. Others like global color schemes didn’t help us because sometimes you have the same color in different contexts and if you don’t split by context color debugging gets hard in large web apps and theming for customers. On the other hand you always need to copy the whole color scheme if you want to reuse one component.
Regards,
René
First :
If you decide to use a different color than
#b0eb00
, you have to change it in multiple places. This quickly becomes aggravating in a large project.Then :
A few people have asked how to change a color using this methodology. For example, how would you swap
$crimson
for$royal-blue
? Because your colors are uniquely named, it’s easy to do a quick find and replace to swap them out. Every modern code editor supports this feature, so change away!Conclusion : doing a find and replace on a color variable is easy, but it becomes aggravating on a color hex code :)
Interesting approach but I guess to name the colors isn’t a best practice. How many colors do you need for a project? Most of online color palette generators use up to 5 patterns for a theme. Ok, rather then 5 let’s say you have to use 10. Still isn’t enough? Well, in this case your approach about use lighten(color) and/or darken(color) would be great indeed. Thanks for sharing!
Hex codes are so boring. Start using HSL and you will actually understand your colours!
Agreed … HSL is 10x more “human decodable” than hex!
Here’s a cheat sheet on visualizing a HSL color:
Hue: increments of 60° give you Red (0), Yellow (60), Green (120), Cyan (180), Blue (240), Violet (300).
Saturation: Anything lower than 100% turns the color gray.
Luminance: 0% is black, 50% is a solid color, and 100% is white.
Yeah, check my post out. It’s about this topic:
http://lovefrontend.com/2014/06/dynamic-colour-palettes-defined-sass/
I think in the second code example, you meant to swap out the hex values to use
the variable.
Oops, you’re right.
Sooo, can you change it? That was the first thing I noticed about the article. Thanks for posting BTW. And the comments here have been as valuable as the article. So thanks to everyone else too. :)
I’ve just noticed this too so maybe it needs to be changed to avoid confusion. Really enjoyed the article though so thanks!
Great post! I also found that my new favorite color picker for OS X, called Sip, also creates memorable names for colors.
The application “Sip” (mac only) also includes those color-namings, so it’s easy when you “sip” a color from some image you directly have an appropriate color-name. It’s funny how recognisable “$darkest-grey” was for me! :) Great post!
Hurray me :-) I’ve been using this method for over a year now.
It evolved naturally and made things easier for me, but I just couldn’t explain it like it as well as in this post.
I think using color names as variables defeats the purpose of having variables in the place.
Variables are used because, well, they can have variable values. In practice, this means that you can just change e.g
$bg_color
from#fff
to#fafafa
.If you had to attach a specific name to a specific color code, then you have practically made the variable to be invariable. Also
$mine-shaft
is only as memorable as#292929
already is. If you ever had to go through your CSS files and do a “search and replace” to change the variable names then you’re simply doing it wrong.The only very little advantage I can see with proper color-naming is making them easier to recognize, but only for certain sensible color names.
Thank to sharing interesting articles and services
http://twodots.in/
I really like the article it brings us some very good points. but…
Why not use primary, secondary, tertiary as your variable names. This would allow you to have a structure to the variable names, keep the colors abstract and easily change the color scheme. While they do not allude to the color they represent making them somewhat difficult to use, it allows you to quickly change every where the primary color was used by changing one value. Allowing you to change from a blue to a red with out having to update the variable name. Just a thought.
How about this:
$lime: #b0eb00;
$bright-red: #b30015;
$dark-blue: #2a00b3;
$brand-primary: $lime;
$brand-secondary: $bright-red;
$brand-tertiary: $dark-blue;
You can always change things in only 1 place, in this case…
Personally I’m not a big fan of naming variables after colors like “heliotrope” or “lime”. First, I would never remember heliotrope, especially if I revisited a project after 6 months or so. And secondly, it’s descriptive, but it’s not flexible. Let’s say a company rebrands and wants to change the colors on their site and suddenly your
$lime
needs to be a red color… you would have to either change each instance of$lime
to$strawberry
(which defeats the purpose of using a variable) or just change the hex and be left with a variable name that no-longer makes sense.I prefer to name things like:
$brand
$brand_tint
(or$brand_light
)$brand_shade
(or$brand_dark
)$accent
This way if someone changes their brand colors from red and green to blue and orange, you can update the hex values in only 1 place and your variable names still make sense to anyone else who might come on the project.
Some things are just always going to be tricky like grays because there are so many shades and it’s all relative to the project. Although I’m not a fan of this naming convention, I personally I have better luck with names like $gray_darkest, $gray and $gray_lightest over names like “$mine-shaft”, “$steel-pipe”, or “$titanium” because everyone has a different color pop in their head when they see those names and it’s impossible to set any relation between them. With words like ‘darkest’ and ‘lightest’ at least we know where to start in relation to other grays.
Love this. I’ve been using the first method of “what does this color actually do” and it’s been pretty beneficial, especially in a team setting. Nice writeup!
I like to use a colour picker when designing in the browser, like ColorZilla or 0to255.com, but most of these return a hex, rgb or hsl colour.
Here is a handy little colour picker for selecting Sass code for a range of tones in a palette:
http://www.regbirch.com/blog/colour-picker-for-sass
Why not combine the best of both worlds and use something like…
That way you can change it only in one place still and have sensible naming conventions.
OSX users you can turn that
Name that color
util into a Dashboard Widget by from the Safari context menu, then its available all the time – neat huh?I agree with the other comments about creating a colour palette using the colour names and then creating other domain specific variables that reference the colour palette for specific uses across my styles
Just use an app called Sip from the App Store ;) does it for you automatically…and it works as an eye drop tool for your desktop!
I’m failing to see the point in naming colour variables after their colour. That hasn’t really added any benefit of using a variable, if you’re simply using
$mine-shaft
, for example, in your Sass code. You may as well just use #363636 for all the benefit of using a variable named after its colour is giving you.This article is completely missing the point. The use of variables (ie. something that’s not fixed in value) is to be able to change them in time so imagine you’ve filled your sass files with a variable named $lime-green and now you want to make that orange.
There’s no benefit in naming colors like that, if you really want to remember their name, you can add a comment for each variable. But that’s also stupid, your editor of choice should be able to show you the color inline (if you really care about that, I don’t).
So a better naming convention (in my book) is to either scope your variables to your modules or have them global or both.
Scoped: — (“button-alert-text-color”)
Global: primary,secondary,etc- or -1,2,3 (“primary-text-color” or “text-color-1”)
Both: -primary,secondary,etc- (“button-primary-text-color”)
— Cezar @ Mix & Go
http://goo.gl/vyc33c
Agreed.
If you’re going to use a search and replace to change $crimson for $royal-blue then you might as well use search and replace to change #990000 to #4169e1.
I use a 2 tire system to manage colors in sass.
First i define the colors like so…
1.Colors are arranged in groups of :
grey,
red,
blue,
green,
yellow,
purple,
pink,
base colors,
Social icon colors by website name.
2.Each group of color has 6 shades. The shades are arranged from light to dark(Only thing you need to remember is that the colors are arranged from light to dark) “color” name being the lightest and “color6” being the darkest.
3.Usage:
If i want a light grey i will just call the variable “$grey”
If i want a darker shade of red i will increase the numbers $grey3, $grey4 and so on to get the darker shades.
$grey: #d1d1d1;
$grey2: #878790;
$grey3: #757575;
$grey4: #616161;
$grey5: #424242;
$grey6: #161416;
4: In the 2nd tire i will use the descriptive variable names like:
$color-text : $grey6;
$color-light-text : $grey2;
The only thing you need to remember is that the colors are arranged from light to dark no need to remember any fancy or funny names as you tend to confuse or forget the fancy color names as your styles build up.
I am currently working on a big project and I have been through the nightmare of organizing sass variables. I have ended up with the following:
I have a variable folder which contains separate SASS files each with a single purpose (one for colours, one for font-sizes and so on…)
Naming the variables is challenging especially when working in a team of many developer. I shall adopt this solution, I really like it.
+1 for this structure, it’s the same I use
I’m totaly desagree with this practice.
Never call our variables with the color name and something like that, it’s not semantic!
This naming,
fails. I say fails. why?
you have the
background-color : $lime;
and then change the background color,
$lime
to some another one, say#232323
,then
$lime
will be#232323
thats a gray, but not lime,result:: EPIC FAIL
+1, he does not understand how the variables work
Hi David,
I use that convention for a while, knowing it has some disadvantages, but I consider this as a good compromise.
Anyway I use Atom on my daily basis, and recently I wrote a small Atom package which names the colors itself :-) Maybe you’d like to check this out:
https://github.com/kkoscielniak/atom-name-that-color
and so on, get a new color just add it to the list aka the “paint cupboard”. An artist will just keep adding tubes of paint to the cupboard.
Get your painters pallet and define your colors…
and so on.
Seriously there’s no overall good solution so don’t worry about it. If we’re spending so much time debating how to organize colors then wtf are we doing? I got far bigger things to be concerned about – and no this doesn’t make me a bad dev.
As long as no colors are defined directly in a partial I couldn’t give too hoots as I’ll figure it out and any intelligible person should be able to. As soon as you go down the path of naming colors your on a slippery slope in my view. Just install a color highlighter plugin and your done; KISS
English grammar to the rescue: superlatives
This approach is nicer if you want to support theming with custom skins:
https://alexpate.uk/journal/naming-sass-color-variables/
The idea:
– create inner color variables
– create abstract palette variables (and your color palette should really be small, just under 10 colors) combining the color variables. Use the abstract variables for all UI element styles
Then, if a customer wants to change a skin, you can just adjust the global abstract variables to new palette colors.
I think you’re missing the whole point of variables here. If I would have to search a file’s for a color variable if I want to change the color, I might as well use plain CSS and HEX color codes and search for #00000 and replace it with #FFFFFF instead of searching for $black and change it to $white.
If the designer has some UI knowledge, they would know that there is a color hierarchy in every interface design. I’d rather go with $cta-primary-color for the CTAs (Call To Action) rather than $somefancyword-green, that way, if the UI/UX architect decides to change the CTAs’ color, I would not have to do a “find and replace” action inside the whole SASS file(s).
Thank for you post!
This solution resolves half my concern. I want to ask about when we use color in a big framework, (1) we will define class for each color variable? Ex:
That is my concern.
If working with large scale applications continuously adding features, updating your layout and throwing 100’s of themes into the mix with junior, new starts, non-frontend devs using the current
scss and you have:
Imagine you have a product with 1000 different theme files with multiple colors changing over time with new features and accessibility colors thrown into the mix, as well as your own possible evolving layout, trying to figure out if this shade of gray is changing this shade of red and so on and so on.
Yes the same color could be used for where red is on the header background, links font and button background, so in your theme its referenced to where red, blue, purple & black could be the base header, buttons and links because their branding only has 2 colors, gray and green and thats worked for them forever.
A better way IMO with a view to readability and maintainability.
Use them as how they are used with a bem style naming method if you so happen to be following bem in your classes.
Some will argue they dont want to waste time writing a longer descriptive color name.
But would you rather use those seconds now than hours later trying to work out blue from red in multiple different places over multiple files in different combinations, when you can change those colors in one place to affect changes over multiple files and lines of code ?
Its important to think about who is going to use, maintain your code when you’re on holiday :)
For those hating on assigning colors to variables, consider that a color can be defined by its name, hex value, rgb (3 or 6 digits), hsl, etc… The benefit of assigning each color to a variable is (especially when there’s multiple devs) that in order to replace all occurrences of that same color you don’t have to search for
The variable does make sense because not everyone uses hex colors, and some of them can be 3 or 6 digits even when they do, so there is some variability per color that the variable addresses well.
Then taking the next step would be to assign those colors to variables that are more re-usable, like
$border-color
,$main-bg
,$title-color
, etc…This is no different than just not using variables at all. What’s the point? The whole point of using variables is because you can change the variables value later on (hence the name variable). If your just doing a find and replace to change the variable name everywhere in your CSS you might as well just do a find and replace to change the hex code…