Check All/None Checkboxes Using MooTools
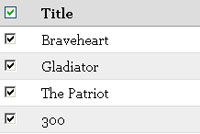
There's nothing worse than having to click every checkbox in a list. Why not allow users to click one item and every checkbox becomes checked? Here's how to do just that with MooTools 1.2.
The XHTML
<table> <tr> <th width="30"><img src="checkboxes/uncheck.jpg" id="ucuc" /></th><th>Title</th> </tr> <tr> <td><input type="checkbox" name="approve[]" class="check-me" value="Braveheart" /></td> <td>Braveheart</td> </tr> <tr> <td><input type="checkbox" name="approve[]" class="check-me" value="Gladiator" /></td> <td>Gladiator</td> </tr> <tr> <td><input type="checkbox" name="approve[]" class="check-me" value="The Patriot" /></td> <td>The Patriot</td> </tr> <tr> <td><input type="checkbox" name="approve[]" class="check-me" value="300" /></td> <td>300</td> </tr> </table>
Note the image with the ucuc ID -- that image will be the checkbox trigger.
The CSS
#ucuc { cursor:pointer; }
Give the image the pointer cursor for enhanced usability.
The MooTools 1.2 JavaScript
window.addEvent('domready', function() { var ucuc = $('ucuc'); ucuc.addEvent('click', function() { if(ucuc.get('rel') == 'yes') { do_check = false; ucuc.set('src','checkboxes/uncheck.jpg').set('rel','no'); } else { do_check = true; ucuc.set('src','checkboxes/check.jpg').set('rel','yes'); } $$('.check-me').each(function(el) { el.checked = do_check; }); }); });
Extremely simple: read in whether to check or uncheck the boxes, take the appropriate action, and swap out the images.
you can do that more simple
could be
Since all Element methods can be applied over instances of Elements class ($$ returns it). Those methods apply loops internally (see Elements.multi)
:)
Thanks for the tips guys!
it would be interesant do this example with pagination, keeping the marked selection during page navigation. It could be with cookies…
Finally someone who can make check all work with a name=”xyz[]”. Only issue is I tried to input your code and got error: “object doesn’t support this property or method” and “style is null or not an object”.
What did I miss here?
Very nice work!
One question: what would you do if you have multiple tables, each inside its own form, and you want to check/uncheck only the checkboxes inside each particular table?
Just incase anybody wants to do it without putting class names on all your checkboxes. Instead, stick an id on a html element that surrounds them all.
This is probably the answer to “Farao’s” question.
Set var yourContainer to be the id of the surrounding html element.
Example, if your container had an id of “monkey” then var yourContainer = “#monkey”. This way it only targets checkboxes in that area. It’s a far better global solution than putting a class on each checkbox.
This method takes the first checkbox found and uses it as a reference for the others to follow, to avoid mismatch checking.
Simplez.
@Adam:
My code above is flawed,ignore it, or David, please delete it :)
Here’s the code that works (shorter too!)
@param container => The mootools representation of the element id that all the checkboxes are in. Eg “#myElementId”
@param obj => Set to ‘this’. That’s because the onclick event should be on the checkbox that is the SelectAll/None, so ‘this’ refers to the current state of this checkbox.
You should write $$(“input[type$=checkbox]”)… tra-la-la
Good suggestion, but that may get elements I don’t want, so maybe:
$$(“input[type$=checkbox].check-me“)
This post is quite old, maybe I should update it.