Add Controls to the PHP Calendar
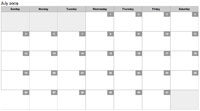
I showed you how to create a PHP calendar last week. The post was very popular so I wanted to follow it up with another post about how you can add controls to the calendar. After all, you don't want your users to need to wait until the next month to see events outside of the current month, right? Now we'll add "Next Month", "Previous Month", and month/year dropdown controls to the calendar.
The PHP => HTML
/* date settings */ $month = (int) ($_GET['month'] ? $_GET['month'] : date('m')); $year = (int) ($_GET['year'] ? $_GET['year'] : date('Y')); /* select month control */ $select_month_control = '<select name="month" id="month">'; for($x = 1; $x <= 12; $x++) { $select_month_control.= '<option value="'.$x.'"'.($x != $month ? '' : ' selected="selected"').'>'.date('F',mktime(0,0,0,$x,1,$year)).'</option>'; } $select_month_control.= '</select>'; /* select year control */ $year_range = 7; $select_year_control = '<select name="year" id="year">'; for($x = ($year-floor($year_range/2)); $x <= ($year+floor($year_range/2)); $x++) { $select_year_control.= '<option value="'.$x.'"'.($x != $year ? '' : ' selected="selected"').'>'.$x.'</option>'; } $select_year_control.= '</select>'; /* "next month" control */ $next_month_link = '<a href="?month='.($month != 12 ? $month + 1 : 1).'&year='.($month != 12 ? $year : $year + 1).'" class="control">Next Month >></a>'; /* "previous month" control */ $previous_month_link = '<a href="?month='.($month != 1 ? $month - 1 : 12).'&year='.($month != 1 ? $year : $year - 1).'" class="control"><< Previous Month</a>'; /* bringing the controls together */ $controls = '<form method="get">'.$select_month_control.$select_year_control.' <input type="submit" name="submit" value="Go" /> '.$previous_month_link.' '.$next_month_link.' </form>'; echo $controls;
I wont explain the code because it's boring and simple but you may be wondering why I didn't include the controls within the PHP function. Since you may or may not want the controls and will want to style them differently, placing the controls within the PHP function would be a bad idea.
Can you think of any other controls to add to the calendar? How about an AJAX method? Share them!
http://davidwalsh.name/dw-content/php-calendar-controls.php?month=2147483648&year=2147483647
That is the maximum date that can be generated from your scripts. Maybe it will show an epoch-like bug in 2147483647-2009=2.147.481.638 years from now.. xD
You should have it highlight “today” :)
@Alex: I could set up a min/max for year values, but I’ll let people like you do your worst :)
@Ahmed: Good idea. Once I get some more ideas, I’ll revise the post with all of them included.
You are about 12 hours too late! XD
Maybe we should all hook up on some big open source calendar class project, or something…
@Chris the Developer: Sorry :D
How about custom time zones too?
Very nice Calendar I’m just gonna repeat the same question as Ahmed did, highlight Todays date.
Zed
How about ading events to the calander?
Nice script. Will be using and giving credit for this one…
Many thanks
@ all the ones that requested Hilighting todays sate I did it by changing the following code
TO
And adding a new item in the CSS for DIV.day-today
Hope that helps, its probably not the best way of doing it, but it seems to work!
Jimmy
Nice script, simple to the point, great addition to the first part.
The only things I could think to add would be maybe some sweet functionality to throw a day into a modal to display all of the events on the day, or to pass data to ajax a model for a more in-depth description of the event or data set.
also on Jimmy’s code block, unless I am missing something there seems to be something missing from his second code block…
but the basics is as follows… compare todays date to the one in cycle, if they match modify the class name.
//where you would add a class to the css to modify the color of the cell to what you want…
heeii.. how about adding event to calendar?
Hi guys – great examples just wondered if you provided downloadable archives of the examples?
thanks again :-)
Another way to highlight the current date, not the smallest amount of code possible but I just made it nice & simple to read for less experienced php guys & gals :-)
Feel free to give me constructive criticism i’m still learning myself :-)
Hi Gav – a few people have posted examples of how to highlight current day – I can get none of the examples to work except yours – good job
Just noticed it does something weird in Internet Explorer 8 (haven’t tried IE6 or 7) works fine in Chrome/Firefox though – On my website it displays the calendar fine, however on my local LAMP development machine it doesn’t – it is the exact same code so cannot see why it would be doing this any ideas? (See the screenshow below)
http://www.gavk.co.uk/calendar/calendar.png
Note – if I refresh the page it then loads fine, however when I click ‘Previous’ or ‘Next’ month it goes back to the weird unformatted style.
Am I doing something really dumb here any responses would be appreciated (but nothin mean lol) ;-)
Thanks
Sorry guys if it feels like I am spamming this!!
Ignore the last question – I must have been having a ‘blonde’ moment – the CSS should have been above the table, I must need more sleep :-)
Thanks again David – this is a really impressive blog! Keep it up! :-D
Hi Guys – Last comment I promise!
Nothing special but some have mentioned having ‘events’ integrated into the calendar, i’ve done some ‘basic’ that grabs events from a mysql DB using the adodb library (note the database stuff is only on my local testing machine not the site URL below as of yet).
http://gavk.co.uk/calendar/calendar-v1.php
I’ve used the jQuery Tools library to do the overlay effect – if this of any use to anyone I can provide the PHP files if anyone wishes so you can play around with it further.
It’s good to give something back for once :-)
Awesome blog David keep it going!
Hi guys,
I’ve been playing around with this example recently and just wondered the best approach for events that span multiple days?
I got a decent demo working that pulls date via the DB for single dates and displays them but cannot work out the best approach for an event that will for example start on 5th Dec & end on the 8th Dec – ideally i’d want this to show this event appear for times, the 5th, 6th, 7th & 8th days of the calendar.
Anyone have any suggestions?
@Gav :
hey bro it’s really helpfull for us if you can send us the PHP codes. we are stuk with an assignment. :((
Thanks
@Gav:
Hi Pankaja – bit busy with a project myself too at the minute – I will at some put these files up with some commenting – as my code is looking very bloated now – while it works it is confusing even when I look at it lol :-)
At some point i’ll upload these somewhere then you can feel free to modify & hopefully improve upon my attempt as i’m no expert (unlike Mr D.Walsh!) :-)
David:
Is there a place where the code for the first two parts, the calender and the controls, are linked together. If not can you show me how to integrate them.
Thanks
WBR
This is what I am looking for, but like WIlliam I am not sure where to put the code for the controls.. Where would it go?
I just cant get the controls to work. Not sure where the colde goes.
@William, Gary..
Just put the controls at the top of the page within the tags – give me a shout if your unsure…
Oooh! I got it working!
Thanks!
William… Where did you put the code?
In my code it ended up after the random number function.
I am so new to this. However I don’t even have a function random_number.
Gary:
I would send you the code I have, but I don’t want to publish my email. One alternative for you is to view the source of the demo, and edit it down to see the relevant parts.
That’s okay. I am sure I will figure it out. @William Rouse:
@William Rouse:
William, if you are still willing to share the code here is my email. gary@ssdgn.com thanks in advnace for the help.
Thank you William. Now I have to figure out how to connect it to the database in order to add the events. Way over my head, but I am learning.
Hey Gav, William or Gary can you send me the code? i am having the same problem and i also want to be able to show the events on the calendar itself.
Thanks for your help. harolfuentes@yahoo.com
Hi Harol – I will try and get this for you on the weekend and illustrate how I have done the events – the way that I have done it is not the best method but does the job. Will sort this out for you in a couple of days just mega-busy at the minute..
@Gav:
Can you take a look at the highlight for the current day also. I see it in the CSS but not in the code.
Thanks
WBR
@William
No worries highlighting the current day is fairly simple – will point that out for u as well.
@Gav and William,
Look forward to the updates, thanks for alll your help.
Hi guys – not sure how exactly I missed this before but there is another article by David Walsh with doing the events here.. well worth a read, looks easier than my method as I required events spanning multiple days, months etc..
http://davidwalsh.name/php-event-calendar
As for setting the current day try this..
/* $today variable will be set to ‘today’ or EMPTY depending on the date matching the current date */
$today = ($list_day == date(‘j’) && $month == date(‘m’) && $year == date(‘Y’)) ? ‘ today’ : ”;
Then when you loop through each day in the calendar when we hit the current day it will print the string ‘today’ – you could put this into something like event stuff…
Long day so not the best explanation I know! Let me know if that doesn’t make sense i’ll give you another heads-up with it.
Gav
then i’d just create a css class called ‘today’ and maybe change colour, font-weight etc…
@Gav: Well after 3 readings it doesn’t make sense and the line of code seems to have syntax errors, so help the lame, and show where in the code you placed this.
WBR
@William: I will try and simplify what I have done as it uses something called ‘adodb’ for getting stuff via a database which will probably confuse the hell out of you :-)
Yeah, you are right, if this is from the old ActiveX Data Object, I have not seen that since I worked on C++ with a Borland compiler. Yikes!
It isn’t that bad lol – I’ve just used this for the database stuff
http://phplens.com/adodb/reference.varibles.adodb_fetch_mode.html & used object orientated programming to ‘seperate’ everything into objects, really useful once you get your head around OOP.
Will simplify for you soon :)
echo $controls
to see the controls….
sorry
echo $controls;
There’s a slight issue with the calendar function when the last day of the month is the last day of the week (e.g. July 2010), where an extra row of days is generated.
The fix is shown in the following code snippet:
@Cindy:
I found the error you described about July 2010 in my version of the code also.
In trying to update my code, I found syntax errors in your example:
$calendar.= ‘’;
This produces a syntax error for me.
Would you be able to post your entire file somewhere for inspection?
Thanks!
WBR
Hello and thank you for some very good tutorials.
I have only one question, i’m a bit new on programming in php, and i wondering how to get the controls to act with the calendag?
Thanks in advance.
I have the calendar working without the database. I am trying to add the highlight today feature mentioned in some of the comments. However, I am an intermediate php programmer and must not understand some of the shorthands used in the code snippets. Does someone have the full calendar code with highlight today implemented, or at least the full code for the affected section?
Is there any way you could send me the code for this calendar. I am trying to adapt it to a site I am working and I am experiencing a few minor glitches. ie: When I add the controls the month that is printed out in the brackets does not correspond to the selected month.
Thanks for your help.
Sorry for the second post, but I ment the header tags when I said brackets.
Is there any way you could send me the code for this calendar. I am trying to adapt it to a site I am working and I am experiencing a few minor glitches. ie: When I add the controls the month that is printed out in the
brackets does not correspond to the selected month.
Thanks for your help.
Thanks for the script.
can anyone help me with high lighting the current day and adding events to the calendar?
Thanks
I know no ones posted in a while but I do not understand where to place the controls in the calendar… if someone would like to send me their full code, they can email me @ eddy_edmondson_@hotmail.com
I’ve placed the calender controls in a private function within a calendar class that I built previously, and included a reference to this function where I actually build the calendar. The controls show up fine, however the calendar itself wont actually change when the controls are used, is there something that I’m doing wrong?
Any help would be appreciated
Hi there I love your calendar script, i copied all out with the additional controls , but I would like to know how to get the controls to operate the calendar??
Hello and thanks for this great calendar…
I have it working fine but only once…
I’ll try to explain what happens :
First time the calendar load fine with events of the month, but if I click on next or previous month, the calendar is redrew but the events are not displayed, the navigation itself works (next mont, previous month are changing the month, but it seems the function does not recalculate the events when changing month…
Any idea ?
Thanks in advance
Controls only change value of the select menu, dont recalculate calendar.
I added the code of controls in a separate function but I get the following error:
Undefined index month,, Undefined index year..
Could you please tell me how to fix this ? this is urgent.
Thanks
where to put controls and how to make work them? Please help me to solve this.
How to fill the empty boxes with the last days of the last month, and the first dates of the next months? :)
How do you prevent the url from changing? Like in the demo?
I think this line :
must be :
we should use
!empty
or!isset
there.i used the following code:
i want to show event and current dat . please help me
how do you solve the problem of Notice: Undefined index: month in C:\wamp\www\david walsh calendar\draw_calendar.php on line 70 and Notice: Undefined index: year in C:\wamp\www\david walsh calendar\draw_calendar.php on line 71 when the calendar is first loaded? they go away when you click next or previous month. i tried doing something like:
but that didn’t work. is there anyway to make the calendar load on the current month?
got it to start on current month by using this:
Hi Guys,
I have done this Calendar and it’s almost working fine but I have this one issue that has cost me days and nights of my life.
When I get to the year 1901 it does only start to show me the first month in the select field “january” and it also does not arrange the days anymore…. anyone out there with help…. ??
Hi, not sure if anyone is still out there for this… but I need some help with getting the events displayed on the calendar to span across multiple days.
After I found this calendar that David Walsh published i implemented it immediately and it’s working beautifully, but i just need this functionality for what I’m using it for. Please help
Hi,
It works fine with months but i have a 404 error when i change Years.
My page is named my_script.php
I assume there is a relation with htmlentities($_SERVER[‘PHP_SELF’]
Thanks a lot