Face Detection with jQuery
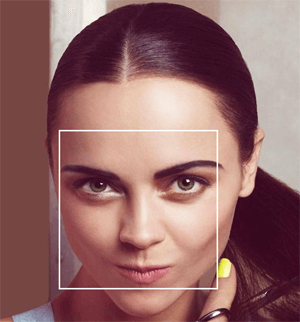
I've always been intrigued by recognition software because I cannot imagine the logic that goes into all of the algorithms. Whether it's voice, face, or other types of detection, people look and sound so different, pictures are shot differently, and from different angles, I cannot fathom how it's all done. Since I already covered booby nudity detection with JavaScript, I thought it would be worth some time to explore face detection. Facebook uses it, so maybe it has application in your websites.
One face detection library I found is Face Detection by Jay Salvat and Liu Liu. This is a standard jQuery plugin that receives an image and returns an array of coordinates of faces found within the image. Let's have a look at how to use it!
jQuery.faceDetection
Four JS files are required for this jQuery plugin:
<script src="jquery-1.4.3.min.js"></script> <!-- mas js --> <script src="facedetection/ccv.js"></script> <script src="facedetection/face.js"></script> <script src="jquery.facedetection.js"></script>
The faceDetection plugin wraps functionality within the first two JavaScript files, and returns an array of objects which represent the coordinates of the faces within the photo (if any are found). An example would be:
var coords = jQuery("#myImage").faceDetection(); /* Returns: { x: 525 y: 435, width: 144, height: 144, positionX: 532.6353328125226, positionY: 443.240976080536, offsetX: 532.6353328125226, offsetY: 443.240976080536, confidence: 12.93120119, neighbour: undefined, } */
You may also add event callbacks to every call:
var coords = jQuery("#myImage").faceDetection({ complete: function(image, coords) { // Do something }, error: function() { console.warn("Could not process image"); } });
It's up to you what you'd like to do when the faces have been found. You could add a square around the person's face:
jQuery("img").each(function() { var img = this; // Get faces cooridnates var coordinates = jQuery(img).faceDetection(); // Make boxes if faces are found if(coordinates.length) { coordinates.forEach(function(coord) { jQuery("", { css: { position: "absolute", left: coord.positionX + 5 + "px", top: coord.positionY + 5 + "px", width: coord.width + "px", height: coord.height + "px", border: "3px solid white" } }).appendTo(img.parentNode); }); } });There's not much more to it than that!
I tried to vary the photos I used faceDetection on and as I expected, the results are the not perfect. They are, however, quite good; no software will be perfect for all cases. The software also does not do facial comparison, so you would need to provide suggestions as to the face's identity via another method. For what this plugin is meant to do, however, it does pretty well. I encourage you to give this a try!
Recent Features
CSS vs. JS Animation: Which is Faster?
How is it possible that JavaScript-based animation has secretly always been as fast — or faster — than CSS transitions? And, how is it possible that Adobe and Google consistently release media-rich mobile sites that rival the performance of native apps? This article serves as a point-by-point...
Regular Expressions for the Rest of Us
Sooner or later you'll run across a regular expression. With their cryptic syntax, confusing documentation and massive learning curve, most developers settle for copying and pasting them from StackOverflow and hoping they work. But what if you could decode regular expressions and harness their power? In...
Incredible Demos
Resize an Image Using Canvas, Drag and Drop and the File API
Recently I was asked to create a user interface that allows someone to upload an image to a server (among other things) so that it could be used in the various web sites my company provides to its clients. Normally this would be an easy task—create a...
MooTools, mediaboxAdvanced, and Mexico
The lightbox is probably one of my favorite parts of the Web 2.0 revolution. No more having to open new windows (which can bog down your computer quite a bit) to see a larger image, video, etc. Instead, the item loads right into the...
Discussion
iPad almost burned when browsing the demo page.
Yeah, that will happen with client-side technology like this. In practice, this is better done on the server side, but it’s still fascinating to see on the client side.
The perfect companion article would be about webworkers :D
Yep latest FF got an slow/unresponsive script error while loading the demo. Interesting though!
Most facial recognition software is done with neural networks….the varied results are because of a limited data set used in training….in theory they can be nearly as good as people at recognizing faces (same technology for voice recognition, OCR (Object Character Recognition), etc.)..it’s essentially pattern matching. I haven’t seen any good neural networks for javascript though, so this is pretty interesting to me.
It would be great if the software could also recognize if people wear glasses, ear rings, wrinkles, et cetera. So that you would be able to apply filters on the image library and make selections.
Nice work but Slow Script Error on FF
This is done somewhat quite easy using matlab.
Too bad that my signal processing teacher was a bitch and I did not learn anything…
yeah and god accidentally forgot to give you brain
any thing on global browser detection am having battles with site displaying on other browsers as it did on my browser
This website http://blog.geotitles.com/2011/08/facebook-like-face-detect
had made a similar tutorial using the same jQuery pluign by Jay Salvat, but they have presented many examples on using the plugin.
May be it is useful for your visitors.
thank you for sharing, this is realy one of the complex jQuery plugins, but how the f**k they do it ?? have any one a document of the algorithm ?
Thank you.
Can you add sound output in your face detection program.. it say your name..
Hi i would like to recognize only one face from the group photo as well from the single image photo too. So what should i do in the code.
It would be great help if anyone say the solution.
Thanks in advance.
It works great but when there is no face to detect in a picture it does not trigger the error function, but only the “complete” function.
It does trigger the error function when the pic is below a certain size however.
I tried checking for undefined (and tried null too) in the array like so:
but no dice.
Any suggestions?
I am developing a chrome app so only testing there.
Nice Tutorial David.. :) you are really rocking.. please share more tutorials on jQuery..
Hi David,
The demo no longer works (in Chrome) because you can no longer embed javascript from raw.github.com .
Sairam
Hi David Walsh,
I am getting the error ‘ this image is invalid’. Can you hint how to solve this problem.
(when I tried it locally and on localhost)
Hi David,
This doesn’t seem to be working in Chrome at all and in FireFox is spits an error back at me. It did manage to pop out a white border box, however it wasn’t on the face.
Man.. hotlinking from github has been disabled. Now your demo doesnt work. Can you update your demo? Cheers!
hello, all.
How could I do this but without displaying the video on the screen ?
I want to detect if someone is in front of the camera and roughly where they are but I don’t need to video to be displayed. Any hints ?
Thanks
Hi David,
Do you have idea that how can we use this one to detect the face shape (like Oval, square, narrow etc…)
Please let me know if you know any API’s for it also…
Thanks
The example only highlights one soccer player, the photo with Brad/Angelina, and one photo in the black and white.
You could also forego any development and just leverage a pre-existing API service that provides this sort of functionality.
For instance the follow face detection API can be hit from javascript client side or server side.
Face Location
https://askmacgyver.com/explore/program/face-location/5w8J9u4z
Hi I need to detect faces while upload a file or dynamically but its not working for me so what to do?