Create an Animated Sliding Button Using MooTools
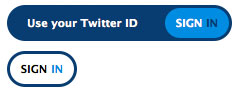
Buttons (or links) are usually the elements on our sites that we want to draw a lot of attention to. Unfortunately many times they end up looking the most boring. You don't have to let that happen though! I recently found a Tympanus post which provided a great method for making button have an unexpected pop. Here's a quick tutorial on how to duplicate that look using MooTools.
The HTML
The system works off of one DIV, two As and SPAN elements. Note the IDs and CSS classes for the CSS.
The CSS
.button_wrap{ position:relative; width:225px; height:36px; overflow:hidden; font-weight:bold; font-size:11px; margin:10px; } .button_aLeft{ width:70px; height:36px; -moz-border-radius:20px; -webkit-border-radius:20px; background-color:#093d6f; color:#fff; top:0px; right:0px; position:absolute; line-height:36px; text-align:left; } .button_aLeft span{ /* display:none; */ visibility:hidden; padding-left:20px; color:#fff; } .button_aRight{ width:70px; height:36px; -moz-border-radius:20px; -webkit-border-radius:20px; background-color:#093d6f; color:#fff; top:0px; left:0px; position:absolute; line-height:36px; text-align:right; } .button_aRight span{ /* display:none; */ visibility:hidden; padding-right:20px; color:#fff; } .button_bLeft{ width:64px; height:30px; background-color:#fff; -moz-border-radius:20px; -webkit-border-radius:20px; color:#000; position:absolute; top:3px; right:3px; text-transform:uppercase; line-height:30px; text-align:center; cursor:pointer; } .button_bLeft span{ color:#008ddd; } .button_bRight{ width:64px; height:30px; background-color:#fff; -moz-border-radius:20px; -webkit-border-radius:20px; color:#000; position:absolute; top:3px; left:3px; text-transform:uppercase; line-height:30px; text-align:center; cursor:pointer; } .button_bRight span{ color:#008ddd; } .button_c{ background-color:#008ddd; color:#fff; text-transform:uppercase; } .button_c span{ color:#093d6f; }
The CSS code above mirrors the original code with the exception that "display:none;" was changed to "visibility:hidden", per the differences in MooTools and jQuery fading methodology. Feel free to modify the basic color/formatting styles in any way you'd like.
The MooTools JavaScript
window.addEvent('domready',function() { $$('.slidebttn').each(function(btn) { var prev = btn.getPrevious('a').set('tween',{ duration: 200 }); var span = prev.getElement('span'); btn.addEvents({ mouseenter: function(e) { btn.addClass('button_c'); prev.tween('width',225); span.fade('in'); }, mouseleave:function(e) { btn.removeClass('button_c'); prev.tween('width',70); span.fade('out'); } }); }); });
The first step is grabbing all button containers and identifying the SPAN and A elements within them. Then we add mouseenter and mouseleave events to the container which do the animation of the primary A/SPAN elements.
Great work by Tympanus. The effect is classy and well executed. Now go and use this on your next project!
Very good, Mr Walsh.
I like this, but I think it comes with a very slight usability issue – when I hovered over and the “Use Twitter account” text popped out, I tried a couple of times to move over and click it like it was offering an alternative sign-in method. Could be a teeny bit frustrating for an impatient user thinking they’re just slipping off the button when it shoots back in.
this one doesn’t work very well with opera…
sorry for this stupid question, i’am a newbe
but how looks the function if i have only one button
$$('.slidebttn').each(function(btn) {...
i think each is then not necessary or?
I like. However, IE not allowing border-radius kinda kills the effect. Looks much better in firefox or chrome.
@derdummkopf: How would you accomplish this without each? The only way I can see of doing so would repeat walks through the DOM to get the previous items.
@John: It may be something where you don’t show IE users this effect.
Nice little dilly hoper here David.
@derdummkopf
you could just take the function from within
.each()
and name it. then pass it a single mootooled element$("one_element_ID")
.Remember to keep the classname or it’ll not have any style
That is a really neat user interface object, I am sure clients will understand what you want from them when buttons start opening and closing for them.
Insane :) Hehe.. have to love it..
Great for exercise … Soon to be implemented on my secret project :D
Hi,
Nice work,
Can u please say what the below line will do in above code.
Specially
getPrevious
functionhow can i make it work with master/content pages?
Verry nice and simple! With a little customization it fits perfect on my site! Thanx.
How do I add a link to these? I tried but keep failing :(
How do I add a link to this button?