JavaScript Errors and How to Fix Them
JavaScript can be a nightmare to debug: Some errors it gives can be very difficult to understand at first, and the line numbers given aren’t always helpful either. Wouldn’t it be useful to have a list where you could look to find out what they mean and how to fix them? Here you go!
Below is a list of the strange errors in JavaScript. Different browsers can give you different messages for the same error, so there are several different examples where applicable.
How to read errors?
Before the list, let’s quickly look at the structure of an error message. Understanding the structure helps understand the errors, and you’ll have less trouble if you run into any errors not listed here.
A typical error from Chrome looks like this:
Uncaught TypeError: undefined is not a function
The structure of the error is as follows:
- Uncaught TypeError: This part of the message is usually not very useful. Uncaught means the error was not caught in a
catch
statement, andTypeError
is the error’s name. - undefined is not a function: This is the message part. With error messages, you have to read them very literally. For example in this case it literally means that the code attempted to use
undefined
like it was a function.
Other webkit-based browsers, like Safari, give errors in a similar format to Chrome. Errors from Firefox are similar, but do not always include the first part, and recent versions of Internet Explorer also give simpler errors than Chrome – but in this case, simpler does not always mean better.
Now onto the actual errors.
Uncaught TypeError: undefined is not a function
Related errors: number is not a function, object is not a function, string is not a function, Unhandled Error: ‘foo’ is not a function, Function Expected
Occurs when attempting to call a value like a function, where the value is not a function. For example:
var foo = undefined; foo();
This error typically occurs if you are trying to call a function in an object, but you typed the name wrong.
var x = document.getElementByID('foo');
Since object properties that don’t exist are undefined
by default, the above would result in this error.
The other variations such as “number is not a function” occur when attempting to call a number like it was a function.
How to fix this error: Ensure the function name is correct. With this error, the line number will usually point at the correct location.
Uncaught ReferenceError: Invalid left-hand side in assignment
Related errors: Uncaught exception: ReferenceError: Cannot assign to ‘functionCall()’, Uncaught exception: ReferenceError: Cannot assign to ‘this’
Caused by attempting to assign a value to something that cannot be assigned to.
The most common example of this error is with if-clauses:
if(doSomething() = 'somevalue')
In this example, the programmer accidentally used a single equals instead of two. The message “left-hand side in assignment” is referring to the part on the left side of the equals sign, so like you can see in the above example, the left-hand side contains something you can’t assign to, leading to the error.
How to fix this error: Make sure you’re not attempting to assign values to function results or to the this
keyword.
Uncaught TypeError: Converting circular structure to JSON
Related errors: Uncaught exception: TypeError: JSON.stringify: Not an acyclic Object, TypeError: cyclic object value, Circular reference in value argument not supported
Always caused by a circular reference in an object, which is then passed into JSON.stringify
.
var a = { }; var b = { a: a }; a.b = b; JSON.stringify(a);
Because both a
and b
in the above example have a reference to each other, the resulting object cannot be converted into JSON.
How to fix this error: Remove circular references like in the example from any objects you want to convert into JSON.
Unexpected token ;
Related errors: Expected ), missing ) after argument list
The JavaScript interpreter expected something, but it wasn’t there. Typically caused by mismatched parentheses or brackets.
The token in this error can vary – it might say “Unexpected token ]” or “Expected {” etc.
How to fix this error: Sometimes the line number with this error doesn’t point to the correct place, making it difficult to fix.
- An error with [ ] { } ( ) is usually caused by a mismatching pair. Check that all your parentheses and brackets have a matching pair. In this case, line number will often point to something else than the problem character
- Unexpected / is related to regular expressions. The line number for this will usually be correct.
- Unexpected ; is usually caused by having a ; inside an object or array literal, or within the argument list of a function call. The line number will usually be correct for this case as well
Uncaught SyntaxError: Unexpected token ILLEGAL
Related errors: Unterminated String Literal, Invalid Line Terminator
A string literal is missing the closing quote.
How to fix this error: Ensure all strings have the correct closing quote.
Uncaught TypeError: Cannot read property ‘foo’ of null, Uncaught TypeError: Cannot read property ‘foo’ of undefined
Related errors: TypeError: someVal is null, Unable to get property ‘foo’ of undefined or null reference
Attempting to read null
or undefined
as if it was an object. For example:
var someVal = null; console.log(someVal.foo);
How to fix this error: Usually caused by typos. Check that the variables used near the line number pointed by the error are correctly named.
Uncaught TypeError: Cannot set property ‘foo’ of null, Uncaught TypeError: Cannot set property ‘foo’ of undefined
Related errors: TypeError: someVal is undefined, Unable to set property ‘foo’ of undefined or null reference
Attempting to write null
or undefined
as if it was an object. For example:
var someVal = null; someVal.foo = 1;
How to fix this error: This too is usually caused by typos. Check the variable names near the line the error points to.
Uncaught RangeError: Maximum call stack size exceeded
Related errors: Uncaught exception: RangeError: Maximum recursion depth exceeded, too much recursion, Stack overflow
Usually caused by a bug in program logic, causing infinite recursive function calls.
How to fix this error: Check recursive functions for bugs that could cause them to keep recursing forever.
Uncaught URIError: URI malformed
Related errors: URIError: malformed URI sequence
Caused by an invalid decodeURIComponent call.
How to fix this error: Check that the decodeURIComponent
call at the error’s line number gets correct input.
XMLHttpRequest cannot load http://some/url/. No ‘Access-Control-Allow-Origin’ header is present on the requested resource
Related errors: Cross-Origin Request Blocked: The Same Origin Policy disallows reading the remote resource at http://some/url/
This error is always caused by the usage of XMLHttpRequest.
How to fix this error: Ensure the request URL is correct and it respects the same-origin policy. A good way to find the offending code is to look at the URL in the error message and find it from your code.
InvalidStateError: An attempt was made to use an object that is not, or is no longer, usable
Related errors: InvalidStateError, DOMException code 11
Means the code called a function that you should not call at the current state. Occurs usually with XMLHttpRequest
, when attempting to call functions on it before it’s ready.
var xhr = new XMLHttpRequest(); xhr.setRequestHeader('Some-Header', 'val');
In this case, you would get the error because the setRequestHeader
function can only be called after calling xhr.open
.
How to fix this error: Look at the code on the line pointed by the error and make sure it runs at the correct time, or add any necessary calls before it (such as xhr.open
)
Conclusion
JavaScript has some of the most unhelpful errors I’ve seen, with the exception of the notorious Expected T_PAAMAYIM_NEKUDOTAYIM
in PHP. With more familiarity the errors start to make more sense. Modern browsers also help, as they no longer give the completely useless errors they used to.
What’s the most confusing error you’ve seen? Share the frustration in the comments!
Want to learn more about these errors and how to prevent them? Detect Problems in JavaScript Automatically with ESLint.
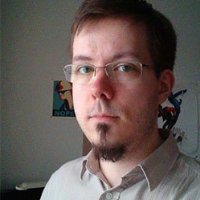
About Jani Hartikainen
Jani Hartikainen has spent over 10 years building web applications. His clients include companies like Nokia and hot super secret startups. When not programming or playing games, Jani writes about JavaScript and high quality code on his site.
To avoid RangeError errors- the best way might be changing recursions to loops, because browsers have limitations for JavaScript call stack- which is usually from 1525 (IE) to 334028 (FF).
I prepared demo to check it in your browser:
http://codepen.io/malyw/pen/pvwoyK
Some Math calculation might take more cycles- so it’s good idea to change them to loops and move e.g. to Web Workers.
That’s a great tip Sergey. It’s true that in some algorithms, it’s possible to run into the recursion limitations without a bug.
This article nails JavaScript errors right on the head. Especially the one about the Unexpected[] or {}. I’ve had that point to the jQuery library when it was just within my own code!
Thanks for sharing!
You can eliminate a lot of these errors by using a linter like jshint or eslint. It’s useful to have a linter in your build process as well as in your editor.
Yeah, I’ve been looking at these kinds of tools lately. Others like Flow and TypeScript could also be useful, though may require a bit more work to set up.
Definitely a topic I might write about in the future!
“I’ve been looking at these kinds of tools lately”? Wow, I thought they were standard practice in the industry nowadays, does anyone really still run their code without checking it with some tool first?
For what is worth, eslint is vastly superior to jshint – more rules (and the ability to write your own), and it differentiates between errors and warnings (so you don’t have to stop a build because of unimportant formatting).
I had similar experience for Javascript, and decided the problem was with the actual language itself. I realise that there is not much point to use Javascript when there are languages available which are not limited and broken and are a pleasure to use and jebug.
Wow! good information very nice article I hope it helps me a lot thanks for sharing
Thanks for this very helpful article. Locating and rectifying error in a large Javascript code has been hugely frustrating, I some times prayed for the day, when some body develops a compiler, interpreter or any helpful tool to help us debug and remove errors.
Great article! Also, I agree with Till about using a linter like JSHint. It’s one way to save page reload time, typing to open the console, finding the correct line where the error occurred, deciphering some of the more cryptic error messages in certain browsers, and teaching good practices when the linter is configured correctly.
Very useful.Thanks!
Awesome article, well done. What further complicates the problem is that each browser has implemented {object Error} in a different way! The behavior and contents change wildly browser to browser. I did a talk on these differences last year:
http://vimeo.com/97537677
Running a JavaScript Error Logging service ( http://Trackjs.com ), we’ve seen tons of crazy errors. Sometimes devices or plugins overload behavior and use JavaScript as a transport layer. For example, Chrome on IOS overloads
XmlHttpRequest
with extra properties and uses it to communicate to the native webkit client. If you mess with theXmlHttpRequest
on this platform, chrome shows tons of nasty security errors!A code highlighting editor will pretty much make almost all of these errors except the circular JSON reference go away.
er.. and the other runtime errors that i didn’t notice at first glance. :-D
Be grateful you don’t still have to code for IE6, and it’s notoriously unhelpful “unspecified error at line 1” :-O
Thanks a bunch. Saved me a lot of stress
Hi David,
I’m getting an Uncaught TypeError when a response from a jsonp request is received after the timeout I specified. Most of the time it works. However, there are times that our api server is so heavily busy. How do I catch the error rather than seeing Uncaught TypeError in the console?
Thanks! Great site!
Neil
Internet Explorer is generating the error “Unable to get property ‘chunkSize’ of undefined or null reference”. What does that mean?
I keep in running into
“ORIGINAL EXCEPTION: TypeError: Cannot read property ‘request’ of undefined”
Hi, I have a problem, that I don’t understand. I am using angular/D3/Typescript in a class that creates a chart. I use d3.evet.clientX and all is well, but in my controller it is undefined. why?
Thanks Mike
IE9 has just offered this pair of doozies with absolutely no reference to whatever the issue is, so 7,000 possible lines of code to sift through. Fun :-|
Not a js error though,
fixed the issue. Head banging time finished.
<meta http-equiv=”X-UA-Compatible” content=”IE=edge”>
hi,
Even though included necessary files am following error
“{Uncaught TypeError: Cannot read property ‘encode’ of undefined}”
am trying to create front end code editor for python mode but couldn’t make it. have had following this example to compile and execute the code.
“http://qnimate.com/create-an-frontend-editor-with-code-highlighting-and-execution/”
could anyone help me to fix it?
Looking forward
Dear Jay,
A look at this error would help
Thanks, Aldo
After getting tons of
undefined is not an object
error just surfing (I gave up on programming in 1965 in the SPS IBM 1620 era) I gave up and decided to find out what I was doing wrong. Thanks for a clear explanation that even an over the hill brain can understand. Good to know the problem isn’t all me ;-)Getting XML5686: Unspecified XML error while getting response. When response XML huge in IE11. Is their any resolution.
Another strange JS error:
Uncaught (in promise)
, pointing to the first empty (!) line of my documentThen, after A LOT of debugging, I realised that it’s caused by an unhandled Promise, rejected by an empty string!
If this isn’t confusing, what is?
Maybe it is cause of uncaught rejection with any parameter?)
You should always write caught handler with promises.
where is the problem?
The snippet above resulting error below in the handler function ;-(
TypeError: callback is not a function