Create a CSS Flipping Animation
You've all asked for it and now I've added it: Internet Explorer support! Annoyingly enough, the change involves rotate the front
and back
elements instead of just the container. Skip to this section if you'd like the Internet Explorer code. IE10+ is supported; IE9 does not support CSS animations.
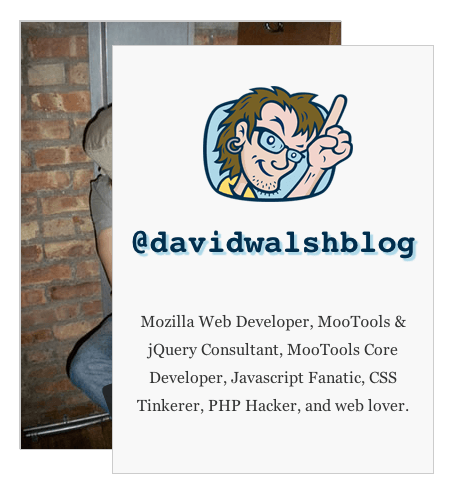
CSS animations are a lot of fun; the beauty of them is that through many simple properties, you can create anything from an elegant fade in to a WTF-Pixar-would-be-proud effect. One CSS effect somewhere in between is the CSS flip effect, whereby there's content on both the front and back of a given container. This tutorial will show you show to create that effect in as simple a manner as possible.
Quick note: this is not the first tutorial about this effect, but I've found the others over-complicated. Many other tutorials add additional styles to code samples which then require the reader to decipher which are needed and which aren't. This tutorial avoids that issue, providing you only the necessary styles; you can pretty up each side of the flip any way you'd like.
The HTML
The HTML structure to accomplish the two-sided effect is as you would expect it to be:
<div class="flip-container" ontouchstart="this.classList.toggle('hover');"> <div class="flipper"> <div class="front"> <!-- front content --> </div> <div class="back"> <!-- back content --> </div> </div> </div>
There are two content panes, "front" and "back", as you would expect, but also two containing elements with very specific roles explained by their CSS. Also note the ontouchstart
piece which allows the panes to swap on touch screens. Obviously you should break that code into a separate, unobtrusive JavaScript block if you wish.
The CSS
I'm willing to bet that outside of the usual vendor prefix bloat, you'd be surprised at how little CSS is involved:
/* entire container, keeps perspective */ .flip-container { perspective: 1000px; } /* flip the pane when hovered */ .flip-container:hover .flipper, .flip-container.hover .flipper { transform: rotateY(180deg); } .flip-container, .front, .back { width: 320px; height: 480px; } /* flip speed goes here */ .flipper { transition: 0.6s; transform-style: preserve-3d; position: relative; } /* hide back of pane during swap */ .front, .back { backface-visibility: hidden; position: absolute; top: 0; left: 0; } /* front pane, placed above back */ .front { z-index: 2; /* for firefox 31 */ transform: rotateY(0deg); } /* back, initially hidden pane */ .back { transform: rotateY(180deg); }
Here's a rough overview of the process:
- The outlying container sets the entire animation area's perspective
- The inner container is the element that actually flips, spinning 180 degrees when the parent container is hovered over. This is also where you control the transition speed. Changing the rotation to -180deg spins the elements in the reverse direction.
- The front and back elements are positioned absolutely so they can "overlay" each other in the same position; their backface-visibility is hidden so the back of the flipped elements don't display during the animation
- The front element has a higher z-index than the back element so the front element may be coded first but it still displays on top
- The back element is rotate 180 degrees, so as to act as the back.
That's really all there is to it! Put this simple structure into place and then style each side as you'd like!
A Note from CSS Animation Expert Ana Tudor
Applying certain properties with certain values (like overflow: hidden
) on the card element would disallow it to have 3D transformed children. I believe this is relevant because I got into trouble with overflow: hidden
precisely in such cases, where all children of the 3D transformed element were in the same plane, but one or more had been rotated by 180deg
.
CSS Flip Toggle
If you'd prefer the element only flip on command via JavaScript, a simple CSS class toggle will do the trick:
.flip-container:hover .flipper, .flip-container.hover .flipper, .flip-container.flip .flipper { transform: rotateY(180deg); }
Adding the flip class to the container element will flip the card using JavaScript -- no user hover required. A JavaScript comment like document.querySelector("#myCard").classList.toggle("flip")
will do the flip!
CSS Vertical Flip
Performing a vertical flip is as easy as flipping the axis and adding the transform-origin
axis value. The origin of the flip must be updated and the card rotated the other way:
.vertical.flip-container { position: relative; } .vertical .back { transform: rotateX(180deg); } .vertical.flip-container .flipper { transform-origin: 100% 213.5px; /* half of height */ } .vertical.flip-container:hover .flipper { transform: rotateX(-180deg); }
You can see that the X
access gets used, not the Y
.
Internet Explorer Support
Internet Explorer requires significant modifications to the standard flip code because it has not yet implemented all of the modern transform
properties. Essentially both the front and back elements need to flipped at the same time:
/* entire container, keeps perspective */ .flip-container { perspective: 1000px; transform-style: preserve-3d; } /* UPDATED! flip the pane when hovered */ .flip-container:hover .back { transform: rotateY(0deg); } .flip-container:hover .front { transform: rotateY(180deg); } .flip-container, .front, .back { width: 320px; height: 480px; } /* flip speed goes here */ .flipper { transition: 0.6s; transform-style: preserve-3d; position: relative; } /* hide back of pane during swap */ .front, .back { backface-visibility: hidden; transition: 0.6s; transform-style: preserve-3d; position: absolute; top: 0; left: 0; } /* UPDATED! front pane, placed above back */ .front { z-index: 2; transform: rotateY(0deg); } /* back, initially hidden pane */ .back { transform: rotateY(-180deg); } /* Some vertical flip updates */ .vertical.flip-container { position: relative; } .vertical .back { transform: rotateX(180deg); } .vertical.flip-container:hover .back { transform: rotateX(0deg); } .vertical.flip-container:hover .front { transform: rotateX(180deg); }
With the code above, IE10 will rotate flip the elements as expected!
The CSS flip animation has always been a classic, representative example of what's possible with CSS animations, and to a lessor extent, 3D CSS animations. What's better is that there's actually very little CSS involved. This effect would be really neat for HTML5 games, and as a standalone "card" effect, it's perfect. Can you think of anything else you'd use this for?
Sounds cool and all, but its kind of hard to check out a
:hover
effect when you’re using a touchscreen. #Justsayin’ ;)I’ve updated the post to work on touchscreen Torkil!
it still has issues with touch screen. For example. The first image works in the first touch but it stops working thereafter and randomly works from there on!!
Hi, my name is Trace I am using this css animation in one of my projects. I would like to attribute/acknowledge you in my code please give some information on how you would like that to look like. Thanks
As TORKIL JOHNSEN said already. Needs logic for touch devices. The example page does randomly flip the one or other card.
Besides that, cool stuff.
Can it be found on github?
The post has been updated for touchscreen.
It’s not on GitHub — all of the code to create the effect is in this blog post.
Firstly, this is a really useful effect, thank you Mr. Walsh!
This might sound odd and most likely has to do with the WP Template I am using, but it is boggling my mind, so here it is –
I’m working on a client site, which will be live shortly (www.stratacus.com/services/)
The flipping works great on my Android, however on my iPad – it works a bit sporadically.
The effect works fine on initial page load, UNTIL I scroll a bit down the page. Once I scroll, I need to press and hold on the image in order to see the flipping effect.
Upon scrolling more with my finger, touching anywhere to scroll, you can tell that the image wants to flip but it doesn’t… Additionally,
If I press beneath the picture after scrolling down the page (basically touching where the image was placed on the screen originally, upon initial load at top page height) the picture then flips!
Very odd behavior and I know it has something to do with the scrolling JS on this template, maybe CSS breakpoints or something, or the template itself somewhere down the line, but I cannot for the life of me wrap my head around it…
I know it isn’t your functionality that is wrong, but something on my end conflicting with it…
I will be leaving the effect regardless because, when it does work, it’s awesome, but if you or anyone else has any insight, that’d be more than appreciated and I’ll be forever indebted, just simply because it is really bugging me at this point. (PS I believe by the time someone will be reading this, the site listed above will be live.)
Thanks again for this great tutorial, any help is appreciated, and feel free to contact me directly if you think you suspect what is causing this.
Thanks a lot guys,
– Paul
paul@unstech.com
Did you have any trouble with the absolute positioning not stacking correctly in a smaller browser window?
Slick! You can even add a css transition delay so it stays flipped for a little after hovering, which gets close enough to using a click event with javascript.
Can you tell me how please?
In Chrome your demos don’t work how you describe. The back is never shown. Rather you just see an exact mirror image of the front.
e.g. When I flip the basic card, I see “tnorf” not “back”
On what platform? Just tested on Mac/Chrome and everything looks correct.
Windows. http://i.imgur.com/sZNPZ.png
I faced the same problem. Worked in Firefox but not in Chrome and Safari. I then went on and added vendor prefixes to all the CSS3 properties such as backface-visibility, transform-style, transition and transform. That sorted it out. Still doesn’t work in Opera and IE9 though.
-webkit-transform
have some bugs with CSSposition:absolute
. They can’t work together. Keep in mind.Great article, works smoothly on Mac/Chrome.
I think this sentence may be wrong though.. “you can’t pretty up each side of the flip any way you’d like.”, or why can’t you, given that you can target .front and .back?
Ugh, major typo — that should have said “can.”
How might this work in a wordpress scenario where posts are displayed in a thumbnail grid where the featured post image is on one side and the post meta is on the other side? how would the permalink work?
Hey!
Nice article, David. However, there are a couple of things we can do to smooth over cross-browser inconsistencies.
1) There is inconsistency in implementation of perspective property in Firefox 15 and earlier;
2) Also, in Chrome, when you have a complex markup you can encounter issues with positioned children of the indirectly transformed elements(.front and .back in this case). Absolutely positioned elements would flicker and shine through flipped parent during transform, whereas relatively positioned ones would do likewise and even worse — they’ll ignore parent’s backface-visibility and “shine” through it in mirrored way after transform is complete. Workaround is simple — you have to enable hardware acceleration on the element.
So, for the above code you would add:
But what about IE? What if we want >=IE10 users to have this cool effect (and I think that right now, we, as web-developers, should care more about IE10 than about IE7 or IE8). It comes with Window 8 this October and it won’t support preserve-3d keyword. How do I know? Because I have W8 running on virtual machine; and they actually were not planning to add it for the release of W8 http://msdn.microsoft.com/en-us/library/ie/hh673529(v=vs.85).aspx (see the note under
Transform-style
property heading).Having that said, we need to modify this code a bit. Instead of flipping one wrapper-element, we’re going to flip both sides of the card simultaneously.
And don’t forget to add a fallback for browsers which doesn’t support 3d-transform (which is simple if you’re using Modernizr, you just need to describe alternative behaviour using no-csstransforms3d class).
After all of the above (as of September 2012):
a) FF 15: applies perspective instead of flattening transformation to 2d;
b) Chrome 22: works fine if you took care and applied the fix(or if you don’t have to bother about positioning inside the front card);
c) Safari 5, 6: when it comes to CSS3, there is no browser better;
d) IE7-IE9: no effect, instant show/hide behavior;
e) IE10: flat transforms with no perspective. And we’re hoping that when support for 3d-transforms is added, our code is going to work as intended.
f) Opera 12.02: instant show/hide without the effect, but we’re hoping too =)
Thanks for your IE10 fix, Artem. It was a help!
http://codepen.io/KurtWM/pen/xhvHe
Thanks for this.. too bad david walsh can’t update the blog to show how this should be done. Took me an extra 30 minutes to figure this out., until i saw your post.
Artem, you’re very insightful and effective comment was a life saver. Was specifically having issues with IE and like Leet Ice, found your code and it fixed my situation immediately.
David Walsh, please consider updating this post with Artem’s code. Your CSS Flip Animation is wonderful and adds a lot (thank you!), but there are still many people using versions of IE that don’t play nice with it and I’m sure Artem’s code could be invaluable to them if it was easier to see.
Thanks Artem, this got David’s code working for me!
I am trying this out and the code from Artem was great except I can’t figure out how to position more than one container on the same row. I just end up with all the containers stacked vertically on top of one another.
Can anyone help me figure out what piece of code I can insert to fix this? I am a novice with this stuff and only understand a bit of it so all my attempts have failed.
Thank you in advance for the help!
I’m having this issue as well. It works fine on a large screen, but on smaller devices the
position: absolute
in the.front, .back {}
classes won’t allow the columns to stack vertically.Hi Amy,
I used bootstrap’s
col-ms-4 (div class="back col-sm-4">)
to position more than one container on the same row.Thanks man, these changes fixed all of my issues!
Artem’s fixes gets it to work on touchscreen. Hurray!
This works great with Safari! Thanks so much!
Awesome David! Any good way to show the backface-visibility during the animation? Every way I’ve tried has had pretty bad performance/flickering.
This is awesome !
I add this feature (with little adjustements) on http://datagamer.fr/ ;)
I tried changing this to flip on the X axis, but I cannot seem to move the origin to the center. It now flips out the top of the container where the back cannot be seen. Any idea what special sauce I need to add to the CSS?
Anyway we can turn this into a 3D look like this site’s links http://www.pukkelpop.be/nl/bestof ??!!
Post coming next week ;)
Hey
Thx David for nice effect, it gives the site another dimension. Keep up the good work.
Also thx for Artem,thx to u it works also for me;)
Hey David, awesome work! How can I trigger the transition without hover, but by clicking a button or something?
Create a class with the
:hover
selector properties, then add that class to the element that should animate.Hi, how do you change the codes if you want the card to automatically flip by itself, once, without any mouseover/click?
Please help!!! i have an urgent project due tomorrow!!!
Hi,
I have been trying every imaginable way to make the card slow down when flipping over.
I would like it to stay “flipped” some second more before turning over again.
Transition-delay, for example, does not seem to work…
Does someone here know a way how to do it (make it flip over completely & then stay flipped a bit longer after cursor moves)? Please share :)
Thanks guys!
Hey David, I just made a CSS-animated greeting card where the card comes out of the envelope and everything. I found your examples really helpful. Thanks for posting them! You can see my project at http://markroland.com/project/CSS-greeting-card
Thanks for posting. Really useful. Using it now.
works for me on firefox, but not on chrome and safari, while your demo works on the 3 of them, any ideas?
thanks!
Alright, now that i applied all the fixes it works on chrome and safari but bugs on firefox.
it’s these two that create the difference: with works great on firefox and not on safari chrome, with bugs on firefox
.flip-container:hover .back, .flip-container.hover .back {
.flip-container:hover .front, .flip-container.hover .front {
thanks in advance!
You probably need to prefix perspective, translate, and transform properties to -webkit-.
Hi, Nice work!
What scripts are included for this to work?
Best,
Jon
No JavaScript necessary — just CSS!
I been searching for something simple and understandable like this!
Question: Can I use this on a commercial site?
thanks
Absolutely Elly! Good luck!
PERFECT!! That was awesome! I was looking for something like this. THANKS!! :)
Nice.
Knowledge – add prefixes for demo downloads.
Thank you!
Does anybody have this working in Chrome on Windows?
I’ve managed to get results on:
Mac: Chrome, Firefox, Safari.
PC: Firefox, Safari
IE and Opera are a total bust. I find Window’s Chrome bizarre. I’ve got text displayed correctly, but upon flipping, it’s just rotating the text completely instead of showing the hidden div.
Hey,
Does’nt work for me with the placed css/html. Is there any javascript needed?
I work on Chrome/Windows. The demo cards rotate well.
Hi guys
Where can I get a working version of this with the vendor preferences because, its just not happening for me on Chrome :(
Are you properly vendor-prefixing properties? http://davidwalsh.name/vendor-prefixes
Hi David,
Really cool post. I tried it and works well in Firefox, Chrome and Safari but not on Opera or IE9. Is there a way to get it working? At least on Opera?
Hi,
I am new to CSS. Could u explain me what is happening in the following lines. I cannot u’stand the work of the selectors.
.flip-container:hover .flipper, .flip-container.hover .flipper
Thank you.
Hi,
nice article, clean and simple. One request, when I compare the animation to the live tiles on my windows phone I notice that the windows version actually goes beyond the 180 degrees briefly then eases back into position before facing flush with the user. Is there a way to recreate this with an animations / keyframes declaration?
Thank you, its works amazing. I do only fight with IE10. I was adding the -ms prefixed and it does only rotate the image on first pane on hover. Anyone know how to fix it?
Hi David,
Thank you for this tuto, I have some issues with touch stuffs.
It works as a charm on a laptop, but not on iphone.
Could you take a look at my code if you have 5min to loose :)
Thank you
In fact, the first touch flips the div but the second dont turn it back.
Check this on http://www.l1nkus.com from an iphone.
Hey David, one question… Is that your code on CodeCanyon? lol Well just had to ask since the codes in the source are the same. The one in CodeCanyon was published not too long ago, and I see the one on this page was published last year.
http://codecanyon.net/item/flipping-cards-3d-with-jquerycss3/3726635
I’m trying to get this working with a container that is filling a div 100% height using position:absolute;top:0;left:0;bottom:0;width:300px. Not having much luck.
The code I’m trying:
https://gist.github.com/anonymous/5081539
I expected it to work when I replace “x-effect” with “effect”, but it doesn’t. Anybody knows how to get such a transform working?
no IE
thanx. it works nice.. thanks a lot..
Hi,
I tried to implement this (great tutorial btw). Unfortunately I ran into an issue with background on the div.flipper – Does anybody have a solution to this?
http://jsbin.com/amokax/1/
Great work!
This isn’t working on internet explorer 9. IE9. Could you please help me out?
Thanks David this is awesome, just what I’ve been trying to figure out how to do.
I do have one question though, is there a simple tweak to keep the transition within its containing div?
SoundCloud manage to do it with their widgets: https://soundcloud.com/pages/widgets
Is this a simple modification, if so, could you shed some light on it?
Thanks! :D
Don’t work with Samsg s4 browser and Samsg s4 Chrome
Seems to no longer work in firefox (Mozilla/5.0 (Windows; U; Windows NT 6.1; en-GB; rv:1.9.2.24) Gecko/20111103 Firefox/3.6.24).
I’ve tested all the examples and none of them seem to work in FF but work in Chrome and IE.
Any idea what has changed in FF to break it?
Works for me in current Firefox and Firefox Nightly.
Hi David,
Thanks, very useful tutorial! But i’ve still some questions. How can I make this flip-effect clickable instead of the hover effect that’s used in the tutorial? I want to create a simple button and when you click on that, it should flip.
Can you give me some more info? Thanks!
Hi David,
I tried this on few browser, Here is the result.
Firefox 21.0 – Works Fine
IE 10 – Flip the text and image, not showing back
Opera 12.15 – No animation
Chrome 26.0 – No animation
Safari 5.1.7 – Works fine
Hope you can find a fix for IE, Opera and Chrome.
Just tried on Chrome 26 on Mac — worked great!
Hi David, Nice work
Just IE 7,8,9 – i see its not functional. If it works there – it would be great
Thanks for the clear tutorial David!
Your demos work fine on chrome 26 for me but I cannot reproduce a working version on any browser.
When I attempt to create my own version, the front and back divs are both viewable all the time, hovering over the div doesn’t initiate any transform. It’s basically a complete fail for me after a few hours of hacking.
Is there any hidden javascript or extra code that isn’t included in your walk-through demo? It would be nice to see a jsfiddle version with all the code necessary.
Thanks again for all your work and selfless contributions to the community!!
Argh… please disregard the above comment. Got it working by adding the appropriate vender prefixes to the css.
Thanks for the clear tutorial David!
I have a question about CSS3 animations. Is there a way to build this animation without using hover or click functions? I´m new using css3 and want to study animations like transitions, to use in games.
Sure Rennan. You would use CSS animations (via keyframes) and then add and remove CSS classes with the animation settings on them.
I rewrote this without using preserve-3d, because Internet Explorer 10 does not support that keyword at this time (Source: http://msdn.microsoft.com/en-us/library/ie/hh673529.aspx). If anyone is interested, my code is available as a GitHub Gist here: https://gist.github.com/smockle/5550032 (CSS and SCSS available).
Big thanks! Took some browser sniffing to solve my problem. But it is solved!
great tutorial. i am working on a card game and i wanted to use this code but didnt figure out how to set flip animation with “onclick” function. can anyone help me ?
This is broken for me in the newest Chrome Canary (29.0.1507.0). The back side of the card doesn’t appear when flipped. Anyone else see this?
@Semih, Yeah, I was also trying to use a click event to set the motion in action, but I jus’t can’t find a way to do it. Tried using an eventListener, but I’m not getting any luck…
@Semih and everyone else who want to have to flip effect activated by clicking, it’s as simple as instead of having ‘.flip-container:hover .flipper’ just rename the class to ‘.flip-container .selected .flipper’ or any other name you see fit and then just have that style applied with click events..
I’m testing some ideas on my dev space and would really like to use this to flip from one image to another. I’m using WordPress and just cannot get this to work – could you help please?! Apologies, I am a complete novice…!
I had nothing but trouble in Chrome 25, even after adding vendor prefixes.
I went with this $6 solution on CodeCanyon, mentioned above, and finally got it to work:
http://codecanyon.net/item/flipping-cards-3d-with-jquerycss3/3726635
Highly recommended – tons more features including automatic, timed animations, different flipping directions, etc.
I have same issue with the chrome Version 27.0.1453.110 m. “The back side of the card doesn’t appear when flipped. Anyone else see this?”
I have used this flip effect from my product page. Please help.
I used your instructions for a personal project, I only added this thing to work with IE10 and touch devices:
it seems that ontouchstart doesn’t work with IE ( i know, I shouldn’t worry about MS anymore). I left both, but don’t know if that’s correct.
Dear Gabirel
You’re probably using css reset file.
I recommend to test without css reset.
Having a strange issue. I am testing a mobile design for my businesses website, and the flip works with hover when I use Safari on my desktop and use the iPhone user agent (to get the proper style sheet).
However, when using it on the actual iPhone device, it flips on the first tap, but does not flip back on the second tap. The same thing happens in Chrome on my Galaxy Note 2 with taps, HOWEVER #2, if I use the S-Pen (which allows for hovering), the flip works both ways just as it does with :hover on the desktop browsers.
I am using using the inline “ontouchstart” code just like your example. in fact, all of the CSS i am using is identical to your example, just with renamed classes. Not sure what the problem is, since the code is exactly the same as on your demo page, other than the Width being 100% instead of pixels.
i also added a javascript alert for touchstart (using jquery ON), to see if the .hover class is being removed properly, and it is. It almost seems like the CSS is not properly updating once the class is removed.
http://monarchmotel.com/rooms/the-boat-room/?theme=monarch2013
I may be running into other issues now. It is a great effect, but i’ll probably skip it for my mobile site for right now (your own demo is having weird issues on my Android phone with Chrome, but not with Safari on my iPhone, weird).
For me, it works well on mobile when I remove
.
can anybody help me with ie9 and the actuall chrome versions doesnt flip it to the back.
thy
On transition delay.
Can anybody help to add the code so that the flipped side remains after having hoovered.
I tried multiple ways but can’t quite figure what’s going wrong. Thanks.
Hi David
this looks amazing
I’m currently working on iPad/iPhone apps that could use this exact feature, but coding is not my strong suit – i’m having a little trouble applying it to my imagery and allowing the interactivity
Dear David !
Please tell something about IE ? how it can be work on IE because without IE this work is useless for developers.
David, this is sooo cool. Thanks for sharing this priceless trick!
It took me awhile to get this through to live but I figured you might like to see how I implemented this.
I even made it degrade to a tool tip for browsers that can’t handle this.
https://store.enmasse.com/tera
In SASS:
Any idea how to make this work with 3 flip faces (not 2 like there). I need it like this:
First face
Toggle button -> Second face
Second toggle button -> Third face
Hey there,
and thank you for the awesome css effect!
I tried using this in combination with my first steps into flexible layouts, where I have multiple divs of different %width and %height dimensions relative to the browser viewport (think metro ui style tiles). Is there a way this might work without the absolute pixel dimensions in the “.flip-container, .front, .back” classes?
Thank you in advance!
Kind regards,
MWill
Delete width and height properties from #f1_container, and then in jQuery:
It works perfectly! Thanksies!
hello,
is there a way to make the image a link still using this css?
Thanks, David! This tutorial is flipping easy :)
I’ve used it (verbatim, for the most part) to add a shuffle button to my photo website.
Take a look when you can!
And thank you.
Oops. The website is at http://www.blindinglyordinarythings.com
You can see the styled css flip on any of the photo pages.
Thanks!
hi, nice tut and work… but is there an option to have the flip animation but with two different sides, not just one side flipping around ?
thanks in advance
there are some mistakes in the code but all in all very nice :-)
yep this is a real pain in the backface-
one way to resolve, when the anim has finished just set display none on the rear facing element. You have to set display block again before initiating the next animation. Not great but fixes it.
Hello! I love this script – thanks so much for posting it!
I too am trying to make the images flip using a mouse click rather than a hover. I read through the comments and still couldn’t figure out how to do it.
I tried using javascript and changed my :hover states to .flip-container.hover .front and .flip-container.hover .back, gave my flip-container div this property:
and added this javascript:
But I keep getting errors and it just isn’t working for me. Anyone have any ideas?
This is the right website for anybody who wants to learn more about this topic. You understand so much understanding it is hard to not to agree with your viewpoint — not that I really would like to. You definitely put a new spin on a subject that has been written about for ages. Wonderful content!
hi dont know if the source code is corect if i use it it dont work but this css those frome the demo: for tohose who it wont work on crome or safari
Subject: IE 10 Problem
This is really great and I have it working with chrome. I took the code from the demo which Valeri posted and that fixed it in CHROME, but, it would not rotate at all in IE 10. I then applied changes offered by Artem Ivanyk. Those changes caused it to rotate 360 degrees really fast in CHROME. Great if you want a subliminal message in CHROME. In IE 10, it rotates 180 degrees but the back side is in reverse. The print is backwards. If anyone has this working in IE 10 AND CHROME please post the solution. I could use it. Also, the current Demo as of this post is not working with the IE 10 version provided with all Windows 8 computers.
Hi David,
thanks for the nice article. In Firefox and Safari it looks nice (2D and 3D, respectively). But on my iPad (retina) the vertical flip seems broken.
Please check out this screenshot: http://www.borislau.de/files/vertical_flip.jpg
Do you already have a fix for this?
Best, Boris
Hi I wanted to see if there is way to get the image to flip every 10 seconds on its own?
and is there a ie 10 fix il be happy if it wil flip as long it dose somthing
hi. very thank you . it was very helpful for me
Is it possible to make the flipped image be a link? So that on a mobile device tapping the image flips it and that image is then tappable as a link?
Great article! Artem Ivanyk, your input came in handy as well when it came time to get my animation working in IE10. Here it is and it works in IE10, Firefox and Chrome. I haven’t tested in Safari. This version animates itself, rather than using hover.
http://codepen.io/KurtWM/pen/xhvHe
Can you tell me whether this code released for use under a particular license?
Use it however you’d like!
I’m trying to create a nice Responsive CSS flipping effect on a banner. I only want the effect to occur on the left side image.
here is the html:
here is the CSS:
How can I make it work so that the left image will also flip when hovering over the right side image ? (while keeping both images responsive)
I sent you an email earlier today regarding a question for a responsive 2 image flip CSS. Forget about it. I figured it out by myself. Thanks for your help anyways.
Awesome!
Good job, David!
I’m trying to implement it on a page, and when I manage well enough.
What I want to achieve is that the animations can also be made at the time of loading the page.
Can you do?
If so, you would be so kind to tell me how it could be done?
Thank you!
I can’t get this to work on Firefox. I feel like I added the correct prefixes but I’m new to this. The animation just keeps flipping back and forth. Works on Safari and Chrome. Demo: http://www.wienerwedding.com/wedding_party — I’m already live, please help!
Paul, instead of writing
you can write
and it will work fine.
Just add
px
to the value.For the toggle flip, what would the jQuery script look like?
its very good, but i cant see to get the right/bottom border outside of the image prior to hover
Don’t works in IE10 & 11. Any idea how I can only display the backside in IE10 and up?
Hi David-
I updated OS X to Mavericks last night and noticed my version of your card flip code was broken on my development site in Chrome 31. I spent the past hour trying to figure out what was wrong, so I came back to your original article and realized the demo no longer works on your site either! Have you noticed/heard of any issues with Mavericks (10.9) and Chrome 31?
The demo works perfectly in Safari.
Thanks!
-Nate
Hello everyone! I am trying to apply a horizontal flip effect but it doesn’t work. I believe that there is a problem with the backface-visibility. Please help! Here is the code:
Great read once again David,
How would you go about making this responsive?
Or is there possibly anywhere this has already been made responsive I can see working for help?
Many thanks
Has anyone adapted this to flip through a calendar which has images above and month below: i.e., each page is a double-side printed, but all files are individual.
Hi David, awesome tutorial. How do I ad some zoom along with flip ? Just like in iOS skype application.
Hi, I’m new and I have a question. Can your CSS flip technique be used on header or P tags ?
I found it working on Chrome with the code of Valeri look at my sample at http://theweeblytricks.weebly.com/8.html
Great love it
not working on IE 11 down though!
FML!
I’ve used this method on my websites some months ago and it was working normally, anyway currently it doesn’t work anymore, even in the david page it is not working as supposed, the back content is not displayed correctly, anyone has a fix for it? I tried managing opacity and
z-index
but the back content pops up without transition… too ugly…This doesn’t seem to be working with Chrome (33.0.1750.46 beta on Ubuntu) where it flips, but the backside doesn’t show up.
Of the few 3d flip effects available on the web, this one is the best and easiest because it doesn’t use jquery. But it doesn’t work on IE, which is a major fallback. I wonder why is Codecanyon’s version working for all IE versions whereas here we are still struggling for the solution.
… There seems to be a solution for all browsers: http://css3playground.com/3d-flip-cards/
But even if it seems simple, it is very difficult to adapt to David’s script (at least I haven’t managed so far).
Probably everybody knows this (I didn’t): jQuery animations use the computer’s hardware, while CSS3 transitions and animations use the browser’s rendering engine. This is one of the reasons that jQuery animations work on some browsers that don’t support CSS3 transitions.
So that’s the reason why David’s script doesn’t work in Explorer. Though it seemed to be its best feature, its ability to work only with CSS and no Jquery is its weakest point!
Quick note: This post has been updated to accommodate for Internet Explorer and now works in all browsers!
I’m trying out your demo in IE9 and it doesn’t seem to work at all. Did you try IE9?
Thanks for the demo!
I’m a novice in css (so correct me if i’m wrong) but i think i discovered something in your demo that could be wrong: if the perspective value has no unit, the perspective effect doesn’t apply to Firefox (tested in Firefox 26 & 27). The flip effect does work though.
If you append an unit to the value (“1000px”), the perspective effect is applied in Firefox.
You are right. According to the spec a unit should follow the value unless it is zero.
http://stackoverflow.com/questions/11997177/using-units-on-css-perspective
I hoped that the code in my previous comment would be formatted as, for exemple, in the Sotiris Iliadis comment. It didn’t worked as planned ;-)
Can someone tell me how to format code in comments?
Thanks.
Hi David and all,
I’m a big fan and follower of your stuff and this code rocks!
I wanted to share with you my implementation of this on a commercial site I have just worked on.
With some nifty additions one can achieve also a delayed effect on nested flippers, check out the front page here and hover over the larger image grids!
http://www.outdoorequipped.com/
Works in all the latest browsers!
Thanks so much for the clean and understandable coding – really cool – CSS is becoming ever so powerful now :)
I am interested in this effect for my WordPress site that I am working on, but have no knowledge on how or where to put this code for this flip animation. Any and all help would be appreciated – where do I place this code, etc. A “CSS For Dummies” explanation will be helpful (if possible) – again, no knowledge on how to code, just the very basics!! Thx.
This is great! In regards to mobile, the current script works with touch events but how about when there are multiple boxes with this effect.
Situation:
I’m doing a list of services with this card effect. Each card will have a title and image of an individual service on the front, and a description on the back. How can I customize the script to flip previous viewed card to the front position while clicking the next card to view the back.
Thanks.
Hi David,
Thanks for this wonderful post. Had a question.
Is there a way to find out in script which side (front or back) is visible at a given time?
Of course, you can check the value of the
transform
property. If the value isrotateY(180deg);
, you’re seeing the back.Hi David,
This works great for me on desktop; however on iPad if I tap a tile and it flips & then links to another page, when you “go back” to the original page, the tile is still flipped. Is there a way to have the tiles in their original state on “go back”?
Hi David,
I was just following up on my comment above sent on the 25th; is there a fix for that bug?
********
This works great for me on desktop; however on iPad if I tap a tile and it flips & then links to another page, when you “go back” to the original page, the tile is still flipped. Is there a way to have the tiles in their original state on “go back”?
********
it is worth mentioning, that this code only works as nice if it is put in style tags directly inside the page. If it is in a css file maybe even with an import, you end up with a weird flick when the page loads. E.g. the content of front and back is loaded first, and then gets initially animated to flick the back to the back
It just wont work for me in Chrome, Safari, Opera – only firefox or IE. Is there something I’m missing – all CSS looks fine to me as updated but can’t get it to work. I think others have had issues but I simply cant see where it is coming from. The front and back content is flipping verically – I’m not sure if this makes a difference?
Otherwise happy with results! Just want it to work in multiple browsers. CSS here:
Thanks in advance!
You need to add vendor prefixes: http://davidwalsh.name/vendor-prefixes
Having failed getting it to work (Vendor Prefixes), viewing, copying and pasting source of the demo then modifying it looks amazing! Thank you.
Hello!
The code works perfect. But when having multiple instances. On tap flips two a time.
Cool effect and tutorial, thanks… I have a problem using many of this together on the same line…
they are always placed one under the previous, not on the same row.
any help?
‘float: left’ solved my previous question ;-)
Earlier you stated you could make this an on demand effect with a javascript css class assignment. however, when I tried that it didn’t work. I think I’m getting confused by the fact that you consolidate a lot of classes and states in a single css declaration. I have the effect working on hover in all the browsers I have tested in but I’d like to bind it to a button. Any clarification you could provide on your code would be most helpful.
Man, about the “overflow:hidden” stuff – you are completely wrong.
If overflow:hidden works badly for you – it is because your container has a zero width/height. Because your “cards” have position:absolute, they do not set their parent’s dimensions.
Can you give an example of a clickable link on the back of your example?
Very radical David going to try and implement this right now.
Hey, is it possibble to make it flip to a random card.
So i can make a random card generator and every time i flip to back it will show a new random card?
There’s a lot missing on this page compared to the examples and it’s not just vendor prefixes. Works ootb in Firefox, but Chrome is a nightmare.
Can anyone help me get this CSS Flip code to work. Much Appreciated. http://production.macniche.com/
Im trying to get this simple CSS 3 Flip animation to work. But it’s not working for me. Can anyone help me? It would be appreciated. I copy the exact code for my css file which is flip.css and used the exact hml provided.
http://production.macniche.com/ I would even pay for help. Thanks.
Drew, the flip animation is working for me on your site using the latest Chrome and Chrome Canary. Which browser are you using?
I’m willing to be that Drew isn’t adding CSS prefixes.
Awesome example. Took me while to find the right code that will work on IE. I’m baffled at how your example works in IE 9 and IE 8? I’m looking at it through IE 10 emulator. Your example works but mine doesn’t. I just copied your code. Is there additional js or polyfill used?
Completely buggy in the new Firefox 30 and Nightly 33 and Aurora 32. Soooo…doesn’t work with any of the new firefox releases.
Thanks for this awesome code.
It worked perfectly in FF, but it wouldn’t work for others… so I fixed it.
This version is for JavaScript controlled flipping, based on a user’s choice, not hover enabled!
Firefox 30 now also needs the MSIE10+ code to work.
Finally a code that works!
How can I flip the entire container? at the moment just the front and back flip. I have added a shadow to the container which gives the look of a card, so I would like to flip the whole lot.
Awesome bit of code so thanks for posting. I did get an issue with Firefox though and pin pointed the issue to the:
The issue was that the front image just flipped and didn’t show the back image. Removed this css and it works as it should. Fine on the browsers i’ve tested.
The issue only happened on the site i’ve built so its one i’ve put in, but thought i’d share just incase others get the issue!
Thanks again!
Thank you, Jon! Scoured this entire thread and your fix was the last piece for me to make this work across all browsers.
Hi.. nice effect.. But on windows phones you gotta touch and hold for it to flip, and on release it flips back.. Is there any way to make it flip just by touching it like on other touch devices (without holding it down)?
Any help will be appreciated.. thanks
Love this code, thanks very much David.
I am hoping someone can help me with changing mine so each block rotates onclick rather than hover. I’m really not sure what I need to change and what javacript to add to my page to make it work…hint and tips would be greatly appreciated.
http://pixelperfectdesignstudio.com/pibworth/?page_id=56
Great work!
Only a little mistake, in
should be:
http://stardesign.com.pl/blog/
Hi,
I want to add three face in a card and two flip it two times. plz help me in implement this.
Thank you.
Hi
I have tried your code. For me it is working fine in IE, Chrome and Firefox. but not working in Windows safari. I have tested your demo page also. it is working fine in Mac Safari. but not working in Windows safari.
The issue is, In windows safari browser front face only flipping, backface is not visible.
Please help me to fix this issue.
Thanks in Advance
Strange bug in the current Chrome: Once flipped, the .back shows ok, however only the right hand side of the .back div (exactly down the middle, pixel perfect) accepts any pointer-events. I have a centred link and only the right half of it is clickable (or even shows the pointer cursor)? Anybody else seen this?
Newest Firefox (31) just need this to work again!
THANK YOU SO MUCH!
Anyone having an issue with the text being blurry when the transition has finished?
This is a fantastic trick, just had a look at the demo. Gonna have a go at this later on my new ipad and see what I can flip! Thanks
Hi! How can you change the Hover to something like a
onclick
?For a dirty css-only solution you could use the active class, but you’d have to keep it pressed to see the animation complete…
Better go with js adding a specific class that starts the animation as David described in his post.
Hi, looks like this animation is a bit touchy in regard to browser
z-index
code updates :) , the version below have been tested on the latest builds available September 2014:* Chrome 37 – works 100%
* Firefox 31 – works 99%, sometimes a side is partially covered by a background color overlay, likely caused by my .front/.back content
* Safari 7.0.6 – works 100% (was having the same issue of @Keshav with the original code, this one works)
I’m displaying a complex canvas editor with selectable/resizable elements on each side, if you were having issue with elements not responding to click events anymore (see @Dom above), this version fixes that too.
Thanks for this article. I am using this with content divs and not images. When doing this, I encountered a weird issue where elements on the left hand side of the “back” would not be clickable. To fix this, it looks like translateZ(1px) has to be added. I found the solution here: http://stackoverflow.com/questions/10886656/webkit-transform-blocking-link
Cheers!
Thanks Darren, after reading all the comments and trail and error, this finally worked for me! :D
For some reason it is not working in IE in my end. Only Chrome. Please help, I’ve tried all the suggestions above.
Hi David,
Thanks for fantastic article. I have used it in my current project. Its working perfectly on all the browsers. But…, when I access this flip from safari which is in a virtual machine, it is very inconsistent.You can test your demo in safari + VM (windows7).
I haven’t found a CSS card flip yet that can work inside of a table. Do you know the reason for this?
I have this implemented on a site that I’m working on right now. Great work on this. Only problem is when I insert the IE fix, it breaks the Chrome/Firefox functionality. Also, after implementing IE fix, text on the “back” of card is all backwards. Do I need to reference a different stylesheet in order to load IE fix and any way I can fix backward text? I’m using Dupal Omega subtheme. I wish IE would just go away. LOL. Any ideas on how to solve this?
This is EXACTLY what is happening with me. If you find a fix, please post back here.
David, any clue?
Hi.
I have some troubles with it:
1)
ontouchstart="this.classList.toggle('hover');"
won’t validate.2)when using then the 3d animation will disable
Thanks for this! i think these sort of designs would be great for limited screen space, like mobile. Probably would want to swipe to spin rather than touch.
Thanks for sharing this. I wrote an AngularJS directive that supports variable image sizes. Using this directive along with a slightly modified version of your markup/css makes it easy to place these throughout an application:
http://codepen.io/ronnieoverby/pen/EaYdvq
I was able to simplify this and still support Chrome 39, Firefox 35, Safari 7, and IE 10-11 (and degrade gracefully in IE 9).
Demo: http://jsbin.com/xomotozeja/1/edit?html,css,output
Relevant CSS:
Hi there, thanks so much for this tutorial. I’ve gotten pretty far as a web newbie in using the card flipping trick for a portfolio website. However, I’ve gotten stuck because it seems that the card flipping does not work for me in Safari. It works fine in Google Chrome, FF, and Opera though. This card flip is when you click the card. In Safari, the card does not show the flip animation effect although after a short pause, the back of the card is shown.
My current website is here: skndesigns.co
Any help would be greatly appreciated.
How does one make it so the flip animation occurs on Mouse Click only (not mouse active). plz help!
This brilliant, but for the life of me I can’t get it to centre align horizontally. Any help would be great.
Where you say:
With the code above, IE10 will rotate flip the elements as expected!
I read on this page ( ) that:
Note that IE 10 and up DO NOT support conditional comments at all.
So would adding a conditional link to ie stylesheet work?
Thanks in advance
Hi David, Thanks you so much for the tutorial. I guess this works perfectly if we know the size of the elements. Like in this case, you have set it
.flip-container, .front, .back {
width: 320px;
height: 480px;
}
But, what if we don’t know size of front and back i.e let it scale dynamically. Then how do we make sure that div element that follows flip-container doesn’t end up on top of flip-container. Because making front and back “absolute” takes them out of document flow?
Is there a way to let the next div flow after flip-container.
Here is the jsfiddle with example
http://jsfiddle.net/zk81h12u/4/
That is exactly my problem as well. Have you found a solution yet?
Hi David,
Bit to ask I know! I’m trying to create a flip horizontal image animation just like yours to include a players (cricket player) pic on the front and text on the back, just like yours. Being an absolute novice this is proving difficult. Any chance tongue in cheek you could provide me with the code, so I can add the players pic (front) and text (back). I’ve fiddled about with your code but I’m not a coder, no probs if you can’t find the time I’ll soldier on.
Please use my email to respond.
Cheers.
Mike
Hi David,
Any response to my previous email?
There will be a drink in it for you!
Mike
For safari:
Hello!
The flip does not work on android samsung galaxy4 or iphone 6
so- I would like to fire the animation via a click instead of a hover. I see the explanation you give-and alos see the demo with toggle button-but this does NOT work on my end.
Can you please provide specific instructions for making this work with a click. I would like to click the front of the card to start the flip- then click the back of the card or anywhere else on the page (including another card front) to flip back to the front. I have multiple cards on the page. Would like to use jquery. Thanks
This works great!!
I online want it to flif when there is a onclick, I tried it myself but I cant figgure it out.. Does somybody knows?
Thanks
Hello–guys please help me out! look here: http://zevtennis.com
on the videos part, I have the flip effect – but it only works on the desktop. I cannot get it to work on a touch screen device( tested ipad, iphone 6, samsung galaxy s4)
notice that on these devices the back seems to show through and clicking does not ‘flip’….HELP!
thanks
an example working in ie firefox and chrome here: http://www.seeveeze.com
Looks great Amine! Can you help me with my site. I just cannot get it to work in IE 9 or on touch devices! HELP!!! I’m fine with the flip effect just switching from the front to the back in IE9 and on touch devices, as long as clicking will work. Right now, clicking works on most modern browsers on the dektop, but NOT on IE9 or on modern touch devices. Just cant figure this out…..
Hi Matt
The trick is simple: the CSS must be different whether you use internet explorer or other browsers. You create therefore 2 setups, lets say a DIV called Ieflip and a DIV called Webkitflip and at pageload you delete one of them based on browser detection.
You can see the 2 differents setups if you inspect the document in internet explorer and then in chrome. I will post my code later today
Thanks Amine. One thing thats bothering me–the issue is not only on IE9–it is also on any touch device(ipad2, iphone6, android galaxy s4) these are modern devices with modern browsers(safari, chrome, dolphin,) but the problem is still happening. If you pull up my site(zevtennis.com) on a dekstop everything works fine with the flips–but if you pull it up on a phone or tablet—BLEEEHHH–not only are the back divs showing behind the front divs, but worse than that-the onclicks are NOT working–so the user cannot flip the front and see the back. The back has the button for the video to play—so functionality is really broke here!!!
any and all help is greatly appreciated.
Note: is seems that David’s demo (http://davidwalsh.name/demo/css-flip.php) is working in touch devices–but not in IE9
I think there is a lot of added stuff in the demo that David did not post as part of the tutorial…..
I am loading data into the front and back divs in the flip-container you made .. Since the data is dynamic there is no telling on what should be the height of the divs … So I tried to set the height of flip-container , front and back as
min-height: 30px;
so that it can grow as much as it wants according to the amount of data …. but it is not working.. all the divs collide when I set the min-height of the flip container … it works only if I set the height of flip-container to 300 or above .. Help me!!Everything works fine in all browsers but less Safari 5.1.7 Windows version, is a bit slowly in effect and has a lot of inconsistency. Someone has some idea how to fix that ?
Behavior: Every time when I move the cursor to image the flip effect has a big delay and many times is very fuzzy when to flip the image.
Hi, first of all thanks for the great flip animation code, and the internet explorer part! I have one question: I have made it full screen and it works perfect, accept for the fact that the front contains just a full page background, but the back side has more content. On small screens i therefore have a large empty space underneath the frontpage background img after scrolling. Any advice? I cant just take out the scrollbar cause mobile users will still accidentily be able to scroll..
Can someone point me to how to use this to flip multiple images vertically in sequence, like for example, a calendar?
Hi Guys,
i love this thing, but i´m having some issues with getting this to work responsive.
I use the http://www.responsivegridsystem.com/, but the footer will also go up behind my four cards. That is – i think – because of the position:absolute; on the .front and .back-Classes.
Got any ideas how to fix this so it will work responsively with flexible footer-height etc.pp.?
Here you can see what i´ve build so far: http://4ffk-media.de/koenige/index.html
Thanks in Advance!
why we are using
transform-style: preserve-3d
? what’s the exact purpose ?Perfect dude! that’s exactly what I was looking for. At first I wanted to use the jQuery plugin Flip but didn’t know I could do it with css. Cheers :)
I cut and copied the same code above .The flip is happening but the image is broken .Why?
Thank you for the nice tutorial. I just managed to put in place and get rid of the hover effect, but implement the click effect on the div box. I just got the problem that it doesn’t work on IE11 – it simply turns the container bottom down..
Here comes my CSS:
Your code works perfectly if there is only one flipper on a page.
The code shows problems on touch browsers only when there are more than one flippers on a page.
For example:
Just visit your own demo page on a touch screen browser. Touch each flipper one by one and you will understand what I mean.
It doesn’t work in Safari for Windows Desktop! Version 5.1.7 on Win 7!
Was just testing it out for different browsers to check compatibility.
Hi,
Thanks for the awesome effect. I have a minor issue showing in Firefox. On the back side, there is 2 paragraphs I am using, When it flips, the space between two paragraph flashing a black color.
Has anyone seen an issue where there’s no transition to the back pane? Sometimes it doesn’t look like it’s turning, the back side instantly shows up on mouseover. When you mouseout, it transitions back to the front. It doesn’t happen all the time, but I hovered and went out many times and it did this about half the time.
Any thoughts?
Yes chad, You are right, also there is some bug showing in mozilla firefox, am using Mozilla FF version 39.
But no idea what’s the issue. You can see the bug report which I’ve made in Bugzilla here..
https://bugzilla.mozilla.org/show_bug.cgi?id=1189340
Hi David,
Can you tell me how I would implement this effect in Webflow?
Thanks
Raj
Hi, David your post has always been helpful to me.
The code block in this post above :
I think can be:
Hope its not a redundant comment.
Cheers!
Firstly: thanks for demonstrating how to do this! taught me heaps.
But… when i add it to a more complex container that has
overflow-hidden
(eg think along the lines of a carousel) it breaks and the front content becomes visible. This might be the overflow issue you mentioned in the article? I tried removing the absolute positioning of the front/back content but then the back content is in a weird position and the effect doesn’t work. Any ideas how to get around this?Cheers for the article. I used this code as a basis for a flipping website with hidden content behind it.
http://codepen.io/thepixelninja/pen/KdKdRb
Hi,
Is it possible to do a flip in javascript. I am using google map api v3. So In my code when user clicks an overlay a new image replaces the old image. Is it possible when the user clicks the overlay the old image flips to produce a new image. Here is a piece of my code.
Thanks for the thorough demo! Much appreciated and the IE10 updates were most useful.
how can i flip the card and not flip back please
I have it working and its amazing, but when I want to do it with more then one image and get them side by side in a div. Anyone got any ideas on how this could be done? Naturally they fall underneath one and other. I have tried float:left; and making the container big enough to hold them all; both have had 0 effect.
Is there a proper solution for using this with a flexible 100% height and width?
ontouchstart="this.classList.toggle('hover');
Won’t work correctly on iOs devices. Tap the flipped image will not flip back. What did I miss?You have to swipe down on it to toggle back and forth. Tapping once flips it, tap twice goes to link, if you did that.
here you go. remove all
ontouchstart="this.classList.toggle('hover');
and add below JS :I have tried all the fixes for IE10+ here but the text on the back is reversed. Has anyone found a fix for this yet?
I have the sam eproblem today on April 22, 2016. I can’t see when your post is dated.
Thank you SOOOOOOO MUCH! (when you’re at the end of the workday and just don’t want to bother to do it yourself … this is magic)
Not work in ie11
It did not work for me in IE11. Only the front is displayed, overlaying the back. It shows the back upon load for a split second. I used the live demo code to get the vertical flip to work. Is there a chunk of code we’re forgetting to place in the style for it to work in IE 11? Someone said the demo works in IE11.
Slightly correction for the CSS Vertical Flip:
Should be:
Hey David Walsh! greetings from Argentina! I was wondering if you could share a video on YouTube showing how to implement the flip card about students I have . Obviously would leave the link to your website and in the video you would appoint as the mastermind .
Thank you for this tutorial! however there is a typo:
Should be
1000px;
http://codepen.io/w3core/pen/XmNWxd
how would I make it so the div flips and flips back but in the same direction rather than flipping back?
Thanks! I’m toying around with this a bit. IE 11 has a delay in the display of the “back”. I hate IE. Thanks for the tutorial.
Even with the IE edit, I am testing it on IE11 and it doesn’t work. Everything is good except when it flips over, the text on the backside is viewed backwards (flipped horizontally) instead of reading and appearing as normal text. Not sure how to fix this.
Thanks a lot for your contribution. Would you know how to add any of your flip CSS code to a image in Squarespace? Is it possible?
Thanks !
I wanted to use several flip cards on the same page and assumed just changing the class name flip-container to flip-container2, flipcontainer3 etc and referecning that would work but it went nuts . How did you get your several examples on one page?
Could you please tell why
.flip-container:hover .flipper
is repeated twice ?One is a
.hover
class in case you want to programmatically make it flip.Hi there!
The animations work pretty fine in chrome but the problem is that the alignment of my elements are pretty much messed up.
Somone one help me out please.
Is there any way to make it work on Android tablet ?
I have been building this.. Not sure how it holds up anywhere outside of chrome yet. But this might give some of you other ideas as to what is out there.
http://codepen.io/justcald/pen/EyaBxG
disclaimer.. None of the effects have prefix’s on them yet.
Great idea, I have played around with your code a lot and determined rather than predefining the height and width of the flip card element, only put
position:absolute;
on the back side of it so that the front content determines the size, that way you just have to make sure the front and back are the same.This way it can be re-used at different sizes without having to write an entirely new set of rules for each size.
I made a puzzle-like image using this flip css for cards, but I seem to have a problem..it all works perfectly in Chrome, but when I open my html in Safari the flips works but some of my front-images of the cards aren’t showing..
What is causing this issue??
I’m attempting to get this working on a website where the images have a responsive width, and no set height. However, this is causing the back side to be very small. Are there any fixes you can think of to make the div height of the back match the height of the image without directly specifying it?
Hey David! Great stuff, I used it for a simple portfolio page I’m developing. However, i’m finding it doesn’t work on iOS (both front and back of ‘cards’ appear on top of each other). It works just fine in Android. Any suggestions of what I can check out to try and fix this?
Hosted here: http://danwulff.github.io/portfolio/
Source code here: https://github.com/danwulff/portfolio (note that the .js file is inserting html via jquery for the individual cards)
Ope, figured it out. Two things:
1)
-webkit-
prefixes are still required for iOS transform CSS code2) adding
onclick=""
to the html tag of the card div causes iOS to recognize a touch as a hoverFeel free to look at the repository linked above for how I implemented that shiz.
My code, and in my opinion great effect to present product on website.
http://codepen.io/winiardesign/pen/XjppKr
how can we allign 6 flip cards in a horizontal
In case anyone doesn’t like the fact that this relies on absolute positioning, I made a version that uses css animations instead and makes it so that absolute positioning is not necessary, thus you can have elements below it if you don’t know what your height of the container will be:
https://codepen.io/anon/pen/bwmkAo
Hi there,
thanks for your work. But one problem I have: the back-side does not react on touch very well, also not in your demo. Why? Only at the very edge and only with some luck it flips back to front.
And is it possible that a card flips back to front when i tap the next card? Does this need js?
Please help. Thanks a lot.
Best, Stephen
we have odd bug. Everything works fine with other browsers but not in Safari. After flip that area flashes front area and after that you can see only back but when clicked then it hovers front’s title and not text from back.
Hi there, great tutorial thanks! I was just wondering if it was possible to get it working for 2 images side by side,i’ve tried putting them in a row or a container but nothing seems to be working, could you help me please? Thanks in advance!
how can i add the javascript and css if i want to flip it on my command. what styles am i going to remove? am i still going to use toggle ?
Hey David, great article! I was wondering if there was a way to get the scrollbar from shaking when you want to scroll down to see more content? Every time i hover over the scrollbar it shakes, I can’t click the down arrow to scroll down. Thanks for your time.
Thanks for the article!
I made it in LESS with IE10 support:
http://codepen.io/grafov/pen/MpBXLx
thank you for your article.
A question:
I use :
document.getElementById('flip-container' + i).classList.toggle('flipper');
to flip cards on mouse click, but i cannot keep my card flipped, when i click another card the previous one flips automatically… there is a way to flip each card obtaining also all card flipped? thank you for your good articles.
Hey Y’all.
I’m trying to use this code to make a “block quote” box for our customer testimonials. Attached is my CSS style for my website regarding the flip animation sequence (line 312-421). What I am trying to do is have the front image be different for every specific customer image. I’m guessing I need to do that in HTML. I’ve toyed with it for awhile and reached a block.
Any info would be wonderful!
I would like to use this approach in a web project I am currently working on. However, I need the dimensions to be flexible. Whenever I try doing that, the flipping effect stops happening.
Very tired tried to get it work in IE11.
All I get is an working example in another browsers.
Author, it’s hard to understand a difference between two solutions.
But in Edge the first solution works.
Good bye, IE!
Great article, works smoothly. Thanks.
I’m having troubles while use the flip in the chrome in android platforms, any idea how to fix it?
I did my own variation on this to integrate with twitter bootstrap by allowing auto width. I also wanted to solve for variable heights by trading position: absolute for float: left.
https://codepen.io/JoelStransky/pen/ZXOGzV
Newbie here. Was having trouble with the back card showing through on mobile. Added:
-webkit-backface-visibility: hidden;
to the CSS and that fixed it, in case others are experiencing this issue!
Thank you! This was super helpful!
This code don’t work with Safari 11 on me. Any code to add make it work?
Got it working on Desktop, on mobile though it does nothing. When I click nothing happens..
Thank you so much man. This is awesome!
Thank you – this is great!
I wanted a book opening effect, and with a slight change your css can do that too :)
Just add:
And replace 180deg with -180deg.
And voila, the page turns outwards on the left hinge, as if opening a book.
Got it to work in ie11, my problem was on rotation it flickered to the back by delaying on the front for about half a second, I’ve fixed my version of ie11.3…
I think the important elements are hiding the front when it goes to the back, then the z-index order, front then back but the back hover has the highest setting for force out the front flip flickering.
I need to go back through and remove come css (probably half of it lol) but these are the important elements. I’m sure some peeps with more experience can explain this better.
Good luck
Then on the back hover I gave that a z-index to force it to the top
Hello! I used the codes you showed here, but for some reason all it does for me is flip my image in a flipcard animation. How can I put my own back side? (Just gonna change the background and add text)
Hi! I have used this guide to create a flip animation. But what if I wanted to have the entire card to be as high as the highest of the front and back sides. Could this be done without using any Javascript?
Sorry but what have you changed to make it work in touchscreen? in my work it doesn’t work.
could you tell me what you did specifically?
Has anyone managed to make it work with the touchscreen? I tried but I see that even the demo doesn’t work here … am I wasting my time or is there hope?
Thanks
Hi David,
I started using this, and needed within a flexbox context (many images thumbnails with file details on back). I changed the event, from hovering to just clicking, I find it a little bit more user friendly (otherwise cards are flipping all the time), so I made this codepen for sharing with the community. Saludos desde Argentina
https://codepen.io/daniel-faure/pen/rNMxOPj
Hi David, I used this for flipping cards in a flex context. PLease see it at the codepen
Saludos, Daniel
https://codepen.io/daniel-faure/pen/rNMxOPj