Say Goodbye to Vendor Prefixes
Imagine you're lying on a beach. Waves slide up and down a sandy shore while the warm sun beats down on your skin. You sip a cool, refreshing drink, and sigh as gulls faintly caw in the distance.
A gentle breeze lightly brushes your fingers as they slide across your keyboard. Tap tap tap. You're writing CSS. Not just any CSS, but pure CSS, the purest you can imagine. There are no vendor prefixes or browser inconsistencies, no external libraries and no compilers. Your code just works.
In this article, I'll show you how this dream can become reality with a tool called Autoprefixer. I'll walk you through the problems it solves and how to set it up.
This article is the second part in my series on flexbox. If you like it, be sure to subscribe to the entire course over at Free Flexbox Starter Course.
Writing Vanilla Flexbox Sucks
With flexbox, there are two things getting in the way of coding utopia: old versions of the syntax and vendor prefixes.
Old Versions of Flexbox
The process for building a new feature like flexbox into HTML and CSS is complex and lengthy. It may seem like the flexbox is fairly new, but the first draft was actually done back in 2009. Since then, flexbox has gone through two major changes, leaving us with three versions of the syntax.
- The 2009 version used
display: box
and had properties that began withbox
. - The 2012 syntax used
display: flexbox
. - The current version uses
display: flex
and properties that begin withflex
. It's extremely unlikely the syntax will change again.
These implementations are very similar, but the syntax is different and the older versions don't support all of the newer features. Unfortunately, Android 4.1 and 4.3 only support the 2009 syntax, and IE10 only supports the 2012 syntax, so if you want maximum browser support you still need to use them.
Vendor Prefixes
Have you ever seen CSS properties starting with -webkit-
, -moz-
, -ms-
or -o-
? Those thing are called vendor prefixes. My favorite explanation of vendor prefixes comes from Peter-Paul Koch of QuirksMode:
Originally, the point of vendor prefixes was to allow browser makers to start supporting experimental CSS declarations.
Let's say a W3C working group is discussing a grid declaration (which, incidentally, wouldn't be such a bad idea). Let's furthermore say that some people create a draft specification, but others disagree with some of the details. As we know, this process may take ages.
Let's furthermore say that Microsoft as an experiment decides to implement the proposed grid. At this point in time, Microsoft cannot be certain that the specification will not change. Therefore, instead of adding grid to its CSS, it adds -ms-grid.
The vendor prefix kind of says "this is the Microsoft interpretation of an ongoing proposal." Thus, if the final definition of grid is different, Microsoft can add a new CSS property grid without breaking pages that depend on -ms-grid.
Putting Them Together
Let's take an example of some flexbox code from the previous lesson:
.fourth-face { display: flex; justify-content: space-between; } .fourth-face .column { display: flex; flex-direction: column; justify-content: space-between; }
When you add in vendor prefixes and old versions of the syntax to support older browsers, it looks like this:
.fourth-face { display: -webkit-box; display: -webkit-flex; display: -ms-flexbox; display: flex; -webkit-box-pack: justify; -webkit-justify-content: space-between; -ms-flex-pack: justify; justify-content: space-between; } .fourth-face .column { display: -webkit-box; display: -webkit-flex; display: -ms-flexbox; display: flex; -webkit-box-orient: vertical; -webkit-box-direction: normal; -webkit-flex-direction: column; -ms-flex-direction: column; flex-direction: column; -webkit-box-pack: justify; -webkit-justify-content: space-between; -ms-flex-pack: justify; justify-content: space-between; }
Yikes! There are a ton of problems with this code.
- We're repeating ourselves. Generally, developers shouldn't duplicate code whenever possible.
- It's a lot of extra work to remember all of those vendor prefixes. They also don't always match the names of the regular properties.
- It's really easy to forget a vendor prefix. If you do, you probably won't notice the problem unless you're developing in that browser.
- It's more difficult to maintain. When you make a change, you have to do it in several places.
Autoprefixer to the Rescue!
Autoprefixer is a tool that automatically adds vendor prefixes to your CSS. It also translates properties to older versions and even removes an unnecessary prefixes. You can even configure it to target specific browsers. Best of all, it works like magic—once it's turned on, you can forget it's there.
CodePen
The easiest way to try out AutoPrefixer is with CodePen. CodePen is online code editor that lets you jump straight into coding. To try it out, head over to CodePen and click on the "New Pen" button.
Next, click the setting icon next to CSS.
Enable Autoprefixer by clicking on the radio button next to the "Autoprefixer" label. I'd also recommend turning on Normalize.
From here, you can type unprefixed CSS into the CSS area and Autoprefixer will do all the work for you!
CodeKit
CodePen is great for small projects, but most web development is done locally. Setting up a computer for development can get extremely complicated. Fortunately, it's easy with CodeKit.
CodeKit is a program that watches and incorporates a ton of popular tools, such as Autoprefixer, Sass and CoffeeScript, into your project. It's $32, but if you're not quite ready for tools like Gulp, it's worth the money.
Note: Prepros is an alternative to CodeKit for OS X and Windows. The instructions for setting it up should be very similar to the CodeKit instructions.
To get started, open up CodeKit and drag a folder into the main window.
Unfortunately, CodeKit doesn't allow you to run Autoprefixer on plain old CSS files. However, you can convert then to Sass files by renaming the extensions to .scss
. You can still write CSS in these files like you normally would. CodeKit automatically copies all of your compiled CSS files into a css
directory, so I'd recommend keeping your source CSS files in an scss
folder.
Click on the settings button and then under "Languages" click on "Special Language Tools".
On this page, check the box next to "Run Autoprefixer". In order to support all of the browsers that can display flexbox, change the "Autoprefixer Browser String" to this:
last 2 versions, Explorer >= 10, Android >= 4.1, Safari >= 7, iOS >= 7
That's it! Now, every time you make a change to one of your files, CodeKit will automatically compile it using Autoprefixer.
Gulp, Grunt and Other Frameworks
Gulp and Grunt are tools that are make it easy to set up a build system for your projects. With them, you can set up powerful build systems that compile your files, run static analysis on your code and even host local servers.
Configuring these tools is a little too in depth for this lesson. However, if you're curious, I'd highly recommend digging into one of them. The gulp-autoprefixer and grunt-autoprefixer packages both include examples in their readme files. If you'd like to see my personal gulpfile, check out this Gist.
Many web application frameworks support Autoprefixer, including Ruby on Rails, ASP.NET, Express and CakePHP. Chances are good there's a way to incorporate Autoprefixer into your favorite framework.
What If You Can't Use Autoprefixer?
If you can't use Autoprefixer in your project, you have a couple options.
The first is to use a library called -prefix-free. This library does the same thing as Autoprefixer, but in the browser. The downside is that it takes extra time and processing power to prefix the CSS, which can make your site feel a little slow, especially on mobile browsers.
The other option is a tool called Pleeease. Pleeease lets you paste in CSS. It then uses Autoprefixer to print out prefixed styles you can copy into your stylesheets.
Wrapping Up
That's it! You've learned how to set up your environment for full CSS awesomeness using tools like including CodePen, CodeKit, Gulp and Grunt. Have a question?
In the next lesson, we'll be taking a look at building 12-column layouts with flexbox. To make sure you get it, sign up for the Free Flexbox Starter Course.
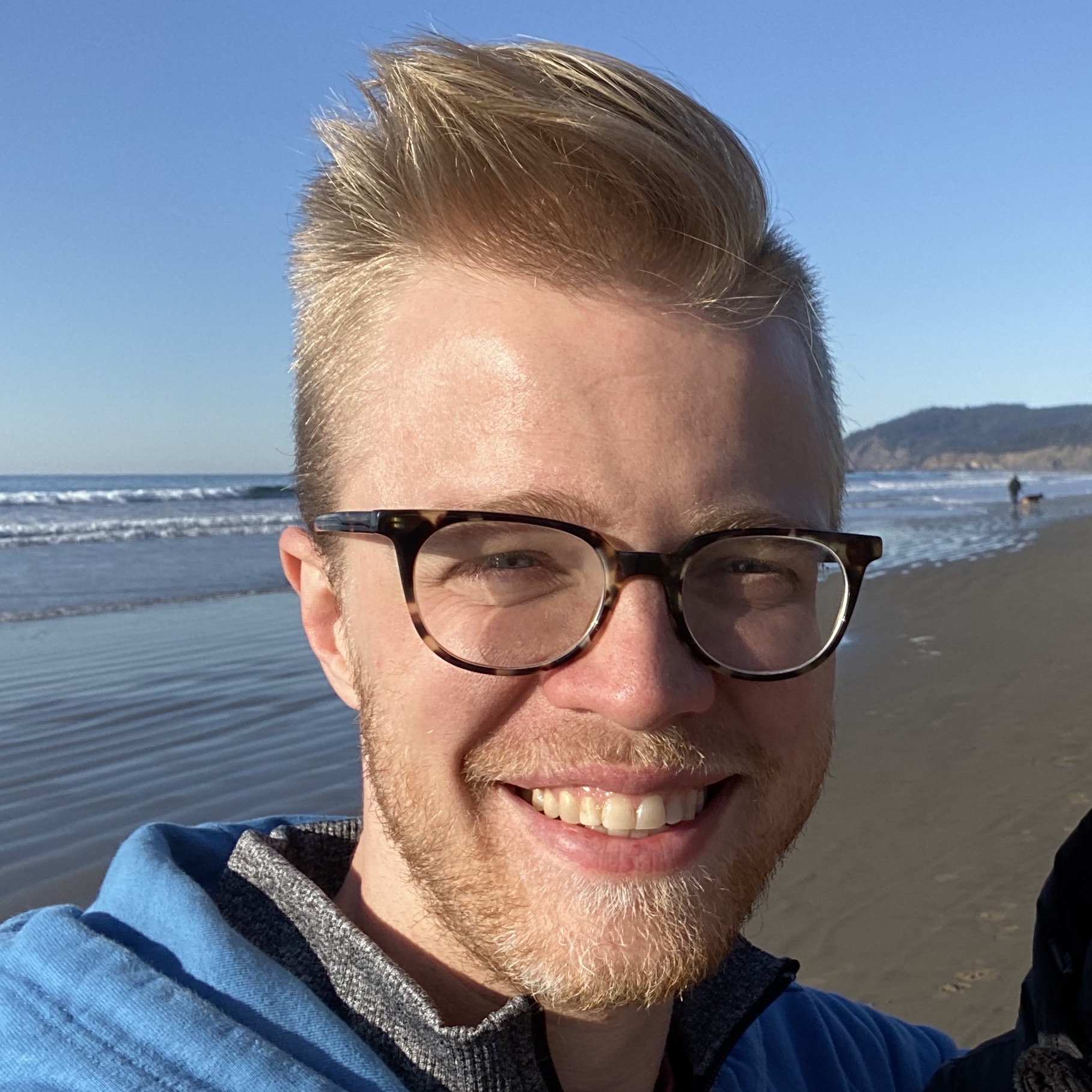
About Landon Schropp
Landon is a developer, designer and entrepreneur based in Kansas City. He's the author of the Unraveling Flexbox. He's passionate about building simple apps people love to use.
we’re using npm with scripts and cssnext to do the trick with auto-prefixing, before we’ve used gulpjs
I just recently started using gulp, and I can’t believe I waited so long to check it out. I’ve been using Auto-prefixer for my codepen projects, but I didn’t even think to look for an integration with gulp. Thanks for the awesome information.
Use Autoprefixer in Sublime Text:
http://www.hongkiat.com/blog/css-automatic-vendor-prefix/
https://github.com/sindresorhus/sublime-autoprefixer
Autoprefixer with Atom:
https://github.com/sindresorhus/atom-autoprefixer
Or, just write code which doesn’t rely on vendor prefixes at all.
This is a joke, right?
Without vendor prefixes, we either have to implement designs with images and hacks like we did back in the Good Ol’ Days™, or not implement parts of the design at all. The latter is not an option in a professional setting.
That said, I don’t believe vendor prefixes are going away any time soon. The CSS spec is still in a state of flux — perhaps more so than previously — and with time I’m confident yet more vendor prefixes will be introduced.
Autoprefixer is a good tool to alleviate the vendor prefix pain, as long as it is kept up to date. Whether you choose to rely on Autoprefixer or on your own mixins is your call. I recommend *not* using -prefix-free; there’s no reason to slow down page render time just to automatically write some extra style rules.
Honestly – no, I wasn’t joking.
I am fully aware of the fact that some things just can’t be realized without vendor-prefixes. But I do keep their usage to an absolute minimum and try to avoid them whenever possible – which works for me in mostly all cases.
You can do a lot more than just vendor prefixes by unsing cssnext
http://cssnext.io/
cssnext is a CSS transpiler that allows you to use the latest CSS syntax today. It transforms new CSS specs into more compatible CSS so you don’t need to wait for browser support.
You can literally write future-proof CSS and forget old preprocessor specific syntax. And this includes Autoprefixer of course.
Try it here http://cssnext.io/playground/
SASS Bourbon also helps to avoid vendor prefixes.
A very nice article. This means one step forward. Thanks a lot!