Geolocation API
Update long overdue -- the Geolocation API is now available in all browsers!
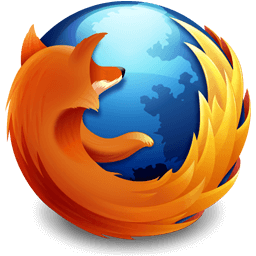
One interesting aspect of web development is geolocation; where is your user viewing your website from? You can base your language locale on that data or show certain products in your store based on the user's location. Let's examine how you can use the geolocation API to get location details!
Detecting Browser Geolocation Capabilities
Feature detection is the best way to confirm the Geolocation API is avilable.
if("geolocation" in navigator) { //w00t! } else { alert("No soup for you! Your browser does not support this feature"); }
They key to detecting Geolocation within your browser is the navigator.geolocation
object. Use in
instead of simply if(navigator.geolocation)
is important because that check may initialize geolocation and take up device resources.
Querying For Geolocation Information
The navigator.geolocation.getCurrentPosition
method is the driving force behind retrieving location details:
if("geolocation" in navigator) { navigator.geolocation.getCurrentPosition(function(position) { console.log(position); }); }
Once you call this method (providing it a function which will execute if your request is successful), the browser will ask the user if they will allow you to retrieve their location information:
When the user allows the website to retrieve their location information, the browser fetches the information, providing you a position object with a payload that looks like:
// "Position" object { coords: { "Coordinates" object accuracy: 65, altitude: 294.4074401855469, altitudeAccuracy: 10, heading: -1, latitude: 43.01256284360166, longitude: -89.44531987692744, speed: -1 }, timestamp: 1429722992094269 }
If you want more information like country, city, and so on, you can use a third party service -- there are many out there.
This API is the foundation of many mobile apps and really should be in the toolbox of any web developer. Best yet is that all browsers now support the Geolocation API. Happy coding!
It will be cool when this becomes a viable option on sites (when more browsers support it)
@Dan: I’m going to write a book about the history of the WWW and that’s going to be the title.
Latitude and Longitude work fine on Opera 10.60.
Kinda scary how easy it is to get your location…
Wow, this creeps me out David! You always seem to amaze me… :-O
A feature like this could be very handy for all those annoyed people who don’t like filling in address forms. Does this always work, even with firewalls/DNS/hotspots/ripping internet from your neighbours?
Hi…I implemented W3C Geo location within MooGeo and you can view in action some demos: http://thinkphp.ro/apps/js-hacks/MooGeo/
Hi…I implemented W3C Geo location within MooGeo and you can view in action some demos: http://is.gd/d8Kgm
Lat and Long seems to work in Chrome 6 for me.
It’s really interesting and cool but it depends a lot on your ISP, for example, I live in Vicenza, Italy, our company uses Fastweb as ISP, and geolocation responds that I stay in Modena, a city in an other region!!!!
This worked great on android’s webkit browser. Also tested in FF and Chrome and worked nice.
Can you update this to use
"geolocation" in navigator
?Here is why: https://github.com/Modernizr/Modernizr/blob/633a5ac/modernizr.js#L478-490
Updated as requested!
Not working in Firefox 23.0.1
Change the last demo part source code
if(navigator.geolocation) {
change toif( "geolocation" in navigator.geolocation) {
Wow quite an impressive feature. @Mohan i faced same issue as Gary and i tried your suggested solution but it did not work.
The browser permission dialogue box can be very annoying to users, especially when I appears on load, before the user can see the rest of the website. It’s particularly annoying on mobile, where the permission dialogue box takes over the screen.
However this blog post outlines a good solution that involves asking the user to initiate geolocation, if they desire, from a link on the web page:
http://nathansh.com/2015/07/13/creepy-to-helpful
Is there a way with pure JavaScript, to show a loading element while geolocation is being retrieved?