Automatically Generate a Photo Gallery from a Directory of Images: Updated
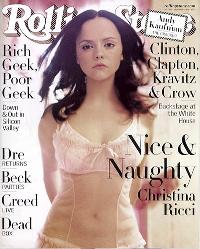
Two years ago Chris Coyier wrote an outstanding tutorial detailing how you can generate a photo gallery based on the images within two directories: a thumbnails directory and an originals directory. I've decided to take his tutorial a step further by showing you how to generate thumbnails for the gallery using PHP. I've also implemented a MooTools lightbox: Smoothbox. The following code will show you how to create a beautiful photo gallery by simply dumping your photos in a directory.
The CSS
.clear { clear:both; } .photo-link { padding:5px; margin:5px; border:1px solid #ccc; display:block; width:200px; float:left; } .photo-link:hover { border-color:#999; }
The images/links will be floated next to each other. The other option would be to use a table. Booooo.
The PHP: Utility Functions
/* function: generates thumbnail */ function make_thumb($src,$dest,$desired_width) { /* read the source image */ $source_image = imagecreatefromjpeg($src); $width = imagesx($source_image); $height = imagesy($source_image); /* find the "desired height" of this thumbnail, relative to the desired width */ $desired_height = floor($height*($desired_width/$width)); /* create a new, "virtual" image */ $virtual_image = imagecreatetruecolor($desired_width,$desired_height); /* copy source image at a resized size */ imagecopyresized($virtual_image,$source_image,0,0,0,0,$desired_width,$desired_height,$width,$height); /* create the physical thumbnail image to its destination */ imagejpeg($virtual_image,$dest); } /* function: returns files from dir */ function get_files($images_dir,$exts = array('jpg')) { $files = array(); if($handle = opendir($images_dir)) { while(false !== ($file = readdir($handle))) { $extension = strtolower(get_file_extension($file)); if($extension && in_array($extension,$exts)) { $files[] = $file; } } closedir($handle); } return $files; } /* function: returns a file's extension */ function get_file_extension($file_name) { return substr(strrchr($file_name,'.'),1); }
We'll use three utility functions to make the system work: get_files (retrieves all of the files in a given directory), get_file_extension, and make_thumb (generates a thumbnail image from a source image). These are good functions to keep at hand for other purposes too.
The PHP: Setting and HTML Generation
/** settings **/ $images_dir = 'preload-images/'; $thumbs_dir = 'preload-images-thumbs/'; $thumbs_width = 200; $images_per_row = 3; /** generate photo gallery **/ $image_files = get_files($images_dir); if(count($image_files)) { $index = 0; foreach($image_files as $index=>$file) { $index++; $thumbnail_image = $thumbs_dir.$file; if(!file_exists($thumbnail_image)) { $extension = get_file_extension($thumbnail_image); if($extension) { make_thumb($images_dir.$file,$thumbnail_image,$thumbs_width); } } echo '<a href="',$images_dir.$file,'" class="photo-link smoothbox" rel="gallery"><img src="',$thumbnail_image,'" /></a>'; if($index % $images_per_row == 0) { echo '<div class="clear"></div>'; } } echo '<div class="clear"></div>'; } else { echo '<p>There are no images in this gallery.</p>'; }
The first step is to define a few simple settings which will dictate image paths, the width by which all thumbnails will be created, and the number of images per row. The action begins with rounding up all of the files. With every image in the gallery, we check to see if a thumbnail exists. If a thumbnail doesn't exist, we use PHP and the utility function above to generate one. When the thumbnail is generated (or there was one there in the first place), we output the HTML link/image. I've given the A element the "smoothbox" CSS class so that Smoothbox will make the larger image display in the lightbox.
The MooTools JavaScript / Smoothbox
All you need to do is include the JavaScript file. Sweet.
That's it! Have any features you'd like to see added? Let me know!
Just a note: it would be wiser to use pathinfo (http://php.net/pathinfo) to get the extension of a file. It would also be nice to use FilterIterator (http://php.net/FilterIterator) along with DirectoryIterator (http://php.net/DirectoryIterator) instead of that boring, inefficient loop in get_files().
Another example with Christina Ricci, I’m starting to think you make this tutorials just to post a photo gallery of her… :)
Why don’t you use
scandir()
?@Mathias Bynens: My solution is PHP4 compliant, as much as people probably don’t care.
Is it possible to set the order of the images?
At this moment it seems to pick the images at random from a particular directory.
You can sort the files by sorting the array of filenames. In the
get_files
function add the linesort($files);
just beforereturn $files;
Very cool! Nice automation of PHP.
nice article. this gave me an idea for a theme that I was working on. I am going to use this. Thanks.
Nice article, yeah! I modified it to work with the jQuery fancybox plugin. What would be a valuable addition is to get it to work with other fromats (PNG, GIF,).
Would you add the code to do that?
Thanks now!
Hi, can You please provide this code (https://davidwalsh.name/generate-photo-gallery) working with fancybox and with png and gif files? Thanks!
Well I had nothing to do tonight so I was able to spend some time to figure out how to add PNG files to a directory and get it to work.
@Mathias – I didn’t know this function. Thanks!
@David – Good tutorial, best regards!
I’ve done something similar myself in the past, but with a few added features. For example, a text file “title.txt” inside the folder which contains an album’s title, and reading the EXIF comment (using PHP) for titles / captions etc of the individual images.
Hi Marc I used this Photogallery for a client wich works great BUT now my client want different Titles on EACH thumbnail picture, and as this thumbnails autogenerate by itself I have NO IDEA how to do this.. and after looking all over internet (I just have no nerves to make a new jquery photogallery all over again!) and found this comment of yours… PLEASE can you tell me HOWWWW I can make this txt file with the Titles?? Im a very beginner in PHP I figured out a lot of things myself by just changing and trying but I never heard about EXIF in my life lol so please if you have a file example or a link something that I can understand to aply it for my client PLEASE I will be more then gratefull!
detail. I like picture 2 a lot :P nice work!
@William Rouse: And how did you do it? I’m pretty interested in PNG and GIF support too…
I want to say it would be simple. There are only two JPG-specific functions; those would change dynamically. Let me play with this some more.
To make it support multiple image types, how about using phpThumb() for the thumbnailing? You could then have all it’s options for thumbnailing, i.e. raidus corners, frames etc. etc….
@Marc: PHPThumb is nice but could be overkill — look how small the current thumbnail-generating function is. Adding PNG and GIF support likely wont add much.
There are a few changes to make to get it to work.
In the index.php file add variable $extension to the call of
make_thumbs()
:In the galleryUtil.php file change the function get_files to this:
And lastly in the make_thumb function except a new parameter for the extension, and set up two if structures:
That’s it.
@Matěj Grabovský: Would you demonstrate how to rewrite the function using the methods you suggested and are available in PHP5
Thanks!
WBR
@David Walsh: While it is nice to keep things compliant… it is better to actually encourage people to move forward, I am pretty sure they are going to be discontinuing support for it soon…
@SeanJA: (It being PHP4…)
@SeanJA: I suppose — I dont’ think it’s that big of a deal.
@William Rouse: Could you not just use imagecreatefromstring instead?
I just read the docs on imagecreatefromstring. Please show me how to use it.
I love all the cool gallery features of your solutions and others I’ve come across. However, they all lack one simple thing for me. Captions.
How could I display captions for your solution?
@Justin: Use PHP’s exif_read_data to read any EXIF comment in the JPG file, and use this as the caption.
I’ve done a few of these in the past few months (including multi-tiered versions with different sub-directories representing different galleries), and have found PHP’s glob() function to be a real timesaver.
Obviously, you’ll want to check that all the files in the directory are indeed images, but you already seem to be doing this with your get_file_extension() function.
Don’t you know glob()? It’s really useful, you could save a lot of lines. :)
@William Rouse: The get_files functions could be rewritten like this: http://gist.github.com/361919
@William Rouse: Sorry, fixed link: http://gist.github.com/361922
I have a image gallery reading from sql database table. table also has thumbnail, bigger_image, qty, color, size fields. I want to display this additional properties alongwith the bigger image when I click the thumbnail.
Please help me. Thanks
@Matěj Grabovský: Thanks for the code.
wow impressive work! I was just thinking how to make a photo gallery with some family pictures and this script will do the job. Thanks!
Thks nice article
can you make a package to download, please.
I agree with Mehdi. This is exactly what I need, but, since I’m a newb, I’m not sure what to do with the three separate code blocks you provide. I would love to have a working package that I could test and edit to fit my needs.
Thanks for sharing your knowledge, David!
could see the GD library used to generate small images … winning hard disk space
I say :P
Hey David,
This is awesome. I was wondering though if we could make this work for a Flickr directory. By this I mean If I uploaded to a specific folder in my Flickr account could i get this to generate the same on my website?
If so, how? I’m not nearly as tech savvy as you so any help would be epic!
David, my question is not very related to this topic, but I could not find a better place to contact you. I was reading the comments of this website:
http://css-tricks.com/redirecting-to-a-splash-page-but-only-once-with-cookies/
and I found your comment there suggesting to use php to set a cookie to show a splash page only once. I’m needing almost the same thing. I want to show an ads banner only once when the first page of the website get loaded.
Do you mind sharing a simple working example as I’m not that expert with php?
Thank you in advance.
PS. I’ll understand if you remove this comment or place it anywhere else.
David, my question is not very related to this topic, but I could not find a better place to contact you. I was reading the comments of this website:
http://css-tricks.com/redirecting-to-a-splash-page-but-only-once-with-cookies/
and I found your comment there suggesting to use php to set a cookie to show a splash page only once. I’m needing almost the same thing. I want to show an ads banner only once when the first page of the website get loaded.
Do you mind sharing a simple working example as I’m not that expert with php?
Thank you in advance.
PS. I’ll understand if you remove this comment or place it anywhere else.
@Vinnie: Posting the same question repeatedly is obviously the way to get help.
@SeanJA: Do you really think it was my intention to post the same question repeatedly? It would be better if you could provide the answer to my question (in case you know the answer) than say what you don’t know.
@Vinnie: It is not really that hard is it?
On the spash page:
$spash = isset($_COOKIE[‘spalsh’])? = false:true;
if($splash) // show spash page and set cookie
else // don’t show splash page and continue to the main page
Is there any chance someone makes a download package with a working gallery? I’m more a designer than a developer but with a working demo and a look at the html/php implementation I think I can make it work.
Thx
Nice Script, I’m curious as to how the script sorts thumbnails? I can’t seem to figure out how it sorts files and how to make it sort by filename. Any help would be appreciated. Thanks.
Very useful script, thanks! I’m also wondering how to sort the thumbnails; preferably alphabetically. Any suggestions?
@Rob and Dez: Ah, here we go… to sort alphabetically, just do this:
After the line
insert this
Hey – I have exactly the same problem as Mehdi and Rudy Vail – the code blocks are confusing, and I have a feeling of that something is missing, do I need the code from the previous version? I can’t make it work (a folder with big pictures, which the code will generate thumbs from and make a gallery, without having to make the thumbs-files myself)
One package with examples would be over-the-top! Nice sharing though :)
/Frido
Is there a way to limit the amout of thumbs coming in?
What if you had 30 images, and you only wanted 10 images shown at a time with maybe some sort of button that adds 10 more. Is that possible with this?
thanks,
yes, can we somehow adopt this script to autocreate “pages”. It already has a set number of images per LINE, how about a set number of LINES per “page”?
I could figure out how to hack this if it the “pages” would be straight html, but I’m lacking the experience in PHP and CSS….
using the older version, I want to sort files and, if possible, add captions to the enlarged versions.
So… for sorting files do I use:
and if so, where does that go?
Thanks for any help and advise. (I already have my thumbnails prepared and named.)
Excellent tutorial, thanks.
I made a minor change based on a problem I have sometimes, which is getting a bunch of images, and they are too large. I know there are batch conversion programs out there that reduce image size, but I thought I’d try this one. It was very easy to do.
I added two varialbes in the settings…
and added
Hi David
Always smarts examples !
Is-it possible to extend your example to make it function using urls rather than images in a folder ? (image of a web page given the url)
Hi
For your info I use the well known IEcapt.exe to create images of web pages given an url …
Even if you need to add some threads to avoid the freeze of your program during the creation of picture, it gives good results … results can be seen here at http://www.saepe.ch
Do you use it too ?
PS : thks again to David who is inspiring a lot !
It took me awhile in figuring it out on how to run this whole package.
So I wrote a little summary on what I did. (maybe saving others time)
Here it goes:
First, create a test folder. (gallery maybe)
then make sure you have these files:
gallery.php – see below
mootools.js
smoothbox.js
smoothbox.css
loading.gif
create a subfolder named preload-images and put your pictures there
create an empty subfolder named preload-images-thumbs
Now for the gallery.php
/* copy and paste the css here */
Well, aside from this little trouble I had. It’s a nice piece of work!
Here’s the (missing) gallery.php from above
<html>
<head>
<script type="text/javascript" src="mootools.js"></script>
<script type="text/javascript" src="smoothbox.js"></script>
<link rel="stylesheet" href="smoothbox.css" type="text/css"/>
<style type="text/css">
/* copy and paste the css here */
</style>
</head>
<body>
<?php
// copy and paste all the php codes here
?>
</body>
</html>
Thank You for that simple breakdown. I am new to php/ advanced scripting, and it’s refreshing to have something actually laid out from square one.
You made this work for me and my client!!
Is there an asy way to get this to work with subfolders?
These subfolders would then be the albums (containing various images) in the gallery
Do you have a sample page to look at – the code in place I mean – so i can see where I’m going wrong.
I cant seem to get this to work.
Anyone have a way of create multple galleries from this script?
Found an alternative that supports subfolders
http://sye.dk/sfpg/
Thank you – went and got it – looks promising!
I really like this automated script and improvement on adding auto thumbnails.
The one change I would like it that the longest side of the thumbnail is a fixed size and the shorter length is in appropriate perspective.
That way the thumbnails are in a square box no matter whether vertical or horizontal.
Will look better that fixed with only.
How do I script that?
Also, how would I use FancyBox? Much prefer it’s look over MooTools SmoothBox?
Thanks for help
I ust tried to do the same thing as I hear you saying Ken..
Now i just changed width=”100″ height=”100″ to maxwidth=”100″ maxheight=”100″ in index.php
Now it made the thumbs display with the right proportions, like I wanted.. But now the way the pictures are displayed all together, is messed up..
Teck it out:
http://artscreen.dk/selection/index.php
Help appreciated
Cheers,
Mads
can you provide a copy of your full script?
that way I can see where you made the changes with maxwidth/maxheight
You are almost there and I want to tinker too but not being a programmer it is a lengthy struggle.
I see you got it working with fancybox instead of smoothbox.
Thanks
Ken
last posting was addressed to Mads
I have 2 questions.
1)
I would like to auto display the titel of the pictures underneath the thumbs (imagename.jpg)
(Like a picture in a folder on my HD)
Any hints on how to do this?
2)
When clicking the thumbs i would like the big picture to be displayed Fullscreen.
Anyone knows if this would be possible some how? I tried to “ask” smartbox through google.. But no luck..
Any help ore hints will make me happy ;)
Have a nice weekend.
Cheers,
Mads
hello are u trying to do something like this where you have your thumbnails generated on top and when you click on it a image will show up underneath it instead of popping up on screen in a light box like this: http://tympanus.net/Tutorials/ResponsiveImageGallery/ , but have the image generated from the folder like this tutorial, i’ve been trying to look for something like that if you find it please let me know
I don’t think PHPThumb is overkill. The thumbnails in this script look jagged. The only thing I did was remove the thumbnail generation script and replace the HTML echo statement with this:
echo '';
I couldn’t figure out how to implement PHPThumb but I did find a solution to the thumbnail quality issue.
Change
imagecopyresized($virtual_image,$source_image,0,0,0,0,$desired_width,$desired_height,$width,$height);
toimagecopyresampled($virtual_image,$source_image,0,0,0,0,$desired_width,$desired_height,$width,$height);
Also change
imagejpeg($virtual_image,$dest);
toimagejpeg($virtual_image,$dest,100);
The default is 75% quality so you have to specify 100. Cheers!
Hi,
I would really like to use this script however I would like the images to be squared. ie. the same size.
Is this possible at all?
Thanks,
Jake
You can use the PHP function imagecopyresized to resize images as they are being processed.
Nice work again David, thanks for sharing. On some browsers (Safari for example) horizontal scrollbars appear when the thumbnail is clicked… any idea how to kill these? (It doesn’t seem to be a small-screen problem).
Also, is it easy/possible to create a second gallery on the same page?
Thanks!
Hi,
Thanks for the code David.
I’m trying to edit it so that the thumbs are displayed in one long row.
I also want the page to expand to the right to accommodate the long row of thumbnails.
No matter what I try, the thumbs always start a new row when they reach the edge of the browser.
Any ideas anyone?
Thanks for this script, I really need this kind of script because I’m a freelance photographer.
From the comment above..some of them like the thumbnaild to be crop..not resized..like this http://scriptandstyle.com/AutoGeneratingGallery/ i wonder how they make the thumbnail crop. This sample comes from the same script with some adjustment.
Anybody know how to make thumbnail like this.
Thanks guys,
apprechiate it
Form and function. Love it.
For the record, and I’m sure almost everyone knows this, but you can’t change filenames and expect this thing to work. For non-programming designers out there, don’t go changing mootools-1.2.4.js to anything shorthand. That’s 4 hours of my life I’ll never see again.
David, I notice the lightbox appears to “swoosh” in from the bottom left … anyway of just making this a simple fade-in fade-out? If functionality is already there, how would you tell a designer non-programmer type how to change this?
Great tool and I plan on using it whenever possible. Good work and thank you! BTW … GO BADGERS! Buckeye killers this year.
i am so pissed at this thing, no matter how much i change $thumbs_width, the thumbs remain at 200 pixels! what is causing this?
help!
The thumb that is being showed, is it a .jpg?
Thanks for this excellent script and the clear example, it was just what I needed.
I agree with various people about the nasty jagged thumbnails so I just replaced the thumbnail generation with PHPThumb (https://github.com/masterexploder/PHPThumb) and now I have nicely resized, cropped thumbnails :)
Thanks again!
Would you mind showing how you adapted this to this script?
Thanks
I just can’t get this to work, my file paths all seem to be correct, i guess i am just a moron but i can’t get this to work, i just keep getting there are no files in this gallery, but i can’t see where i am going wrong, can someone give me “the idiots guide” to doing this, i feel it would be so much much better than any other gallery i could use and so much easier once it’s up and running
Is there some place I where I can download all files in one package?
Thanks for the tutorial!! and for some tips by everyone here.. :)
I’ve got everything to work except for the Smoothbox.
am I doing something wrong? I don’t want to say what I’ve done because I’ve done exactly like the tutorial says..
am I missing something? a file? something?
thanks!
When I try to use this script I get an error message
Fatal error: Out of memory (allocated 11272192) (tried to allocate 6400 bytes) in /home/maitland/public_html/gallery/upload.php on line 101
On line 101 is
$source_handle = $function_to_read($images_dir . '/' . $filename);
http://davidwalsh.name/increase-php-memory-limit-ini_set
How do i go about sorting by the latest added?
This will be helpful.
http://www.webdeveloper.com/forum/showthread.php?t=162099
I actually found that while looking online, you must forgive me though as i am not the most experienced of coders! I couldn’t really figure out how to implement that in to the current script
Hi !
Great plugin !
It’s possible for multiple folder ?
Cant seem to make it work.
I keep getting “There are no images in this gallery.”
Ive been frying my brains for over 2 hours…
Followed Nobody’s walkthrough, changed folders thinking maybe this is a relative/absolute path mistake and still nothing.
Could someone please make a package of this?
Pretty please with sugar on top?
Hi David,
a very nice solution. I get it to work when the images are stored in the same folder as the php file that is executed. I am about to include it into an Magento installation and my core images are separated from the file that runs the script. Im passing $this->getSkinUrl(‘images/gallery/collection/’); that holds “http://localhost/magento/skin/frontend/mytheme/default/images/gallery/collection/ ” to the variable $images_dir. The result, “There are no images in this gallery.” Can you help me out here? Give me some hints? I would really appreciate it!
This is really nice! But how do I get it to work when my images are separated from the script. I am trying to implement it with my Magento installation. I am passing $this->getSkinUrl(‘images/gallery/collection/’); that holds “http://localhost/magento/skin/frontend/mytheme/default/images/gallery/collection/” to $images_dir. That gives me the result “There are no images in this gallery.” Can you help me out here? I would really appreciate it!
Could someone please illustrate how i can make those thumbnails square using this?
http://return-true.com/2009/02/making-cropping-thumbnails-square-using-php-gd/
I think this was mentioned in one of the earlier posts!
Thanks!
Mihir.
Has anyone had an issue of the images not appearing in order?
TY for this very good info. :)
I’m trying to use this script and I have the gallery working properly, however it keeps replicating the entire gallery anytime the page is refreshed. What can I do to prevent this?
Did you find an answer to this question? I’m having the same problem.
i got this error
Parse error: syntax error, unexpected ‘;’ in /home/media125/public_html/ingamepic.co.cc/new.php on line 37
What happened to the original Chris Coyier tutorial? The link no longer works :( I’d much rather use that one, unless of course the thumbnails can be automatically generated to be the same size without warping the image.
No wait, never mind! I used this one. Only reason I was attracted to the old auto gallery was because of the beautiful square cropped thumbnails. But I’m loving the auto thumbnails I must say.
Just want to chime in with the rest of them on how to add the square cropping code mentioned above.
Hi,
Great tutorial, thank you.
Have a question though. How hard would it be to modify it to handle subdirectories?
Tried to combine it with another solution but failed unfortunately.
Was also thinking about creating the thumbnails in a similar way using the same dir structure as the images.
Do you have any suggestions? All ideas are appriciated.
Thank you.
Hi there :)
Managed to work out your tutorial and am pretty pleased since I’m very new to PHP… But I was wondering, I would very much like all my thumbnails to be the same width and height, even though some are portrait and some are landscape. How would I edit your code to change the image height to be fixed to take part of the image?
Hope I am making sense! :) Thanks for your help :)
i wanted to upload photos and display the images in the album html code.Can anyone help me in gettine the simple and easily understandable code?
Fantastic, easy and all I needed. Thank you very mush…Kim, Denmark
Can someone package this up for download. I don’t know enough php to do it from scratch and while the tutorial here is great it doesn’t help someone just trying to play around.
Anyone have something working that they would care to share?
A fair amount of people here want a demo to download. Here’s my complete working version that I incorporated into colorbox, because it’s by far superior to smoothbox. NB. the example pictures may be a little racy for some, but they won’t kill anyone ;) http://www.sendspace.com/file/6vq0zm
Many thanks to David, not only for this, but for a great website generally ♥
Didn’t kill me but was funny that you HAD to use racy images. Thanks for the help though. :)
Hi – Hope someone can help… Whatever I do, I can’t seem to get the thumbnails to auto-generate. All I get are the boxes with ? (missing image) icons. When clicked, these link correctly to the larger images. Have checked that I do have PHP5 with GD2. Am using mootools-1.2.5-core-nc.js with smoothbox. I also tried Will Knot B Revealed’s demo, with exactly the same result – missing thumbs linking to correct images. Linux server.
Am wondering if this is a permissions problem – has anyone else had the same issue?
Thanks in advance….
Have just answered my own question… Yes.
Changed the permissions on the thumbnail folder and they are now showing up! Not sure how safe 777 is tho, so any comments on that would be most welcome!
Cheers
Miranda – 777 is read/write and execute on all files for owner,group and all
755 is usually best that means your stuff cannot be over written by joe public – you may also need to change who “owns” the files according to the filesystem
if your on some linux system – check the commands chown -R and chmod -R
I am creating a repo of @Will Knot B. Revealed Snr.’s colorbox version to add new features etc. I am new to php so this will actually be a nice project for me. I am wanting to add subfolder abilities so that there are multiple albums etc. https://github.com/ferman/php-auto-gallery
This is exactly what I needed.. you can see how I implemented it here. http://www.superfavicon.com Now updating my site is easy.. all I do is drop the files in the folder.. I also added a little script that strips the extension from the filename so I can use it as alt text for the image. I also added a script that auto counts the files in the directory and displays that number below the logo.
One question though.. how is it deciding the order of the files when displaying. On my favicon site above I can’t get a grasp on how its deciding to output the images. I would love ABC order.
I’m also having trouble figuring out how the order is layed out. It’s not ABC, modification date/time, filesize. Any help on that?
Hi,
Awesome demo, however do you have the complete file available to download – for a php numpty like me?
Cheers :-)
Has anyone figured out, or looked into how to paginate the results? I am bring up hundreds of images and trying to separate the results into pages or dynamically using something like jquery.
Also whilst on this, is there a way to allow a front end user to search image file names?
Ok I have found some search script that works great… except it is for exact results only, but I need to modify it to search for anything containing:
Me again!!
So i got it to search for just containing using strstr:
But it is case sensitive, which I really dont want!!!
haha
stristr idiot
Ok but still wondering about pagination! :)
Useful starting point, but I think you’re missing an imagedestroy…
Hi
I like the skript but I have ongoing new pictures in my directory (I am a photographer) and I want to have the thumbnails updated every time after I have a new picture uploaded (or simply do a update every 10 sec , for example)
How to do this ?
Hannes
First at all thank you for this simple and useful script.
I took some time to modify it a bit adding the square crop function, a little piece of code to generate captions from the file names and some basic style to the gallery (this time using the old free version of Fancybox). If you want to take a view I’ve uploaded everything in a Zip file, here:
https://dl.dropbox.com/u/11390458/PHP-Automatic-Gallery.zip
It still needs some kind of function to regenerate the thumbnails if the settings were modified.
Cheers!
File updated with Ajax pagination ;-) (same link as before)
can i get that file i need your version to this photogallery for the crop thumbnails
I have added this to a page as it is exactly what I was looking for, but every time the page refreshes it recreates more thumbnails of the thumbnail images it created in the same directory and displays them too. I end up with hundreds of repeated images because it is echoing out the thumbnails as well as the original images.
I do have real, original thumbnails that I have uploaded in a sub directory called “/imageThumbs/” but it won’t read them.
Do the thumbs need to have some sort of addition to the filename to be recognised? also, why won’t it create its thumbnails in the “/imageThumbs/” sub directory?
I added this to find the folders
$albumRef = $_GET[‘LinkRef’];
include(‘ConnectToDb.php’);
$query = “SELECT * FROM albumData WHERE albumRef = ‘”. $albumRef.”‘”;
$result = mysql_query($query) or die(mysql_error());
$row = mysql_fetch_array($result);
$albumPath = $row[‘albumPath’];
/** settings **/
$images_dir = $albumPath;
$thumbs_dir = $albumPath . “imageThumbs”;
$thumbs_width = 200;
$images_per_row = 4;
I’d written
$thumbs_dir = $albumPath . “imageThumbs”;
instead of
$thumbs_dir = $albumPath . “imageThumbs/”;
with the forward slash – now works a treat! Thank you so much.
I want a gallery with multiple folders as albums, just like the one in facebook,
That is i have an admin panel and i upload images different albums i.e folders, and i create new albums also.
So how to implement this.
Can u please help.
Thanks in advence
this one is working for me: http://codecanyon.net/item/auto-grid-responsive-gallery/3864088
How do I add this to a WordPress page/post?
Is there a way to specify an image dir that is up a level?
Any idea how to get a title of the filename without an extension? TIA!
is there any way to limit the height rather than the width?
I have tried to change thumbs size but it remain at 200 pixels!
Is there a way to “hard crop” the thumbnails so they are all the same height and width?
Just saw Alex Dukal’s addition in the comments. That is excellent. Works perfectly. Thanks a ton both David and Alex.
Alex, you should do your own blog post on that, it’s good work!
Awesome… I am looking for this for the past 2 days.. Thanks a Lot
4 years old, and still one of the best demo/howto for a php gallery. Although i would love to see a way to avoid filenames as ALT tag : someone told me we could create an images.ini in image folder that set the ALT tag to whatever you put in it (mynicepicture.jpg = “My Nice Picture”) then retrieve the output in the main gallery.php, but I have no idea how to implement this. Any idea or suggestion ? An example maybe ?
Great article. Is there any way to add titles to the images? And can it pull tiff files?
Thanks!
Doesn’t work with mootools-core-1.5.0-full-nocompat-yc.js
Works with: mootools-1.2.4.js
I can’t see the dates when posts were made here, but I found this script very useful in July 2014. I got the same error message that Dilip Choudhary got because at first I had set the image directory outside of the folder where the gallery script was. When I moved the images to the default image folder in the SAME directory at the gallery script, it worked fine.
Hi,
If anyone has an issue with ordering of images then you could make it work as expected by adding PHP
sort
function ininside gallery.php. Hope this helps someone.
Oh thank you, thank you rseni. I have been using this wonderful script since July, but have been going crazy because I could not get the pictures to go in order. The documentation says the pictures should load in the number order you’ve set in the naming, but mine don’t. I couldn’t figure out what order it was – it appeared completely random.
I just stopped by here to see if I could get the email address of anyone who helped put this script together, but it appears you have answered my question. I put the sort line in the function in the script as you described, and it cleared up the order problem.
Thanks bunches for taking the time to update everyone. I owe you one.
Regards
CJ Rhoads
How can you make it sort by date? I need my Gallery to display the thumbnails with the newest files first.
if you prefer, like me, automaticaly create folder in the same time the script creates thumbs, here is the adding :
Hey :) Love the idea! How exactly can I connect/implement my DropBox? the link keeps sending me back here :/
Hugs from Norway
– Cathrine
Hi,
Can add the ability to clear all thumbnails inside the thumbs folder after a user defined time interval say 12 or 24 hours.
thanks
rseni
Hi there,
First, great add on congrats. This can could be used Dropbox picture folders. Do you plan somethig like that in future?
Thanks.
Jon from http://Dropbox18gb.com
Thank you to everyone who contributed to this page. I am not good at programming – but I am good at copying and pasting and when I see the code for myself, I can typically understand and then make it fit my needs. This project is awesome and I was able to implement it without any trouble – and even customize it for my needs. Thanks again! You people are awesome!
Fatal error: Call to undefined function
get_files()
in D:\website\running\photo\photo_v8\admin\auto.php on line 8It would be very easy by using php glod function
you can add as many extensions as u want..
Cool! Thanks. :)
Can this be modified to also view .pdf files?