Get Bitcoin Value with curl or Node.js
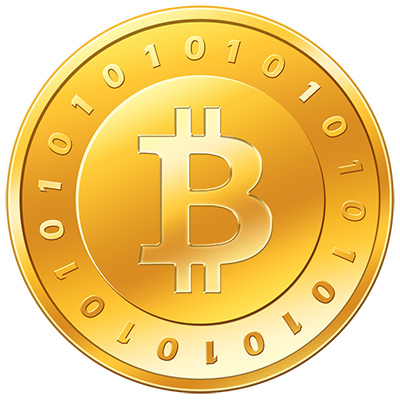
Last year I started dabbling in bitcoin. Of course I was immediately cocky as bitcoin value went up 20% as soon as I bought it, then was humbled as bitcoin's value tumbled down 50%. From boathouse to outhouse. From caviar to ramen noodles. It was brutal.
Anyways, I was often checking the price of bitcoin because it would move up and down quite quickly. I was going to Coinbase to check but as a developer I prefer to do something nerdy to get the value. I've taken a few moments to get the value of bitcoin in a few programmatic ways.
Get Bitcoin Value via Shell + curl
If I want to be low-level "nerd alert" mode, I'll use this command:
curl -s http://api.coindesk.com/v1/bpi/currentprice.json | python -c "import json, sys; print(json.load(sys.stdin)['bpi']['USD']['rate'])"
That command will provide the USD
value of a single bitcoin. You can use GBP
or EUR
if you prefer those currencies. Services other than CoinDesk's main feed may provide another currency value.
Get Bitcoin Value via Node.js
The lowest level server-side JavaScript would look like this:
var http = require('http'); http.get({ host: 'api.coindesk.com', path: '/v1/bpi/currentprice.json' }, function(response) { // Continuously update stream with data var body = ''; response.on('data', function(d) { body += d; }); response.on('end', function() { // Data reception is done, do whatever with it! var parsed = JSON.parse(body); console.log(parsed.bpi.USD.rate); }); } );
As you probably know, this post is less about the code and more about working with the CoinDesk API endpoint. CoinDesk does provide other endpoints to get historical bitcoin data, but I'm more concerned about my money now.
You may want to be compatible with Python 3 there (add () for print, and it is still compatible with Python 2):
No wonder you’re worried about your money. I’ve always had the hunch that cryptocurrencies are nothing more than a fad.
That’s why I’ve never joined the party, even though I may be wrong.
I own 10 bitcoin at an average of ~$340, so I didn’t bet the farm on it, but I am down a decent amount. Just an experiment. :)
This python thing is awful, please try
jq
(http://stedolan.github.io/jq/):http is the httpie tool http://httpie.org
Nice! Thanks for the tip, soyuka!
Use
curl -s
for curl silent mode just to make it cleaner :)Nice suggestion! Updated!
Tada! Groovy one-liner:
or from the command line:
I preferred to just use awk and tr to trim the output:
and I set it up in ~/.bash_profile to create an alias:
If you have json_pp available, here’s a quick recipe that works and not subject to some issues I had with other examples using python.