Building a 3D MMO Using WebSockets
Hi there! My name is Nick Janssen, creator of Ironbane, a 3D MMO that uses WebGL and WebSockets. With this article I would like to give you a better insight in MMO's and make you less afraid of the complexities involved in building one. From my experience I have found that people consider them very hard, while they are in fact quite easy to make using the web technologies of today.
A MMO? You Can't Do That!
MMO's are cool. Yet they are considered one of the hardest things to make when it comes to developing software. I believe MMO's are mainly intimidating to people because of historical reasons.
In the old days, network programming used to be very hard. Complex socket calls were everywhere, multithreading was necessary and JSON was still unknown. A lot has changed since then with the coming of Node.js, its event loop and easy to use socket libraries.
In addition, writing a 3D game was a challenge on its own. You had to include the right libraries, install dependencies on the client, and write complicated engine calls to do trivial things such as creating a texture. Getting a triangle to show on the screen was already quite an accomplishment.
Creating a Texture with DirectX10
D3DX10_IMAGE_LOAD_INFO loadInfo; ZeroMemory( &loadInfo, sizeof(D3DX10_IMAGE_LOAD_INFO) ); loadInfo.BindFlags = D3D10_BIND_SHADER_RESOURCE; ID3D10Resource *pTexture = NULL; D3DX10CreateTextureFromFile( pDevice, L"crate.gif", &loadInfo, NULL, &pTexture, NULL );
Creating a texture with Three.JS
var texture = THREE.ImageUtils.loadTexture('crate.gif'),
The Beginnings
For our MMO Ironbane I took things one at a time, and it worked out very well. Remember, Rome wasn't built in a day. But with today's technology you can achieve things at a much faster pace than what was ever possible before.
I started from a three.js terrain demo and modified it step by step. Within a few days, I had a plane running around with a texture that looked like the pixelated back of a guy.
The next step was to make the player connect to a centralized server. Using Socket.IO I set up a very simple Node.js backend that responds to player connections, and puts them in a global unitList managed by a service called worldHandler:
io.sockets.on("connection", function (socket) { socket.unit = null; socket.on("connectServer", function (data, reply) { var unit = new IB.Player(data); worldHandler.addUnit(unit); }); });
Telling Players About Other Players Nearby
To let players know which other players are nearby, the server has to know at any time which players can see other players. To do so, every player instance on the server makes use of an otherUnits array. This array is simply filled with instances of other entities which are currently in the vicinity.
When a new player is added to the worldHandler, their otherUnits list gets updated depending on where they are in the world. Later, when they move around, this list is evaluated again, and any changes to this list are sent to the client in the form of addUnit and removeUnit socket events.
Now, I would like to point out that the first letter of MMO stands for Massive. For massive games, every player should not know about every other player because it will melt your server.
Spatial Partioning
To remedy this, you need spatial partioning. In a nutshell, this means that you divide your world into a grid. To visualize it, think of it as the server making use of a Snap To Grid option, to "snap" the position of the players to an imaginary grid. The positions of the players are not altered, rather the server just calculates what the new snapped position of the player would be.
With many players spanning over many different positions, some will have the same "snapped" position. A player then, should only know about all players that are snapped in the same position and all players that are just one cell away from them. You can easily convert between grid and world positions using these functions:
function worldToGridCoordinates(x, y, gridsize) { if ( gridsize % 2 != 0 ) console.error("gridsize not dividable by 2!"); var gridHalf = gridsize / 2; x = Math.floor((x + gridHalf)/gridsize); y = Math.floor((y + gridHalf)/gridsize); return { x: x, y: y }; } function gridToWorldCoordinates(x, y, gridsize) { if ( gridsize % 2 != 0 ) console.error("gridsize not dividable by 2!"); x = (x * gridsize); y = (y * gridsize); return { x: x, y: y }; }
When a new player is created on the server, they automatically add themselves to a multidimensional array of units on the worldHandler, using the grid position. In Ironbane we even use an additional zone index, since most MMO's have multiple areas where players can reside.
worldHandler.world[this.zone][this.cellX][this.cellY].units.push(this);
Updating the List of Players Nearby
Once they are added to the list of units on the server, the next step is calculate which other players are nearby.
// We have two lists // There is a list of units we currently have, and a list that we will have once we recalculate // If an item is in the first list, but no longer in the second list, do removeOtherUnit // If an item is in the first & second list, don't do anything // If an item is only in the last list, do addOtherUnit var firstList = this.otherUnits; var secondList = []; // Check for all players that are nearby and add them to secondList var gridPosition = worldToGridPosition(this.x, this.y, 50); var cx = gridPosition.x; var cy = gridPosition.y; for (var x = cx - 1; x <= cx + 1; x++) { for (var y = cy - 1; y <= cy + 1; y++) { _.each(worldHandler.units[this.zone][x][y], function(unit) { if (unit !== this) { secondList.push(unit); } }, this); } } for (var i = 0; i < firstList.length; i++) { if (secondList.indexOf(firstList[i]) === -1) { // Not found in the second list, so remove it this.removeOtherUnit(firstList[i]); } } for (var i = 0; i < secondList.length; i++) { if (firstList.indexOf(secondList[i]) === -1) { // Not found in the first list, so add it this.addOtherUnit(secondList[i]); } }
Here, addOtherUnit() adds that player to their otherUnits array, and sends a packet to the client informing a new player has entered in their vicinity. This packet will contain the initial position, velocity, name and other metadata which only needs to be sent once. removeOtherUnit() simply removes the player from their array, and tells the client to destroy that player.
var packet = { id: id, position: unit.position, name: unit.name, isGameMaster: true }; this.socket.emit("addUnit", packet);
Sending Packets to the Players
Now, we have the beating heart of an MMO. The final step is to inform the players on a regular basis the positions of the other players in their vicinity. We do this step only twice per second, because we do not want to overload the server.
_.each(this.world, function(zone) { _.each(zone, function(cellX) { _.each(cellX, function(cellY) { _.each(cellY.units, function(unit) { var snapshot = []; _.each(unit.otherUnits, function(otherUnit) { var packet = { id:otherUnit.id, x:otherUnit.x, y:otherUnit.y }; snapshot.push(packet); )); if ( snapshot.length ) { unit.socket.emit("snapshot", snapshot); } )); }); }); });
Conclusion
That's really all there is to building an MMO. The only things left to do now are building the features that are unique to your game, fine-tuning and security.
I hope I have given you fresh insights into MMO programming, and above all courage to start working on them. At Ironbane we are surely looking for collaborators! You can find the full source code of Ironbane straight on GitHub, and you should be able to install it on your machine with ease.
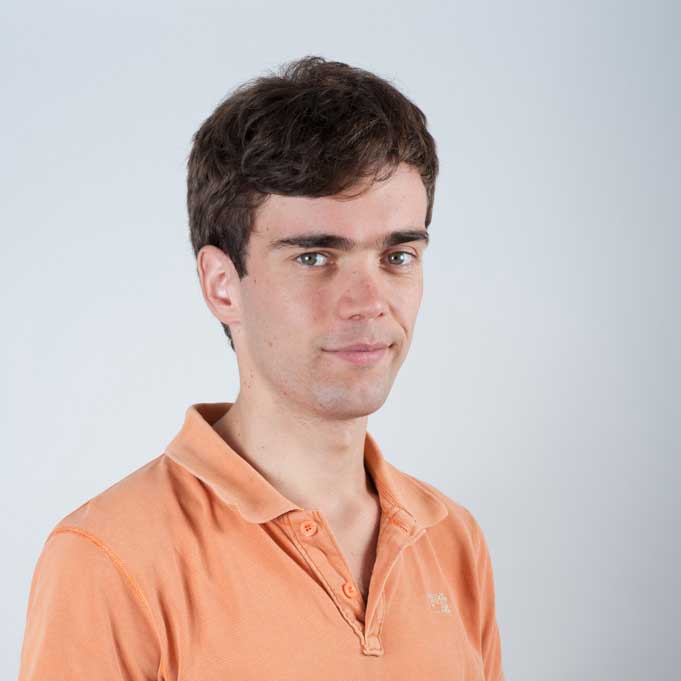
About Nick Janssen
Nick Janssen is a full-stack web developer with a passion for creating cool applications, originally from Belgium.
May I ask you?
I’m looking for a physics stuff for FPS and TPS games.
How does it detect the difference between slippery steep precipices and not slippery gentle slopes?
I was wondering, if you could post another article for it.
Have you thought about building your MMO using WebRTC as an alternative to WebSockets? I could yield much better performance if the clients were to connect to a MCU server through UDP instead of transmitting the data over TCP to your webserver.
kengdie
Good job, Nick!
On a similar topic, I recently wrote this article:
Optimizing Multiplayer 3D Game Synchronization Over the Web
http://blog.lightstreamer.com/2013/10/optimizing-multiplayer-3d-game.html
Some of these topics were discussed at the latest HTML5 Developer Conference in San Francisco:
http://www.youtube.com/watch?v=cSEx3mhsoHg
That’s Awesome Nick!
Let me ask you a couple of questions… :)
1. What happens if a player is on the border? He would be invisible for players bordering his cell, right? And he couldn’t see players on the other border neither.
2. About spawning objects, how would you spawn them? So, having an array list of all the objects in the world and sending them to users around? But that means, that the server would have to check in every snapshot if the user is near objects and that would melt the server, right? Any better approach? :)
3. How would you control NPC’s moves? I’m creating an online game with node js + unity (sockets.io) and everything is running fine but the problem is that Unity doesn’t know anything about the terrain so, I’m kinda lost about how to move npc’s to follow players or try to attack them (pathfinding)
Thank you very much!
Hi Bernat,
My apologies for the late response.
1) What do you mean, on the border? A player will always be within the bounds of a cell, so there would be no such thing as a border where you can be at.
2) You don’t need to check if a player would be near all other objects on the server, since the server already knows its cell position. Therefore, if you add an object, you know its position and also its cell position. You then add it to the right cell of the world. Players only need to know about units in adjacent cells, so there’s really not that much CPU load involved.
3) Please look at steering behaviours, it’s what you are looking for. I recommend to read a book on AI such as http://www.amazon.com/Programming-Example-Wordware-Developers-Library/dp/1556220782 it explains very well about AI, pathfinding and steering behaviours.
It’s easy to build a MMO if you consider a server which syncs position of nearby players 2 times a second a MMO. I don’t mean to be the bad guy or something but building a MMO is hard for the following reasons
1- Maintaining a game with nice gameplay with reasonable bandwidth and writing a server which really scales to a massive number of players without spenindg huge money on it is hard.
2- MMOs need a lot of content like characters, quests, world parts and .. if you keep MMORPGs in mind
3- writing gameplay which is fair considering network delay is not the easiest thing to do in life
4- adding a business model and all other services on top in a way to make money out of it is a hard task.
5- a lot more
One thing, You will not be able to make a realtime MMO game sending data using any string format, JSON included. Please study the subject matter more.
“You will not be able to make a realtime MMO game sending data using any string format”
Care to provide a source for this assertion?
Sending strings is the same as any other byte stream. Sure, if you don’t use it carefully, you can end up with too much overhead, but used properly, how is it different than sending any other buffer type?