MooTools Kwicks Plugin
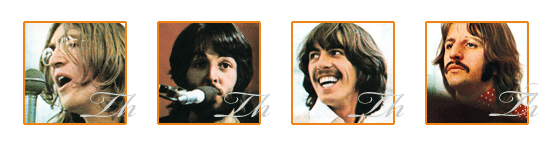
I wrote a post titled Get Slick with MooTools Kwicks ages ago. The post was quite popular and the effect has been used often. Looking back now, the original code doesn't look as clean as it could. I've revised the original Kwicks code so that Kwicks is laid out in the form of a standard MooTools plugin.
The HTML
<div id="kwick"> <ul id="kwicks"> <li><a class="kwick john" href="http://en.wikipedia.org/wiki/John_lennon" title="John Lennon"><span>John Lennon</span></a></li> <li><a class="kwick paul" href="http://en.wikipedia.org/wiki/Paul_mccartney" title="Paul McCartney"><span>Paul McCartney</span></a></li> <li><a class="kwick george" href="http://en.wikipedia.org/wiki/George_harrison" title="George Harrison"><span>George Harrison</span></a></li> <li><a class="kwick ringo" href="http://en.wikipedia.org/wiki/Ringo_starr" title="Ringo Starr"><span>Ringo Starr</span></a></li> </ul> </div>
The Kwicks system works based off of an HTML list containing list items with links.
The CSS
#kwick { width:590px; } #kwicks { height:143px; list-style-type:none; margin:0; padding:0; } #kwick li { float:left; } #kwick .kwick { display:block; cursor:pointer; overflow:hidden; height:143px; width:134px; } #kwick .kwick span { display:none; } #kwick .john { background:url(kwicks/john.gif) no-repeat; } #kwick .paul { background:url(kwicks/paul.gif) no-repeat; } #kwick .george { background:url(kwicks/george.gif) no-repeat; } #kwick .ringo { background:url(kwicks/ringo.gif) no-repeat; }
The most key of the selectors is ".kwick" which features "overflow:hidden". Overflow hidden will allow our squeezing of elements.
The MooTools JavaScript
var Kwicks = new Class({ Implements: [Options], options: { squeezeWidth: 100, maxWidth: 285 }, initialize: function(list,options) { this.setOptions(options); this.list = document.id(list); this.parse(); }, parse: function() { //vars var items = this.list.getElements('a'), fx = new Fx.Elements(items, {wait: false, duration: 250, transition:Fx.Transitions.Cubic.easeOut}), startWidths = [], options = this.options; //kwicks items items.each(function(item,i) { startWidths.push(item.getStyle('width').toInt()); item.addEvent('mouseenter',function(){ var fxSettings = {}; fxSettings[i] = { 'width': [item.getStyle('width').toInt(),options.maxWidth] }; items.each(function(sibling,ii) { if(sibling != item) { var w = sibling.getStyle('width').toInt(); if (w != options.squeezeWidth) { fxSettings[ii] = { 'width': [w,options.squeezeWidth] }; } } },this); fx.start(fxSettings); },this); },this); //list this.list.addEvent('mouseleave',function() { var fxSettings = {}; items.each(function(item,i) { fxSettings[i] = { width: [item.getStyle('width').toInt(), startWidths[i]] }; }); fx.start(fxSettings); }); } }); /* USAGE */ window.addEvent('domready',function() { var kwicks = new Kwicks('kwicks'); });
Unlike my original post, this implementation works off of the MooTools Class system. The class is small and features only one real method but the class' code is also much cleaner than my original implementation!
The Kwicks effect is tasteful, smooth, and attention-grabbing. I recommend adding this to any website that could use a bit of dynamism.
Very good…
Where do you find the time to write all these plugins?
Are you saying I have no life? :) Efficiency comes with practice.
Awesome thanks for retooling it :)
I really like Kwicks but the time it takes to make the images just perfect/right/etc has always deterred me from actually using it.
Good work!
Hmmmm….nice article david!! You are My teacher….
So how would we do this if we didn’t want to use a list?
Lets say, a group of divs, within a div?
Oh man, this is verrrry nice. I’ve been looking for something like this for quite a while and didn’t want to resort to flash.
Also, kudos for using images of the fab four.
Hi, I’m new to mootools.
I need to use mootools version 1.2.1 for a project, so I created a mootools.js with all needed classes. But it doesn’t work.. It only works with your mootools.js, which I downloaded from your demo version. Is there an incompatibility? I tried also 1.2.4 and it doesn’t work, too. I hope you can help me!
Hi mate! Love your Mootools magic :)
However, I’ve discovered a weird bug in this class that seemingly only applies to Google Chrome. Sometimes, the startWidths doesn’t get properly filled, and the menu fold back to 0 pixels when your mouse leaves it. Haven’t been able to find a fix for this, unfortunately, I just hard-coded the values I needed into it.
Hi David,
I’ve been using SmoothScroll with Mootools core 1.3 (excellent plug-in, by the way) but can’t get Kwicks working with anything other than core 1.2.3. As I’d like to use both plug-ins on the same webpage, I was wondering if there’s anything you can suggest to fix it?
Any help would be greatly appreciated, thanks.
Can you provide any detail as to the error?
The browser doesn’t give any errors when I try to use Kwicks with core 1.3; the images used just fail to expand upon mouseover.
I’m probably just failing to implement the plug-in correctly. I managed to get it working using core 1.2.3 by following the example of your demo, and am assuming that to get it working with core 1.3 I just need to substitute one for the other and update which script the page loads.
Out of curiosity, how would you go about changing your Kwicks demo so that it uses core 1.3?
Thanks again, David.
I’ve just been updating a website from Moo 1.2 to 1.3, and with the compatibility layer active everything has gone smoothly, save for an odd bug with the Kwicks. It seems that mouseleave can fail to return the Kwicks to their initial size, if you hover over them too quickly. I’ve verified the problem with Safari and Firefox thus far.
I’ve set up a js.fiddle to illustrate the problem:
http://jsfiddle.net/WHmZX/
Any help on this would be much appreciated.
Fixed, there was a link:’cancel’ missing from the FX.Elements:
http://jsfiddle.net/WHmZX/2/
Fixed the flakey behaviour of mouse.leave in Moo 1.3, there was a link:’cancel’ missing from the FX.Elements:
http://jsfiddle.net/WHmZX/2/
Really nice one and i would appreciate your thoughts.
I be thankful for your thoughtful enter.
Guys,
I want to add social media buttons right after the blog post. How can I do that? Help!
Thank you