Drag & Drop Elements to the Trash with MooTools 1.2
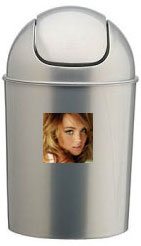
Everyone loves dragging garbage files from their desktop into their trash can. There's a certain amount of irony in doing something on your computer that you also do in real life. It's also a quick way to get rid of things. That's why I've created a trash can class using MooTools 1.2.
The CSS
#trash { width:141px; height:246px; float:left; background:url(trash.jpg) 0 0 no-repeat; } .trashable { background:#eee; padding:20px; font-size:24px; width:50px; height:50px; margin:10px; text-align:center; cursor:move; }
Nothing special. I set the trash can in the background of a DIV and set some default styles for the "trashables" class.
The XHTML
<div id="trash"></div> <div class="trashable"></div> <div class="trashable"></div> <div class="trashable"></div>
Just a trash DIV and the trash items.
The MooTools 1.2 Class
/* class */ var trashCan = new Class({ //implements Implements: [Options,Events], //options options: { trashCan: $('trash'), trashables: $$('.trashable') }, //initialization initialize: function(options) { //set options this.setOptions(options); //prevent def document.ondragstart = function() { return false; }; //drag/drop $$('.trashable').each(function(drag) { new Drag.Move(drag, { droppables: this.options.trashCan, onDrop: function(el,droppable) { if(droppable) { alert('Disposing of ' + el.get('rel') + '!'); drag.dispose(); } }, onEnter: function(el,droppable) { }, onLeave: function(el,droppable) { } }); }.bind(this)); } }); /* usage */ var trash = new trashCan({ trashCan: $('trash'), trashables: $$('.trashable') });
The class is extremely simple to use! All you need to do is provide "trashCan" and "trashables" items.
What Would I Use This For?
Lots of things. Managing the server's file collection from within a browser, for example, would be a great usage. You could also create a bunch of tasks that you delete when you have them completed.
hare your thoughts and ideas for this class!
Ah, nice one David! (And the example cracked me up!) Thanks for sharing, man.
Why you initialize the dragging on start for all elements. If you have 50 or 100 items there is big overhead cause the most of them don’t will be dragged. It would be better to add only one
mousedown
event on the container that holds of all.trashable
elements. When the user starts clicking create a new Drag object withevent.target
asdragable
element(if target.hasClass('trashable'))
.Great example anyway.
Great Work.
Hi there David,
Do you know any way to make the draggable revert to its original position like in a ‘sortable’ list?
Cheers.
So sorry, I forget to wrap in
tag
Hello David:
Me again, this is what I have ’till now: http://jsfiddle.net/escoffie/dAAxY/1/
What’s happening:
1) The sort behavior is not working
2) The el.dispose() is weird lefting behind its children elements
3) The confirm does always the same if I click OK or Cancel
What should happen:
1) The sort behavior should work when dragging the red text
2) The entire LI element and its children should have been removed when dragged to the trash can
3) When asked, Ok should proceed to dispose of the element, and cancel , should revert the element to the UL set.
Thank you in advance, and please delete my previous posts.
Hello David:
Me again, this is what I have ’till now: http://jsfiddle.net/escoffie/dAAxY/1/
What’s happening:
1) The sort behavior is not working
2) The el.dispose() is weird lefting behind its children elements
3) The confirm does always the same if I click OK or Cancel
What should happen:
1) The sort behavior should work when dragging the red text
2) The entire LI element and its children should have been removed when dragged to the trash can
3) When asked, Ok should proceed to dispose of the element, and cancel , should revert the element to the UL set.
Thank you in advance, and please delete my previous posts.
For anyone interested, I finally figured out how to make sortables + trashables elements.
Here is a JSFiddle with the solution: http://jsfiddle.net/escoffie/nD6bL/
It uses Element.collide from Jean-Nicolas Boulay
Any improvements are welcome!