Validate CSP from Command Line
The content security policy spec has been an amazing front-end security tool to help prevent XSS and other types of attacks. I'd go as far to say that every site should implement as specific CSP as possible. If you aren't familiar with CSPs, here's a quick example:
Content-Security-Policy: default-src 'self'; img-src *; media-src media1.com media2.com; script-src userscripts.example.com
If a linked resource or content on the page doesn't pass a given CSP rule, it wont be loaded. Of course getting a massive site to pass one CSP is difficult -- just ask Facebook:
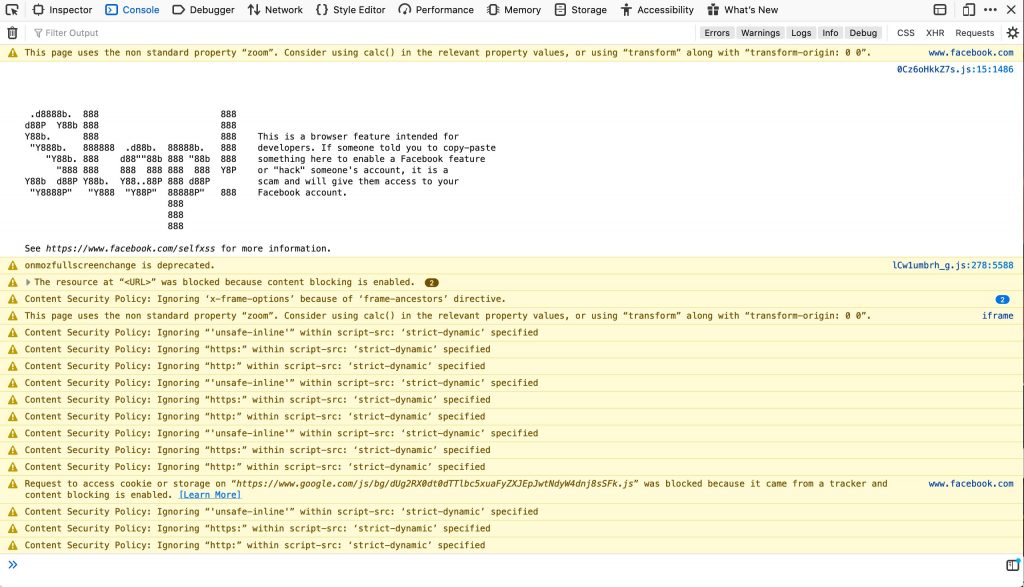
Browsers provide you CSP error and warning information in the web console but that doesn't help developers prevent issues before a push to production. Enter seespee -- a Node.js utility that allows you to validate CSPs from command line!
To get the CSP directives for a given page, you simply run seespee
with a URL:
seespee https://davidwalsh.name/demo/csp-example.php /* Content-Security-Policy: default-src 'self'; frame-ancestors 'self'; frame-src 'none'; img-src 'none'; media-src 'self' *.example.com; object-src 'none'; report-uri https://example.com/violationReportForCSP.php; script-src 'self' 'unsafe-inline' cdnjs.cloudflare.com; style-src 'self' 'unsafe-inline'; */
If you'd like to validate that a given page's CSP passes, which you could do during build or in CI, add the --validate
flag:
seespee https://davidwalsh.name/demo/csp-example.php --validate /* ✘ ERROR: Validation failed: The Content-Security-Policy does not whitelist the following resources: script-src cdnjs.cloudflare.com; https://cdnjs.cloudflare.com/ajax/libs/html5shiv/3.7/html5shiv.js */
If the validation step returns a non-zero status, you know CSP has failed and thus the patch shouldn't be merged.
You can also use seespee from within your Node.js scripts:
var seespee = require('seespee'); seespee('https://davidwalsh.name/demo/csp-example.php').then(function(result) { console.log(result.contentSecurityPolicy); // default-src \'none\'; style-src https://assets-cdn.github.com; ... });
Having a utility like seespee, and not needing to manually check in the browser, is so useful. A solid CSP can be difficult to create but even harder to maintain as the site changes. Use seespee and CI to prevent unwanted CSP and site fails!