Fx.Rotate: Animated Element Rotation with MooTools
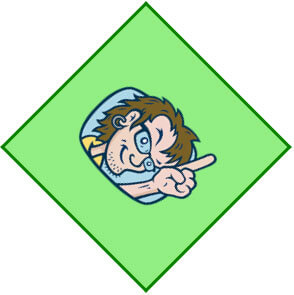
I was recently perusing the MooTools Forge and I saw a neat little plugin that allows for static element rotation: Fx.Rotate. Fx.Rotate is an extension of MooTools' native Fx class and rotates the element via CSS within each A-grade browser it supports. Let's examine how you can easily incorporate animated element rotation within your website.
The MooTools JavaScript
How you use the plugin and when you instantiate the Fx.Rotate class is up to you. For the sake of this being an introduction to the class, we'll keep it simple and make the element rotate when a button is clicked:
window.addEvent('domready',function() { var rotate = new Fx.Rotate('rotate-me',{duration:'long'}); document.id('do-rotate').addEvent('click',function() { rotate.start(0,45); //showtime! }); });
When the DOM is set, we assign a click listener to the button which rotates the element from 0 degrees (its original state) to 45 degrees. You can probably already tell that the start method is the animator. The class is super small because it extends Fx; Fx is where the animation logic is housed.
The MooTools Class
I cannot say that the code, as it's presently available, is up to MooTools' standards:
(function (window,undef){ var deg2radians = Math.PI * 2 / 360 , prefix = ""; function getMatrix(deg){ rad = deg * deg2radians ; costheta = Math.cos(rad); sintheta = Math.sin(rad); a = parseFloat(costheta).toFixed(8); c = parseFloat(-sintheta).toFixed(8); b = parseFloat(sintheta).toFixed(8); d = parseFloat(costheta).toFixed(8); return [a,b,c,d]; } if (Browser.Engine.gecko) prefix = 'moz'; if (Browser.Engine.webkit) prefix = 'webkit'; if (Browser.Engine.presto) prefix = 'o'; Fx.Rotate = new Class({ Extends : Fx , element : null , initialize : function(el,options){ this.element = $(el); this.element.setStyle("-"+prefix+"-transform-origin","center center"); if (Browser.Engine.trident){ this.element.setStyle("filter","progid:DXImageTransform.Microsoft.Matrix(sizingMethod='auto expand')"); } this.parent(options); } , start : function(from,to){ this.parent(from,to); } , set : function (current){ if (Browser.Engine.trident) current *=-1; var matrix = getMatrix(current); if (Browser.Engine.trident){ this.element.filters.item(0).M11 = matrix[0]; this.element.filters.item(0).M12 = matrix[1]; this.element.filters.item(0).M21 = matrix[2]; this.element.filters.item(0).M22 = matrix[3]; }else{ this.element.setStyle("-"+prefix+"-transform","matrix("+matrix[0]+","+matrix[1]+","+matrix[2]+", "+matrix[3]+", 0, 0)"); } } }); })(this);
While this code works (and that's what's most important), I'd prefer to see the class look like this:
Fx.Rotate = new Class({ Extends: Fx, initialize: function(element,options) { this.element = document.id(element); this.prefix = (Browser.Engine.gecko ? 'moz' : (Browser.Engine.webkit ? 'webkit' : 'o')); this.radions = Math.PI * 2 / 360; if(Browser.Engine.trident) { // IE this.element.setStyle('filter','progid:DXImageTransform.Microsoft.Matrix(sizingMethod="auto expand")'); } else { this.element.setStyle('-' + this.prefix + '-transform-origin','center center'); } this.parent(options); }, start: function(from,to) { this.parent(from,to); }, set: function(current) { if (Browser.Engine.trident) current *=-1; var matrix = getMatrix(current); if (Browser.Engine.trident){ this.element.filters.item(0).M11 = matrix[0]; this.element.filters.item(0).M12 = matrix[1]; this.element.filters.item(0).M21 = matrix[2]; this.element.filters.item(0).M22 = matrix[3]; }else{ this.element.setStyle('-' + this.prefix + '-transform','matrix(' + matrix[0] + ',' + matrix[1] + ',' + matrix[2] + ', ' + matrix[3] + ', 0, 0)'); } }, getMatrix: function(deg) { var rad = deg * (this.radions), costheta = Math.cos(rad), sintheta = Math.sin(rad); var a = parseFloat(costheta).toFixed(8), c = parseFloat(-sintheta).toFixed(8), b = parseFloat(sintheta).toFixed(8), d = parseFloat(costheta).toFixed(8); return [a,b,c,d]; } });
Just a bit of reorganization; no real change of logic. Mine is a bit prettier -- at that cost is the re-check of logic for each instance but the processing to do that is so negligible that I'm not concerned about it.
While it's a tad hard to think of an "every day, practical" use of this class, I think that this snippet has its merits and would probably work out great for some purposes of someone's website! Also, take a trip down memory lane and check out Fx.Explode!
http://github.com/arian/Element.Style.Transform
No IE yet?
Doesn’t work in My IE8 :(
Works for me!
Doesn’t work in my IE6 ;)
Um, he spelled “radians” correctly. :-)
…just a minor detail. :)
It’s really fun to see my code used by others :)
I’ll think of implementing it that way, though, if we’re at it, there’s no reason to put the prefix in the initialize:
I usually use public methods only for stuff that should be extended. I don’t see why creating an extra instance of getMatrix makes the code cleaner or better. But, i guess you can never know…
Also, the IE version has a very odd behavior… no idea why
This guy seems to have come up with a fix using jQuery for the “drifting” rotation in IE, but I can’t get my head around producing a mooTools equivalent.
Take a look at the source code of http://jsbin.com/adiwa3/53
He appears to have based his work on the cssSandpaper library:
http://www.useragentman.com/blog/2010/03/09/cross-browser-css-transforms-even-in-ie/
Implemented the fixes. Should have JSlinted before submitting to noticed all the implied global decelerations on the original code (taken from MSDN…)…
All fixed now.
I reckon putting the getMatrix as a method makes since as it will probably be a good place to fix the IE travel problem
It doesn’t work in Google Chrome 9.0.597.98
Peter. I gave up on this library, it’s flawed. I started using jQueryRotate which is a very good implementation of element rotation.
here is the same thing only on jquery.
sorry for my English, I use google translator.
And jquery sign a couple of days, so do not scold severely.
Greetings from Russia:)