Implement the Google AJAX Search API
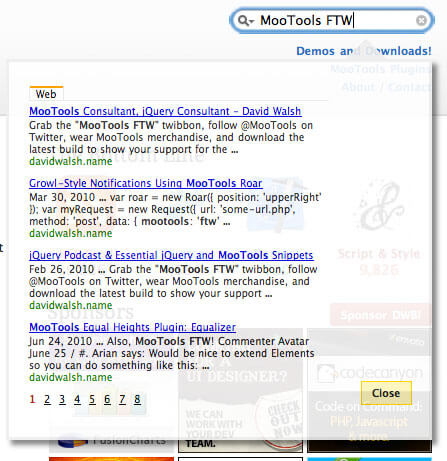
Let's be honest...WordPress' search functionality isn't great. Let's be more honest...no search functionality is better than Google's. Luckily for us, Google provides an awesome method by which we can use their search for our own site: the Google AJAX Search API. Let me show you how to implement this awesome API within your own website!
Sign Up!
Google's AJAX Search API requires that you sign up for an API key. Signing up is free and you'll be done with the process of retrieving a key in a few minutes.
You'll also need to provide a domain for which they key will work for; one key per domain.
The HTML
<!-- SEARCH FORM --> <form action="http://www.google.com/search" method="get"> <!-- HTML5 SEARCH BOX! --> <input type="search" id="search-box" name="q" results="5" placeholder="Search..." autocomplete="on" /> <!-- SEARCH davidwalsh.name ONLY! --> <input type="hidden" name="sitesearch" value="davidwalsh.name" /> <!-- SEARCH BUTTON --> <input id="search-submit" type="submit" value="Search" /> </form> <!-- ASYNCHRONOUSLY LOAD THE AJAX SEARCH API; MOOTOOLS TOO! --> <script type="text/javascript" src="http://www.google.com/jsapi?key=MY_REALLY_REALLY_REALLY_REALLY_REALLY_REALLY_LONG_KEY"></script> <script type="text/javascript"> google.load('mootools','1.2.4'); google.load('search','1'); </script>
You'll want to use a "real" form so that if the user doesn't have JavaScript, they'll get directed to Google for their search. Beyond that, follow the hidden input line to ensure your search will work. You may also note that the search box has autocomplete and placeholder attributes -- those are HTML5 functionality, nothing to do with Google's AJAX Search API.
The CSS
/* results positioning */ #search-results { position:absolute; z-index:90; top:40px; right:10px; visibility:hidden; } /* triangle! */ #search-results-pointer { width:0px; height:0px; border-left:20px solid transparent; border-right:20px solid transparent; border-bottom:20px solid #eee; margin-left:80%; } /* content DIV which holds search results! */ #search-results-content { position:relative; padding:20px; background:#fff; border:3px solid #eee; width:380px; min-height:200px; -webkit-box-shadow: 5px 5px 5px rgba(0, 0, 0, 0.5) }
The CSS above simply position the elements where I want them per my design. I even used a CSS triangle!
The JavaScript
window.addEvent('domready',function(){ /* search */ var searchBox = $('search-box'), searchLoaded=false, searchFn = function() { /* We're lazyloading all of the search stuff. After all, why create elements, add listeners, etc. if the user never gets there? */ if(!searchLoaded) { searchLoaded = true; //set searchLoaded to "true"; no more loading! //build elements! var container = new Element('div',{ id: 'search-results' }).inject($('search-area'),'after'); var wrapper = new Element('div',{ styles: { position: 'relative' } }).inject(container); new Element('div',{ id: 'search-results-pointer' }).inject(wrapper); var contentContainer = new Element('div',{ id: 'search-results-content' }).inject(wrapper); var closer = new Element('a', { href: 'javascript:;', text: 'Close', styles: { position: 'absolute', //position the "Close" link bottom: 35, right: 20 }, events: { click: function() { container.fade(0); } } }).inject(wrapper); //google interaction var search = new google.search.WebSearch(), control = new google.search.SearchControl(), options = new google.search.DrawOptions(); //set google options options.setDrawMode(google.search.SearchControl.DRAW_MODE_TABBED); options.setInput(searchBox); //set search options search.setUserDefinedClassSuffix('siteSearch'); search.setSiteRestriction('davidwalsh.name'); search.setLinkTarget(google.search.Search.LINK_TARGET_SELF); //set search controls control.addSearcher(search); control.draw(contentContainer,options); control.setNoResultsString('No results were found.'); //add listeners to search box searchBox.addEvents({ keyup: function(e) { if(searchBox.value && searchBox.value != searchBox.get('placeholder')) { container.fade(0.9); control.execute(searchBox.value); } else { container.fade(0); } } }); searchBox.removeEvent('focus',searchFn); } }; searchBox.addEvent('focus',searchFn); });
There's a fair amount of JavaScript above so stay with me. The following are the steps for implementing the Google AJAX API:
- Create an element to house the results of the search.
- Create a "Close" link which will allow the user to close the search results window.
- Create our Google-given class instance:
- A Web Search (you can also create Local Search if you'd like...). google.search.WebSearch options. I've chosen to add tabs and set the input as my search box.
- A SearchControl instance. google.search.SearchControl options. "siteSearch" is my suffix for results, I've restricted my search to the davidwalsh.name domain, and form submission will trigger results to display in the current window (instead of a new window).
- A DrawOptions instance. google.search.DrawOptions options. With my DrawOptions instance, I've set search control, set the draw container with the options we've created, and I've decided to use Google's default "no results" message
Once the search controls are created, it's time to attach events to the search box to show and hide the search results container based on the contents of the search box. That's all!
As you can see, I've chosen to use the MooTools (FTW) JavaScript toolkit to create the element that houses the results, the "Close" link, and to bind events to the search box. You could just as easily use Dojo or jQuery for element creation and even handling.
In all honesty, I couldn't believe how easy it was to implement Google AJAX search. It's an easy want to implement search on your website, especially if you're currently using WordPress' search. I recommend taking the time to implement Google's AJAX Search API -- the day it takes you to get it working will save your users hours of pain!
Very nice.
Shouldn’t you be promoting using document.id now instead of $! Shame on you!
I guess when you live in a totally mootools world (how dreamy) you don’t have to worry about things conflicting.
It’s ironic that via the demo, you cant find this blog post :D.
How long does it take for it to index new content?
It’s ironic that via the demo, you can’t find this blog post :D.
How long does it take for it to index new content?
Very useful thank you!
Useful stuff, as always!
Thanks David!
Beautifully done. Not only does this code WORK much better than most, it also LOOKs great as well.
waiting jquery implementation (-:
@none: MooTools was only used for retrieving elements, creating elements, and adding event listeners; all of these are basic parts of jQuery. If you have jQuery experience, this should be easy to implement.
I tried to get this to work. I even uploaded your Moo Tools file and copied the exact script you had on your demo page (of course changing the API key to my own). The search button always loads a Google page without showing the housing element.
Is there something I’m missing?
Hey there, I wrote really quick and dirty jQuery implementation of this, check it out:
http://juhq.wordpress.com/2010/08/03/google-ajax-search-with-jquery/
(the blog entry looks like a mess, sorry about that)
@Kyle Hogan: I’m having this problem as well
David, if you have can you help out? I implemented it the way you wrote it here: http://bit.ly/bhmW3A – Is google not loading mootools correctly? Also, should “set search controls” be “// set search controls”
@Kyle Hogan, @James Lin: Can either of you provide me a link to the pages for which this isn’t working?
@David Walsh: I find a lot of your articles are easy to understand and to implement. However I tried implementing this myself and getting the same problems as the above test… Copied the code word for word… And it is still not working… Is there anything possibly missing?
Always possible, but I need a link to a page to see if I’ve missed something. Are you getting any errors?
Just saw guys that some “//” where remove and you’re getting parse errors. Try my updated code.
@David Walsh: Just tried out the updated code still not working… Here is a link to one that I am working on atm.
http://new.bluesoap.com.au/
@Danz: I found the issue — the searchLoaded variable wasn’t set to true. I’ve adjusted my code above.
Help.
I am going to be crazy after 1 day…cannot get this great google ajax search api.
Can someone send me an example ready to use?
…I got the code from here but it does not works…I am sure it’s a problem to my side.
of course I got my key and replaced thedavidwalsh.name with my domain
thanks
My test page
http://www.byman.it/google/searchform.php
@David Walsh: Unfortunately I still can’t get it to work with the updated code. Any help is appreciated! http://www.thespectrum.net/search/
Good job but you must include a crappy Google’s brand somewhere… look at
I got my google key, I copied all code and changed : key and site for search…but
nothing, someone can send me the right code via mail in a file?
thanks
http://www.byman.it/google/searchform.php
@byman64: You’re missing all of the MooTools code in my post.
@James Lin: Put all of the MooTools code below your
google.load()
stuff, and wrap it in awindow.addEvent('domready',function(){ /*moo here */ });
block.Thanks to all…code sorted…I think
I also added
Now I see the result box but empty…
other suggests?
pls
@David Walsh: Hey man, thanks for the update. It works perfectly now.
@Danz:
It only works for the windows but no data appear …
If I type site:byman.it google show a lot of results…
any idea?
@byman64: I found out why it was not working for you properly… On line 52 you repeated “jsapi?key=” twice. I copied your code and remove the repeated part and it works like a charm… Try that out.
thanks! I am not a really expert but I failed a copy and past…I was really tired…
btw thanks to you now it works…
I down know if google relase really all pages…or something is still wrong.
If I type for ie s now i see something, but if I type sa no result…
This is what google knows
http://www.google.it/search?sourceid=chrome&ie=UTF-8&q=site:byman.it
pls can you test the google ajax search
http://www.byman.it/google/searchform.php
ciao
thanks again for great support
ops….of course you right…I think it is stressing me…copy and past error…
well now I see some results but there is a strange result.
I notes just a couple of results…try to type a search something and if you get some results read a word and try to serch it.
No result with 2 chars inserted.
Please, test it.
thanks
Here the google ajax search
========================================
http://www.byman.it/google/searchform.php
This is what google knows of byman.it
==========================
http://www.google.it/search?sourceid=chrome&ie=UTF-8&q=site:byman.it
Works for me!
Hey Its works fine Great work.
Thank you David.
Have you guys noticed that the search result links are always “davidwalsh.name” whereas when you search Google you get “davidwalsh.name/contact” (For example). Any ideas on how to fix this? (Check out my JQuery implementation with a Lightbox on http://www.softkube.com). Cheers :D
Hi Dave, this is a great tutorial. Even I could follow it!
At least I thought I had, but my ajax box is not appearing on search. Instead search takes the user to a new page as per normal. I’ve checked and double checked my code, updated to the latest mootools version, tried both inline and separate file for scripts.
What are the common pitfalls, and where should I be looking for problems? Really appreciate any pointers to help me learn how this works!
Would you like to explain how to get faster loading for ajax api.. :) Peace
Thank you David.
Just a little note about setting the target to self on the search options.
In order to be effective this option should be set right after calling the control.addSearcher(search);
Here is same script on jQuery:
download
. Look it in work with small changes possiblehere
.Thank you David
The code is very good,
But i see the result search limited, it just show max 36 result.
I think that we must charge $ for google to get full result ??
A really helpful post but the search API is depreciated :((
How can I make search for exact words?
How can I evaluate in codes that, my words have been found or not?
how about implement on Dojo..?
I clicked the link to get the API key, and Google says that API keys are no longer required for Loader.
Does this break the code? I have it loaded on my site, but the search doesn’t implement AJAX instead it takes the user to Google and provides results there.
http://www.mermagica.com/store-test/index.php?main_page=page&id=13&chapter=0
Javascript is enabled on my browser, and other scripts work fine.
Any help you can provide is appreciated!
Found this to be extremely useful many thanks