How to Create a QR Code
QR codes aren't everyone's cup of tea but I quite like them. If I see something I want to remember or check out later, especially when on the road, it's super easy to take a quick picture -- it's much easier than trying to remember a URL and much faster than typing it in on a tiny keyboard.
If you need to generate QR codes, for a client or yourself, there's a really nice JavaScript project: node-qrcode. Let's look at the different wys and output formats you can use to create a QR code!
Start by installing the library:
yarn add qrcode
Create QR Code Image Data
With the QR code utility available, you can generate a data URI for the QR code which you can use with an <img>
element:
const generateQR = async text => { try { console.log(await QRCode.toDataURL(text)) } catch (err) { console.error(err) } } generateQR("https://davidwalsh.name"); /* data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAIQAAACECAYAAABRRIOnAAAAAklEQVR4AewaftIAAAOMSURBVO3BQY7kRgADwWSh///l9B584EmAIPV41mBE/IOZfx1mymGmHGbKYaYcZsphphxmymGmHGbKYaYcZsphphxmymGmHGbKh4eS8JNUWhKayh1JaCotCU3ljiT8JJUnDjPlMFMOM+XDy1TelIQ7ktBUrqi0JNyRhKZyReVNSXjTYaYcZsphpnz4siTcofKbqHxTEu5Q+abDTDnMlMNM+fCXU2lJaEloKnckoak0lf+Tw0w5zJTDTPnwl0vCFZWWhKbyRBKayt/sMFMOM+UwUz58mco3qVxJQlP5TVR+k8NMOcyUw0z58LIk/KQkNJU7ktBUWhLelITf7DBTDjPlMFM+PKTyX1JpSWgqdyThTSp/k8NMOcyUw0z58FASmsqVJPykJDSVKyotCXckoalcSUJTaUm4Q+WJw0w5zJTDTIl/8EVJuKLSktBUnkhCU2lJeELljiS8SeVNh5lymCmHmRL/4Acl4YpKS0JTuSMJTeVKEppKS8ITKi0JV1SuJKGpPHGYKYeZcpgp8Q9elIQrKi0JV1RaEppKS0JT+aYkNJWWhCsqLQlPqDxxmCmHmXKYKR8eSkJTuUOlJaEl4Q6VloSm0pLQVK4k4ZtU7kjCmw4z5TBTDjMl/sEDSXhC5Y4kXFFpSWgqV5LwJpUrSWgqV5LQVN50mCmHmXKYKR++TOWOJHxTEu5QaUloKi0JLQlN5UoS7khCU3niMFMOM+UwUz68TKUloam0JDSVK0m4Q6Uloam8SeVKEppKS8IVlZaENx1mymGmHGZK/IMHktBUWhKuqFxJwhMqdyShqbQkfJNKS8IVlTcdZsphphxmyoeHVK6oPKFyJQlN5UoSvknljiT8JoeZcpgph5ny4aEk/CSVK0m4Q6Ul4YpKS8KVJDSVO1SuJKGpPHGYKYeZcpgpH16m8qYkvEmlJeGOJNyh8qYkNJU3HWbKYaYcZsqHL0vCHSpPqLQk3KHSknBHEn5SEprKE4eZcpgph5ny4X9O5YrKHSotCXeo/GaHmXKYKYeZ8uEvp9KS0FSuJKGpNJUrKi0JTeVKEn6Tw0w5zJTDTPnwZSr/pSQ0laZyJQlN5YkkXFFpSfhJh5lymCmHmfLhZUn4SUm4onIlCU8koam0JDSVK0loKleS8KbDTDnMlMNMiX8w86/DTDnMlMNMOcyUw0w5zJTDTDnMlMNMOcyUw0w5zJTDTDnMlMNM+Qeve5EOUrEhtwAAAABJRU5ErkJggg== */
Create a QR Code in Terminal
If you want to see the QR code in the terminal via Node.js, you can do so by passing a config object:
const generateQR = async text => { try { console.log(await QRCode.toString(text, {type: 'terminal'})) } catch (err) { console.error(err) } }
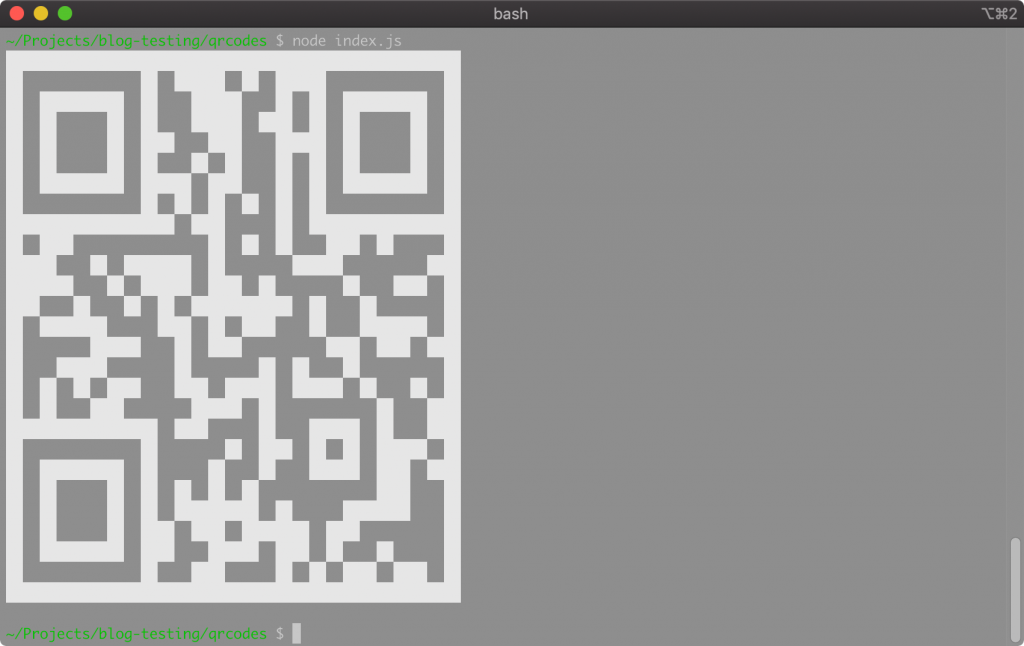
Create a QR Code Image
You can generate a PNG, SVG, or UTF8 image for the QR code:
const generateQR = async text => { try { await QRCode.toFile('./davidwash-qr-code.png', text); } catch (err) { console.error(err) } }
Create a QR Code on Canvas
If you use a utility like Browserify and webpack, you can use qrcode
on the client side:
var canvas = document.getElementById('canvas'); const generateQR = async text => { try { await QRCode.toCanvas(canvas, text) } catch (err) { console.error(err) } } generateQR("https://davidwalsh.name");
This awesome QR code library also allows you to create much more than I've shown here, including binary data and with a variety of options. If you need to create a QR code, look no further than node-qr code!
https://chart.googleapis.com/chart?chs=300×300&cht=qr&choe=UTF-8&chl=https://davidwalsh.name
I thought you were going to show how to do it without libraries :(
Any library for creating QR code in ReactJS ?
is it a link of a QRcode