Fix Flow Node Issue “property querySelector of unknown”
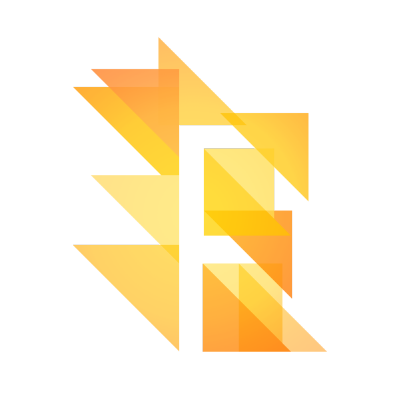
Flow, the static type checker used in many React projects, feels like a gift and a curse at times; a gift in that it identifies weaknesses in your code, and a curse that sometimes you feel like you're needlessly adjusting your code to satisfy Flow. I've grown to appreciate Flow but that doesn't mean I end up spending extra hours finding new ways to code.
I recently ran into an issue where I was querying for a node in a React element and then using querySelector
on that node to find a child; surprisingly Flow took issue:
Cannot call node.querySelector because property querySelector of unknown type [1] is not a function. 71│ const { maxHeight } = this.state; 72│ const node = ReactDOM.findDOMNode(this); [1] 73│ if (node && node.querySelector) { 74│ const popupNode = node.querySelector(".preview-popup"); 75│ if (popupNode) { 76│ popupNode.style.maxHeight = `${maxHeight}px`; 77│ } /private/tmp/flow/flowlib_34a4c903/react-dom.js [1] 14│ ): null | Element | Text; Found 1 error
It turns out that findDOMNode
can return type Text
, and thus querySelectorAll
would be undefined
; Flow doesn't like undefined
. The solution is to use instanceOf
with HTMLElement
:
if (node instanceOf HTMLElement) { // ... }
The solution makes sense but a part of me silently rages that && node.querySelector
doesn't qualify. In the end, Flow is so helpful that little changes like this don't get me too wound up.