The Simple Intro to SVG Animation
This article serves as a first step toward mastering SVG element animation. Included within are links to key resources for diving deeper, so bookmark this page and refer back to it throughout your journey toward SVG mastery.
An SVG element is a special type of DOM element that mimics the syntax of a standard HTML element. SVG elements have unique tags, attributes, and behaviors that allow them to define arbitrary shapes -- essentially providing the ability to create an image directly within the DOM, and thereby benefit from the JavaScript- and CSS-based manipulation that DOM elements can be subjected to.
As a teaser of what we're about to learn, check out these demos that are only possible thanks to SVG:
There are three significant benefits to creating graphics in SVG rather than using rendered images (PNG, JPEG, etc.): First, SVG compresses incredibly well; graphics defined in SVG have smaller file sizes than their PNG/JPEG equivalents. Second, SVG graphics scale to any resolution without the loss of clarity; they look razor sharp on all desktop and mobile screens. Third, you can animate the individual components of an SVG graphic at run-time (using JavaScript and CSS).
To create an SVG graphic, either design it by hand using DOM elements to represent each piece of your graphic, or use your favorite photo editor to draw arbitrary shapes then export the resulting SVG code for copy-pasting into your HTML. For a primer on exporting SVGs, read this fantastic article: Working With SVG.
SVG Animation
Neither jQuery nor CSS transitions offer complete support for the animation of SVG-specific styling properties (namely, positional and dimensional properties). Further, CSS transitions do not allow you to animate SVG elements on IE9, nor can they be used to apply transforms to SVGs on any version of IE.
Accordingly, to animate SVG elements, either use a dedicated SVG manipulation library or a JavaScript animation library that has support for SVG. The most popular dedicated SVG manipulation library is Snap.svg, and the most popular JavaScript animation library with SVG support is Velocity.js. Since Velocity.js contains extensive cross-browser SVG support, is lightweight, and should already be your weapon of choice for web animation, that's the library we'll be using in this article.
Velocity.js automatically detects when it's being used to animate an SVG element then seamlessly applies SVG-specific properties without you having to modify your code in any way.
SVG Styling
SVG elements accept a few of the standard CSS properties, but not all of them. (More on this shortly.) In addition, SVGs accept a special set of "presentational" attributes, such as fill, x, and y, which also serve to define how an SVG is visually rendered. There is no functional difference between specifying an SVG style via CSS or as an attribute -- the SVG spec merely divides properties amongst the two.
Here is an example of an SVG circle element next to an SVG rect element -- both of which are contained inside a mandatory SVG container element (which tells the browser that what's contained within is SVG markup instead of HTML markup). Notice how color styles are defined using CSS, but dimensional properties are defined via attributes:
<svg version="1.1" width="300" height="200" xmlns="http://www.w3.org/2000/svg"> <circle cx="100" cy="100" r="200" style="fill: blue" /> <rect x="100" y="100" width="200" height="200" style="fill: blue" /> </svg>
(There are other special SVG elements, such as ellipse, line, and text. For a complete listing, refer to MDN.)
There are three broad categories of SVG-specific styling properties: color, gradient, dimensional, and stroke. For a full list of SVG elements' animatable CSS properties and presentational attributes, refer to Velocity.js's SVG animation documentation.
The color properties consist of fill and stroke. fill is equivalent to background-color in CSS, whereas stroke is equivalent to border-color. Using Velocity, these properties are animated the same way that you animate standard CSS properties:
// Animate the SVG element to a red fill and a black stroke $svgElement.velocity({ fill: "#ff0000", stroke: "#000000" }); // Note that the following WON'T work since these CSS properties are NOT supported by SVG: $svgElement.velocity({ backgroundColor: "#ff0000", borderColor: "#000000" });
The gradient properties include stopColor
, stopOpacity
, and offset
. They are used to define multi-purpose gradients that you structure via SVG markup. To learn more about SVG gradients, refer to MDN's SVG Gradient Guide.
The dimensional properties are those that describe an SVG element's position and size. These attributes differ slightly amongst SVG element types (e.g. rect vs. circle):
// Unlike HTML, SVG positioning is NOT defined with top/right/bottom/left, float, or margin properties // Rectangles have their x (left) and y (top) values defined relative to their top-left corner $("rect").velocity({ x: 100, y: 100 }); // In contrast, circles have their x and y values defined relative to their center (hence, cx and cy properties) $("circle").velocity({ cx: 100, cy: 100 });
// Rectangles have their width and height defined the same way that DOM elements do $("rect").velocity({ width: 200, height: 200 }); // Circles have no concept of "width" or "height"; instead, they take a radius attribute (r): $("circ").velocity({ r: 100 });
Stroke properties are a unique set of SVG styling definitions that amount to putting the CSS border property on steroids. Two key differences from border are the ability to create custom strokes and the ability to animate a stroke's movement. Use cases include handwriting effects, gradual reveal effects, and much more. Refer to SVG Line Animation for an overview of SVG stroke animation.
Putting it all together, here's a complete SVG animation demo using Velocity.js:
See the Pen Velocity.js - Feature: SVG by Julian Shapiro (@julianshapiro) on CodePen.
Fork that pen and start toying around with SVG animation.
Positional Attributes vs. CSS Transforms
You may be wondering, What's the difference between using the x/cx y/cy positional attributes instead of using CSS transforms (e.g. translateX, translateY)? The answer is browser support: IE (including IE11) do not support CSS transforms on SVG elements. As for the topic of hardware acceleration, all browsers (including IE) hardware-accelerate positional attributes by default -- so, when it comes to SVG animation performance, attributes are equivalent to CSS properties.
To summarize:
// The x and y attributes work everywhere that SVG elements do (IE8+, Android 3+) $("rect").velocity({ x: 100, y: 100 }); // Alternatively, positional transforms (such as *translateX* and *translateY*) work everywhere EXCEPT IE $("rect").velocity({ translateX: 100, translateY: 100 });
Diving Deeper
With our introduction to SVG animation complete, head on over to MDN's SVG guide for an in-depth walkthrough of every aspect of working with SVG's.
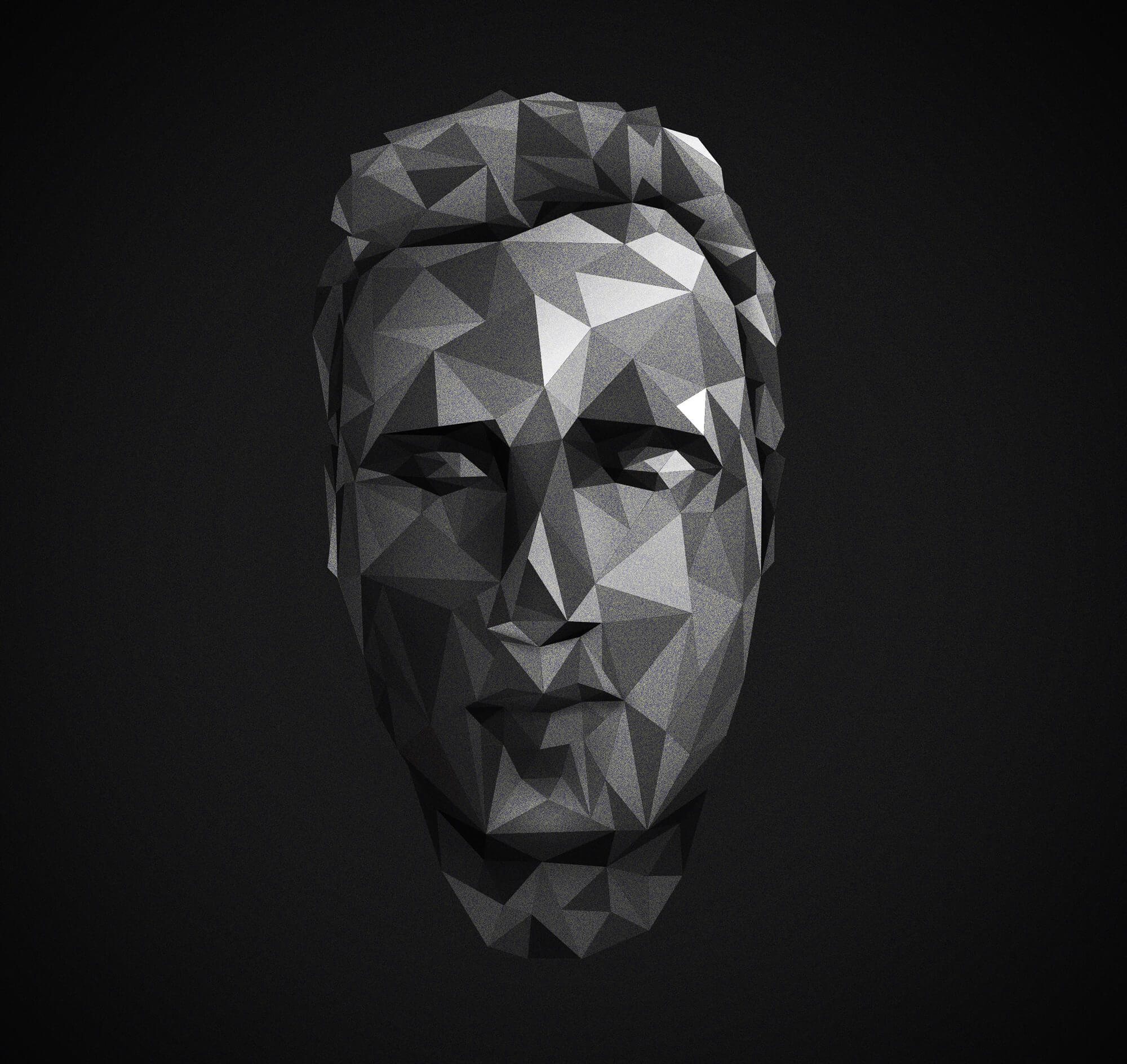
About Julian Shapiro
Julian Shapiro is a startup founder and full-stack developer from Vancouver. Read more about him at Julian.com.
To make a data flow diagram in runtime do you suggest SVG?
If you’re looking to detect mouse events, then yes — SVG is a reasonable option.
while the idea of this article is interesting, it’s not useful because it relies on jQuery to work. Not generic enough, therefore not a good introduction to SVG in HTML.
While I see your point, that’s still a very naive comment.
Regardless, Velocity is dropping its jQuery dependency in about 2 weeks. It’ll run completely stand alone at that time (or with jQuery, if it’s present on the page).
This is brilliant thanks, been using SVG where possible on websites, jQuery or no!
Thank you for this post! I think using jQuery isn’t that bad! It’s selecting elements, to work with.. that’s completely ok i think. Then, when it comes to animation and performance of course, i’ll be using velocity functions forever now.
Greetings from Germany
I’m fascinated with the “Isotyope Demo”. I’m wondering how to produce those points for svg? Is there a tool that can export it to a 3d svg? With adobe illustrator, the svg is just a flat 2d polygons.
is velocity.js really better than GSAP.JS for animating SVG ?
Pure css animations are better than both. A plugin only provides a simplified interface to lower level tools; they never reinvent the wheel
Velocity is adapted to UI, Gsap is better for game.
Velocity have a syntaxe better to read than Gsap too.
Good to note that velocity won’t animate a fill color from transparent to solid colors (it’ll start with white if initial state has fill=none)
Please guide me if I want to make animation like this video. https://www.youtube.com/watch?v=5Be2YnlRIg8
Which method should I use
Good to note that velocity won’t animate a fill color from transparent to solid colors
Velocity.js is praised here and elsewhere, but doesn’t seem to be actively supported anymore. Am I right?