MooTools, Mario, and Portal
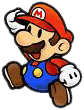
I'm a big fan of video games. I don't get much time to play them but I'll put down the MacBook Pro long enough to get a few games in. One of my favorites is Portal. For those who don't know, what's wrong with you? Portal is a strategic game that requires a lot of thinking and good timing. You get two "portal guns" which allow you to get from place to place. Check out some gameplay here.
While Portal now has a place in my heart, it needs to share space with the original Mario games. Don't ask me why, but I got the idea of combining the two in my head. And I'm not a video game programmer so I needed to rock MooTools to accomplish it. It was actually quite simple.
The XHTML
<!-- LIGHTS and CAMERA --> <div id="stage"> <!-- LEFT TUBE --> <img id="tube-left" src="mario-pipe.png" alt="Left Pipe" /> <!-- RIGHT TUBE --> <img id="tube-right" src="mario-pipe.png" alt="Right Pipe" /> <!-- LEFT MARIO --> <img id="mario-left" src="mario-left.png" alt="Mario!" /> <!-- RIGHT MARIO --> <img id="mario-right" src="mario-right.png" alt="Mario!" /> <!-- FLOOR --> <div id="floor"></div> </div>
Just a stage and some imagery which will be manipulated with CSS and MooTools JavaScript.
The CSS
#stage { width:600px; height:250px; position:relative; background:#5573EB; } #stage img { display:block; } #tube-left { position:absolute; bottom:0; left:100px; z-index:5; } #tube-right { position:absolute; bottom:0; right:100px; z-index:5; } #mario-left { position:absolute; bottom:0; left:110px; z-index:3; } #mario-right { position:absolute; bottom:0; right:110px; z-index:3; } #baddy { position:absolute; bottom:30px; left:180px; z-index:5; } #box { position:absolute; bottom:130px; left:290px; z-index:5; } #floor { position:absolute; bottom:0; left:0; height:30px; width:600px; background:url(mario-floor.png) 0 0; right:0; }
The absolute positioning is key here. The hardest part is measuring things up -- once you've got that, you're golden. Also be sure that the pipes have a larger z-index than the Marios.
The MooTools JavaScript
(function($) { window.addEvent('domready',function() { /* the master */ var animation = function() { /* settings */ var upDuration = 300, downDuration = 500, upHeight = 200, baddyDuration = 10000, rightDistance = 400; var lefty = $('mario-left'), righty = $('mario-right'), baddy = $('baddy'); /* left animations time! */ var leftFxUp = new Fx.Tween(lefty,{ duration: upDuration, onComplete: function() { leftFxDown.start('bottom',0); } }).start('bottom',upHeight); var leftFxDown = new Fx.Tween(lefty,{ duration: downDuration, onComplete: function() { rightFxUp.start('bottom',upHeight); } }); /* right animation time! */ var rightFxUp = new Fx.Tween(righty,{ duration: upDuration, onComplete: function() { rightFxDown.start('bottom',0); } }); var rightFxDown = new Fx.Tween(righty,{ duration: downDuration, onComplete: function() { leftFxUp.start('bottom',upHeight); } }); /* baddy animation */ var baddyRightFx = new Fx.Tween(baddy,{ duration: baddyDuration, onComplete: function() { baddyLeftFx.start('left',rightDistance); } }); var baddyLeftFx = new Fx.Tween(baddy,{ duration: baddyDuration, onComplete: function() { baddyRightFx.start('left',180); } }).start('left',rightDistance); }; /* ACTION! */ animation(); }); })(document.id);
The MooTools JavaScript is the fun part. I create 4 Fx.Tween objects that will act as the "up" and "down" animations. Once one finishes, the next one is directed start. So simple!
I know: ridiculous. It was a great way to mix the old school an new school though!
Very nice effect !
It’s so funny !
It’s one of those very cool, yet useless items on the web. But it can help you dissect the code and understand the animation that’s being used here.
Keep it up Walsh. Love your posts!
Add some easing and it will seem like there are real-time browser-based physics at work :) How do you come up with this stuff? It is cool though.
The vertical effects should be parabolic so it looks like gravity is at work. (
Fx.Transitions.Quad
)The walking mushroom is a touch of genius :P
That really put a smile on my face. Thanks!
does this mean you could add keyboard events to move the mario image?
That was really cool. I also used to play Mario when I was a child but not anymore.
:)