Linkify Your Twitter Feed
So, you want to display your Twitter status on your blog? No problem, use the API. But, what if you want to display links in your status like twitter.com itself does? No problem, use regular expressions.
Here is a function that will turn all HTTP URLs, Twitter @usernames, and #tags into links:
The PHP
function linkify_twitter_status($status_text) { // linkify URLs $status_text = preg_replace( '/(https?:\/\/\S+)/', '<a href="\1">\1</a>', $status_text ); // linkify twitter users $status_text = preg_replace( '/(^|\s)@(\w+)/', '\1@<a href="http://twitter.com/\2">\2</a>', $status_text ); // linkify tags $status_text = preg_replace( '/(^|\s)#(\w+)/', '\1#<a href="http://search.twitter.com/search?q=%23\2">\2</a>', $status_text ); return $status_text; }
Using It
There are many ways to grab statuses from Twitter. Here, I will show a very simple method with no caching -- suitable for demonstration purposes. Feel free to substitute your favorite Twitter-fetching method.
$url = 'http://twitter.com/statuses/user_timeline/davidwalshblog.json?count=1'; $json = file_get_contents($url); $statuses = json_decode($json); $status_text = $statuses[0]->text; echo "before: ", $status_text, "\n"; echo "after: ", linkify_twitter_status($status_text), "\n";
The above code turns:
@davidwalshblog: Check it out http://is.gd/hd2D #awesome..into:
@<a href="http://twitter.com/davidwalshblog">davidwalshblog</a>: Check it out <a href="http://is.gd/hd2D">http://is.gd/hd2D</a> #<a href="http://search.twitter.com/search?q=%23awesome">awesome</a>
Pretty simple, eh?
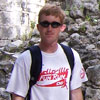
About Jeremy Parrish
Jeremy Parrish is the Systems Administrator for Econoprint, a print/design/web company in Verona, Wisconsin. He has a BS in Computer Engineering and Computer Science from the University of Wisconsin--Madison. In his spare time he enjoys running marathons with his wife and working on various coding projects. You can check out Jeremy's blog at http://rrish.org/.
Great article!
Hey David!
This works just fantastic. Took like 2 mins for me to impliment it into my website and I am a total php beginner!
Thank you so much!
Cheers from Bonn, germany,
Oliver
Hi Oliver,
I’m glad it worked for you. It’s nice when you can add something useful in just 2 minutes!
Exactly what I’ve been looking for actually. Thanks :)
Nice work Jeremy. Nice to see someone putting Regexs to good use!
Hi, i think @Luke’s website contains a trojan in his favicon.ico file. Check it.
Wow, I needed this. Thanks a lot.
Jeremy!
Thank you so much. I have been searching and searching for any easy way to do this that was not already buried in a wordpress plugin or some other place where there were a bunch of extras I didn’t need. All I wanted was to call a simple function passing username and the number of statuses to echo.
I combined all your stuff and wrote a function called get_Tweets() . It takes $username and $count as variables, loops through the count and echos $count number of statuses as LI elements. So simple, yet I couldn’t find the starting point anywhere!
To include it with the wordpress theme I am building, I just stuck the finished function in my functions.php file then called get_Tweets(‘USERNAME’, ‘COUNT’) between a UL tag.
I wanted to include a copy of the code in here, but it didn’t seem to want to let me include the php and it looked a little confusing.
I would be happy to share with anyone who is interested.
Thanks again, Jeremy!
Going to subscribe to your RSS and check out the rest of your site.
Marty
@Marty: Thanks for the note. I’m glad you found it useful! If you want, you could probably throw your code up on David’s pastebin and throw a link back here in the comments. It sounds like it might be useful for others.
I’ve created a wordpress plugin that will automatically create tag and user links in your posts/pages/comments when #tagname or @username are used… However, I’ve just noticed an issue. When the function runs hooked to “the_content()” if you have any inline styles (color=”#ab0000;”) then the colors are assumed to be tags since they have the pound symbol in front of them.
Is there a way to make the regex differentiate between css color codes and tags you insert?
@Josh: The function should really only be passed the actual tweet text, without any container markup. However, if you can assure that there is no whitespace character immediately preceding the ‘#’ character (e.g. “color:#ab0000;”), you should be OK. I still wouldn’t recommend using it that way, though.
Any idea on why I’m getting a “Fatal error: Call to undefined function: json_decode()”? I’m running PHP 5.2.9 so I believe this function would be in there.
Thank you so much for creating this! I’ve got everything else sorted for a little Twitter App I’m working on, but I have no clue when it comes to Regex in PHP, so this is perfect!
Thanks!!
Save my day…
Im kind of a dummy with this stuff but I thought I was supposed to put everything inside of the function.php file, but that doesnt seem to work.
What about URLs that contain hash characters? Won’t the part of the URL after the tag get linkified as a hashtag?
thank you very much. i have been searching for this for two days and could not find any solutions. but luckily i see this and done in 10 minutes.
Great article. Thanks. Helped me.
Thanks for the regexp!
Hi David,
i use your code and this code to display feeds on my page $this->linkify($feed_item->text) and I have small problem if you can help? The result is Twitter results feedhttp://t.co/F6xyyR9Ee7 and I would like to be like this Twitter results feed. can you help me? Any idea how I could do? Thank you very much
i write code result again:
this is the result:
Twitter results feed http://t.co/F6xyyR9Ee7
and i want to be like this
Twitter results feed
basically want “Twitter results feed” to be inside of tag
Robert:
replace this:
with this:
Sorry, my code pasted wrong.
I meant to say replace the
\1
with whatever text you want the link to be in the second parameter of thepreg_replace
functionhttps://gist.github.com/anonymous/9237526
Any ideas on how to use this, but grab the display name of the URL to use as the anchor output instead of just the URL itself.
For example.. If Im trying to promote my blog site and I have a tweet that says: “MyBlog.com has undergone a facelift and is ready for everyone to read.” The replacement link would say “http://t.co/12345abc has undergone…”.
This is ugly and ruins the read… How would one get the display name from the feed and use that to put in the anchor instead of the ugly URL?