Link Nudging Using Dojo
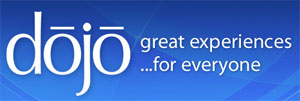
In the past we've tinkered with link nudging with MooTools and link nudging with jQuery. In an effort to familiarize myself with other JavaScript frameworks, we're going to try to duplicate that effect with another awesome framework: Dojo.
The JavaScript: Attempt 1
dojo.addOnLoad(function() { var links = dojo.query('a.nudge'); //foreach... dojo.forEach(links,function(link) { var left = dojo.style(link,'paddingLeft'); dojo.connect(link,'onmouseenter',function() { dojo.animateProperty({ node:link, properties: { paddingLeft: (left + 10) } }).play(); }); dojo.connect(link,'onmouseleave',function() { dojo.animateProperty({ node:link, properties: { paddingLeft: left } }).play(); }); }); });
Once the DOM is ready, we use the dojo.query method to find all of the links to nudge. For every link we find, we record its original left padding and add mouseenter and mouseleave events to each link to animate its left padding.
The JavaScript: Better Solution
dojo.ready(function() { dojo.query('a.nudge').forEach(function(link){ var left = dojo.style(link,'paddingLeft'); dojo.connect(link,'onmouseenter',function() { dojo.anim(link, { paddingLeft:20 }); }); dojo.connect(link,'onmouseleave',function() { dojo.anim(link, { paddingLeft:left }); }); }); });
Dojo lead Pete Higgins showed me a more condensed version of his script.
Simple, no? The best way to learn to use any JavaScript framework is to duplicate a given set of code you are familiar with, much like we did here. What do you think about this Dojo example? Look close to jQuery and MooTools?
The mouseenter/mouseleave thing is so common it is actually combined as an API in plugd (soon to be in Dojo proper). With plugd, the Dojo code could look like:
Couldn’t agree more on what you said: “The best way to learn to use any javascript framework is to duplicate a given set of code you are familiar with”
By the way, I think the Mootools code is more semantic than the rest, I mean, with jQuery one has to know that .hover is an event, unlike MooTools which says
.addEvent
.I liked Dojo’s aproch, but the .connect is new to me, but just by reading it I understood that it will connect link to the event and the function.
Very nice example and keep up the good work.
Hi Rafael / David
@Rafael F P Viana: You can use jQuery’s bind method which is the same as MooTool’s addEvent essentially.
Good post David, I actually really like Dojo’s layout so I might investigate. However, it’s clear that MT/jQ are the top two so I think I’m concentrating my skill on those two.
Nice one. One note/question – if your anchors needed a default padding-left of 15px, then the effect would be muted a bit. Rather than setting padding-left to a static 20, what about changing line 5 to a relative 20?
dojo.anim(link, { paddingLeft: (left + 20) });
@Brad Pritchard: Much better — I’ll update the post!
Do you just limiting to Dojo Base? In Dojo Core there is
dojo.behavior
that has some additional functionality.http://www.dojotoolkit.org/api/dojo/behavior.html
With
dojo.behavior
you can write your code like this:The good part on
dojo.behavior
is that you can calldojo.behavior.apply()
as often as you like. It only updates your DOM incremental. Meaning it only adds the events to newly created DOM nodes, or only add things to a node that you added after the last call todojo.behavior.apply()
.That means you can do some ajax add nodes to your DOM, and call
dojo.behavior.apply()
after it. And the new nodes get your defined behavior.@Sid Burn: Thanks for the tip Sid. By the way, I’ve never given a link the “nude” tag before. :)
Ah “nude” oder “nudge” for a non-native english speaker it is almost the same. ;)