Retrieve Your Gmail Emails Using PHP and IMAP
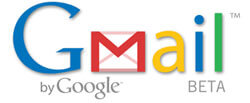
Grabbing emails from your Gmail account using PHP is probably easier than you think. Armed with PHP and its IMAP extension, you can retrieve emails from your Gmail account in no time! Just for fun, I'll be using the MooTools Fx.Accordion plugin to display each email.
The CSS
div.toggler { border:1px solid #ccc; background:url(gmail2.jpg) 10px 12px #eee no-repeat; cursor:pointer; padding:10px 32px; } div.toggler .subject { font-weight:bold; } div.read { color:#666; } div.toggler .from, div.toggler .date { font-style:italic; font-size:11px; } div.body { padding:10px 20px; }
Some simple CSS formatting.
The MooTools JavaScript
window.addEvent('domready',function() { var togglers = $$('div.toggler'); if(togglers.length) var gmail = new Fx.Accordion(togglers,$$('div.body')); togglers.addEvent('click',function() { this.addClass('read').removeClass('unread'); }); togglers[0].fireEvent('click'); //first one starts out read });
Some simple accordion code.
The PHP
/* connect to gmail */ $hostname = '{imap.gmail.com:993/imap/ssl}INBOX'; $username = 'davidwalshblog@gmail.com'; $password = 'davidwalsh'; /* try to connect */ $inbox = imap_open($hostname,$username,$password) or die('Cannot connect to Gmail: ' . imap_last_error()); /* grab emails */ $emails = imap_search($inbox,'ALL'); /* if emails are returned, cycle through each... */ if($emails) { /* begin output var */ $output = ''; /* put the newest emails on top */ rsort($emails); /* for every email... */ foreach($emails as $email_number) { /* get information specific to this email */ $overview = imap_fetch_overview($inbox,$email_number,0); $message = imap_fetchbody($inbox,$email_number,2); /* output the email header information */ $output.= '<div class="toggler '.($overview[0]->seen ? 'read' : 'unread').'">'; $output.= '<span class="subject">'.$overview[0]->subject.'</span> '; $output.= '<span class="from">'.$overview[0]->from.'</span>'; $output.= '<span class="date">on '.$overview[0]->date.'</span>'; $output.= '</div>'; /* output the email body */ $output.= '<div class="body">'.$message.'</div>'; } echo $output; } /* close the connection */ imap_close($inbox);
With our individual username/password settings set, we connect to Gmail. Once connected, we request all emails. If we find emails, I reverse sort the emails so that the newest emails appear on top. For every email we receive, I output the subject and message in MooTools Fx.Accordion format.
Now that I've shown you the basics, it's time for you to build your next great PHP email app! Go ahead and send a few emails to davidwalshblog@gmail.com to see your eamil display on the page!
There are some encoding issues and I've not found a great way to include images. Any tips appreciated!
great and useful many thanks David!
hello,
I get this error
this is from:
please help
Your server has not installed the IMAP extension, it should be enabled for the use of all IMAP functions, in your php.ini search the line:
and restart apache service. Good Luck
hello, but I am at GoDaddy Shared Hosting (linux) and have no access to my php.ini file.
Any Idea?
open cpanel then select
PHP_SETTING
, and change the PHP version to PHP 5.6, then enable check onphp_imap
modulenice shot Dave!
I love it. Wish I could mark emails as read when you toggle them though.
Oh, and I noticed a flicker when toggling “unread” emails.
NVM the flicker thing, guess it was my FF acting weird :P
Your picture is outdated…gmail is out of beta ;)
I did that on purpose. Always beta!
O
could you help me get into my account, i forgot the password i would greatly appreciate it. i listed my gmail address(vh7780@gmail.com
)
how can one get a list of contacts of a gmail account? Facebook and most social networking sites do that…but how?
Awesome post. Can we do it for other emails ie Yahoo, Hotmail etc.
wow this is great code. Congratulations…
how to display new mails only?
@scvinodkumar – The method imap_fetch_overview() returns an array, one parameter of which is called ‘seen’. This flag indicates whether the message has been read or not. Likewise you have ‘answered’, whether you’ve replied to the message.
Good post David, thank you.
@Devang Paliwal: Absolutely! You’d need to get each service’s IMAP information though.
Is it secure? Could this be implemented with accounts contain personal and confidential mails?
@Zues: How you use this is up to you. I don’t recommend making your primary email account public.
Hmmm okay. But this is something great. Can brainstorm and come up with new and cool ideas :)
Can someone help ?
@soso: please enable “extension=php_imap.dll” in php.ini
I want get attachment de Gmail. How? Thanks.
Hey this looks awesome!
I tried to use it with wamp and am getting this error…any ideas? Thanks a lot for any help!!
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in C:\wamp\www\email\howdy.php on line 13
Cannot connect to Gmail: Can’t open mailbox {imap.gmail.com:993/imap/ssl}INBOX: invalid remote specification
@chris: Not sure, could be an outbound connection or firewall issue. “Localhost” always bring trouble.
Thanks David, you were correct it was a firewall issue that blocked 993.
@chris:i have the same problem and i cannot understand how to solve this problem pls explain me
is this works for inline attachments such as picture added inside the text through outlook express?
Great stuff!
Q? Why I get this error : Cannot connect to Gmail: Can’t open mailbox {imap.gmail.com:993/imap/ssl}INBOX: invalid remote specification
@Iván: I had this same error, it’s your firewall settings blocking 993 port. When you unblock it, it works.
I´ve fixed my problem.
Thank You Chris.
please explain how did fixed your problem..same problem for me till now i didn’t fix my problem
Thank you so much you are genius!!!
great idea,
demo is down though, “Cannot connect to Gmail: Too many login failures”
turn on your less secure apps on your email. visit https://www.google.com/settings/security/lesssecureapps. it’s work for me
i also change the
$hostname
to this –>@nimz: Ah, a Chelsea boy. Nice. Since I posted the article the accounts been hacked at. I’ll see if I can restore it.
Cannot connect to Gmail: Can’t open mailbox {imap.gmail.com:993/imap/ssl}INBOX: invalid remote specification – this error i get, when i run this code in localhost means local system..
hi varun,
On August 31, 2009 you had 1 doubt that “Cannot connect to Gmail: Can’t open mailbox {imap.gmail.com:993/imap/ssl}INBOX: invalid remote specification ” for that gmail retrieve question on “http://davidwalsh.name/gmail-php-imap”
will you tell me how did you fixed that error
since am having same problem here.
Thank You
dude.. awesome job.. but im unsing latin characters and i got ugly formed emails.. there is any parameter for change the charset?
This doesn’t work for me for some reason. I’m running it locally on Wamp, and I turned the firewall off… I can get the email subjects but when I click on them they don’t do anything.
maybe Im missing something but I could not find a download link to download this amazing script.
I downloaded the mootools.js file into a dir on my cpanel server. Then created a new file and think I put the above codes into the right place etc but when i try to load the page,it just sits there saying loading in the status bar, nothing happens, been waiting 9 minutes without error message.
any ideas please or am i missing a download link to download all files needed.
cheers
Dextor
Dextor: All of the necessary code is here. I’m guessing that your machine is having a problem connecting to GMail, or GMail is slow. The fact that it tries for a while instead of just dying means it’s attempting to get to Gmail. You may also want to change your username and password.
wow quick response, thanks David, can i pastbin my file so you can see if i have it setup right?
here is the pastebin.ca link
http://pastebin.ca/1570904
@Dextor: You need to make your code a complete page with, , etc. I also don’t see you bringing in the MooTools library.
This look better?
dreamweaver code color says the css / mootools part looks odd / wrong color like the syntax is out of place / incorrect
http://pastebin.ca/1570922
I get this error….
Cannot connect to imap: Certificate failure for imap.gmail.com: unable to get local issuer certificate: /C=US/O=Google Inc/CN=Google Internet Authority
change
{imap.gmail.com:993/ssl}
to
{imap.gmail.com:993/ssl/novalidate-cert}
How to solve the encoding problem’s???
Thank’s if you have an answer!
I get a bunch of garbage that looks like a SSL certificate after the first message and the body does not print on remaining emails. Any ideas?
–1248921015.4E4FF544.18054 Date: Wed, 29 Jul 2009 19:30:15 -0700 MIME-Version: 1.0 Content-Type: image/gif Content-Transfer-Encoding: base64 Content-Disposition: inline; filename=”email-vox-logo.gif” Content-ID: R0lGODlhawAtAOYAANcZIP////fR0v/8/Od1edgeJdopMPO7vd05P/309f739/GusONdYtccI/nf 4P76+uqFidkkKvzv79ghKPrk5dw0OvK2uNknLfjW2N9HTd5CR/fU1dsxOOd4fPvn5++go+Vqb+Nf ZN9ESvbJy/3x8uBPVNosMuyTluFVWuh6fuJaX9w3PfCoq+yQk/zs7d08Qudyd+6bnuVnbPXGyO+m qPvp6tsvNfrh4vXDxfTBw+RlaeFSV+ZtcemDhvfO0OBMUuuMj+h9geuNkeuLjt9KT/bMzeqIjPnc 3emAhO2Ym/CrrvjZ2uRiZ+2VmfO5u++jpt4/RfS+wPGxs+ZwdPKztuJXXO6eoQAAAAAAAAAAAAAA AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAACH5 BAAAAAAALAAAAABrAC0AAAf/gAGCg4QSAocJhIqDR4cbi5CCjYcCD5GQFI6XhJQem5+KAkgVAKWl L0Y3igMLIRGmAAUMT5aKChYpIrCmJiEnGJFOIBe7VTGJhCRNO7sGIFGglzcgu7sFHSSCTi/VsBVK gxswxN3VGR8KgyNE5aUGMYIDJ+TlPwLRijMG7bAIS0j8YMFYwiNgORs0AsRoYBAAAwooGhZ4gk9b gYYYMza0obFjC3wY6HUcSbIkRgugBugyybKlSwARPG2i8XKkiQw6CMDIgKDmyA6fNHRTIqDIkIwZ LByC0s4EhBmQHLQQGtBAEQE5mPErceCQinIT0pkCAiAAACAUyhkZFALjowBp/7tFEIJsk5IVAU8I ItGznAkJgjYwLHdAUNkMZwFkoNntxaAFDR0LatKNCAVILiA9mMIvpqAD7RbEW1kuhWGzZMn2aPeW xASDEAYh3qUjnaAHVHSYMDVBRYzMgxa2AzGIQbcSgz4ERHG6rHMY7WILMh7QhyAH1UIMGCSlr9wW tgMob1fk+kVYBRwIkrCPX4ZLKdqtGMS4XYVBLXZpQDYgvkEiNQwSRDvICSLELtIFUFBAP1ySXzvW BSDBYOUEMQhVpTQAjCAyZIQAYAE8gFc5ooXoHQK1jNCQDpdYwE8Pg2hVzgiCYLALAYMk0ZEK2wUA WTkcIANaKYUFoFJDQ1ySwP9r5dwnyHjdmNCjEejJ5MF5GrEwCGnVJGgccYLo2BCNl3RIniBxdQOU IN4BwMQgBJCEQI9WtJPedQZY+YpBUHyCAZbVWChICYQJIsAuJS5ZEg5o8hPCIJcJQk1DJW5yYJM9 ntCNAT2uBot6AQxJEoyCjDijIjhg9GZKhJ4awBHdgBkAKbzhZ5IKg3zVjgg9mtgQB8CBkkBE3Zgm CIamFKmiN4N0YFKfgizYDkqCsNAQApHiowCtu9jQIwS7hCWIf6YgAKdJ8wkSZztBDsJeQAWoUtED TLQDVQA2wiJDPBzBYoOAJr3HIT9UKALlrgFGM0AV/Kw5KywlprpLA7XUN9L/voL80A4Dg/Q4ALLl vBDsJkfxw6kgAJUyQTYBrLvLW/mS9MEgIqEHKgkM9Ciqo6A4AGg5OQiyrEPxtLdLExeSVEDCMXfZ LAAzT9dQwZukHBCORranZajlEMEdSQ6D2027AQgGgAEg+myQCJs8sJtBJwfgbAMgSlvNPRl3dEHC CvRbDbUBtApA2A1tCEkUGRUJGnMBKFAzLByj+XZDDRQZgJjVRO4jLA0cIUgCHBikVyQPNjSFIAoY ELWL/DgxiA9G89MARWjuaQ2ooO+i+Y/8YAwJuXDbxkOkZrZzgeE3cBll0KiDbEqCYu9i+WwEXuJy Q0WCqgCT/CAwMg2mwnKB/xEsG9nW2MiYXQ2KQgckMCQDZiSrILwHhACogwjQBAH8DxFFrwFIgK66 ATjBVWN0AZhUORgHiYMZ5ALhCcD5GhKBDwBwEzgIn+4e0xk9tQNrkGhaQwAnAe5hBAGVikQRiFWO OwUwdPyAwSA0RaJNcCMjGuAfAagzkgvogAUCeMQNBIALGPKjAjpkYTsaAAP+caYbEwBRJJ7gkypa sWGfGABTrsjFK0YgYZvwgQm7SEaWbA0U9SvjSGx3xQRF4wBstM8MoNOQEGSFJBdIggKiF5Ad+IAd DUFgRZYwwHIwIWFKMOKm4CGIHDCAQgFZgRCkaIE2VWMu23kABMaoH0ZVJGAfIIgdAGxAALwNQgFW UGIpfnCC8g3CBTHQAbfQU4Ie3EsRD6CBCiAJAA0MQYqCqIERbmiKAoQghZ9cxCGAuIRPGCIKXRnZ JTywzBwcAh8kEAAOlJKtSAxRCjMQQF0qEggAOw== –1248921015.4E4FF544.18054 Date: Wed, 29 Jul 2009 19:30:15 -0700 MIME-Version: 1.0 Content-Type: image/gif Content-Transfer-Encoding: base64 Content-Disposition: inline; filename=”spacer.gif” Content-ID: R0lGODlhAQABAIAAAAAAAAAAACH5BAEAAAAALAAAAAABAAEAAAICRAEAOw== –1248921015.4E4FF544.18054–
Bob Snider : If the email has an attachment you will see that. The code in this place is just an idea to make a better code. Check the Imap functions at php.net . I’m still working in this, the idea is good but not complete.
hi this is superb script…
but i got some errors
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/ospreyan/public_html/kushal/mail.php on line 8
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection refused
can you help me in that
i used ur exact code. there is no error but half of the code is printed instead of the emails.
here is the code i took from u exactly. try to run it with ur username and password. does it run correct. change the username and password. thanks anybody.
Please correct your output stanza
$output.= ‘seen ? “read” : “unread”)’;
$output.= $overview[0]->subject;
$output.= $overview[0]->from;
$output.= ‘on’.$overview[0]->date;
If you are getting a lot of weird characters in the message body (i.e. =0D or =0A) then you have to check the encoding with imap_fetchstructure() and then decode it properly. You only need to decode for Quoted-Printable and Base64, however. Here is my code for this:
Note that I use UIDs, so this will have to be changed for however you are doing it.
@deepak: Fatal error: Call to undefined function imap_open() in C:\wamp\www\swaticlass\imapp.php
Im geeting this errior could nyone solve it
Cery cool script !!! But I notice that is very very slow to load. Why ?
hi,
I got this error..Call to undefined function imap_open()
I enabled “extension=php_imap.dll” in php.ini
stil I have the same problem
Hi eicky,
Have you tried restart your server after you enabled the extension?
@chris: how to blocked the 993 port on win xp…i had been added the port on exception, but still not working…
thanks…!!
@David Walsh: one more…
i used wamp on my server with localhost,…
and the error is stil “couldn’t not open stream”…
my server spec is : php. 5.2 and apache 2
if i replaced the php_imap.dll with the new one from php.5.3..the error is “Call to undefined function imap_open()”..even the php_imap extension is still enable on php.ini..
can u explain how to setting the php.ini or the firewall setup…please mail me…ty..
hi this is superb script…
but i got some errors
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/ospreyan/public_html/kushal/mail.php on line 8
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection refused
can you help me in that
If you are using localhost and having problems, look into XAMPP instead of WAMP
this is very good idea…..
Can you help me to take the html code of each mail….. and also need to display only the unreaded messages ….. please help me sir…..
It doesn’t seem to work with Danish characters, any suggestions?
its working well for me .. i m using this with my own server not with gmail. thanks for this great effort…..
great, thank you!
hi david,
can we use jquery instead of using mootools javascript.
if u have problem with certificate, add /novalidate-cert
imap.gmail.com:993/imap/ssl/novalidate-cert
if you cant connect then its the port 993 which is blocked (outbound blocking)
i have great ideas to use this, muahahahaaha(evil laughter)
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in \\hmfsw\web\dtcwin071\6700.com.ar\public_html\ver\mail.php on line 8
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Host unreachable the php say’s that, i be read about this and say’s “993 port is locked by firewall” what i can do?
@deepak: your PHP implementation probably requires <?php, not just <? on the first line
@Bob Snider: Its an atachment – you need to read up on mime and how to parse email
david, amazing! can it retrieve also the sent mails? or even user generated folders? i will be studying this IMAP thing.. thanks for the great idea! feed on!
@David Walsh: i seem to be having the same problem as nimz where i’m getting “Cannot connect to Gmail: Too many login failures” even though my accounts are perfectly fine?
Thank you, David, very useful stuff.
Something strange in folder structure, where does Google store spam…
Hi David,
Just wanted to know if we could also acquire the attachments with the email as well.
If this is possible please let me know..
In advance,
Thanks for your help..
hey david can you please post the whole script here at your blog, because i think your codes are incomplete and i cannot see sliding animation in my page…
Thanks
Wel the script is 100% working, but i want to know how to paginate the inbox to multiple pages, can anybody tell me?
Interesting technique, and I like how you included the accordian to make things more interesting.
Do we really need another PHP mail app though? :) Integration possibilities are endless though of course.
Excellent lesson. I have a question. Im using it to retreive an overview of my gmail into my private intranet. Works great but I would like to make every subject link back to the gmail account directly.. i know i need to get some kind of ID for each mail then redirect to mail.google.com/#inbox or something.. any ideas?
Thanks david really helpfull
@Iván: Ivan its prob with your config file…go to php.ini and enable (extension_imap blah blah)…and you are done…
Hi,
First of: Great code example, gets a man up and running in no time! Big thanks.
Second: have you been folowing the release of gMail IMAP + oAuth?
That opens the door to all sorts of weird and wonderful opertunities… anything from “Open your gmail from my website” to a Gmail Facebook Tab (because, lets be honest, the current implementations suck :-)
greetz,
P.
I saved the php code in a TextWrangler document and uploaded it, but on my localhost (MAMP with an IMAP code edit) that delivers a blank page and on x10hosting, I get the following:
Parse error: syntax error, unexpected $end in /home/r5radar/public_html/walsh.php on line 70
(I have a thorough understanding of HTML, have some experience with XML, and am just learning php)
How should the accordion, CSS and php codes be saved? I just want to get this awesome code to work before I embed it in an actual page or design…
@eicky: i think you need to copy the dll into your php directory as well…
@Dan: Please help me Dan
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in C:\xampp\htdocs\POC\test.php on line 24
Cannot connect to Gmail: Can’t open mailbox {imap.gmail.com:993/imap/ssl}INBOX: invalid remote specification
it was a firewall is connecting 993.
Still i am getting above error
Regards,
Naresh
Hi all,
David ur script really nice, But i want mail download from pop3, if u have any idea for that, Please help me…
Thanks & Regards,
Vinoth S
I’m download the mail from local(means my company) mail server, and the values are spilit and stored into DB, But its a POP amail server, so if u have a idea or something please help me….
How modify code only view 5 last new mail receive on gmail inbox?
Hi, i m receiveing this
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/uppstu5/public_html/test_gmail.php on line 8
Cannot connect to Gmail: Too many login failures
Plz help me
how to do this for Yahoo Mail??
thanks :)
This is a nice and useful script but “how the hell do I copy that code??”
Just line by line? That’s “scary”!! :p
Can someone link a zip with the good files.
i got the same message.can somebody help
Hi
I have getting the problem that my js file is not working.
Some error is displaying that
window.addEvent is not a function
.I tried but i can’t get any solution so i want to ask that how to resolve this error.
@bob snider
1248921015.4E4FF544.18054 Date: Wed, 29 Jul 2009 19:30:15 -0700 MIME-Version: 1.0 Content-Type: image/gif Content-Transfer-Encoding: —>base64 Content-Disposition<—- inline
its encrypted with Base 64 strings, all one needs to do is add a php base64 decode function to render it to text or html. Dav, images should show when decrypted assuming that the correct image html attribute is executed from the browser .
For complete image rendering use urldecode or preg_replace (%22, 3D%22,etc to ” and = etc) after base64 decode scripting, just rendered an image on a html editor with an example gmail source code.
problem example I had after decoding base64:
src=”3D%22http://www.example.com/images/email_example-head1.gif%22
Dear David
Thanks for this code, I found it really useful. However when I send a mail with an attachment it goes all wrong…
Errr….what is that MooTools code?
Btw I use the php code only. On the browser it shows
Fatal error: Maximum execution time of 60 seconds exceeded in C:\Program Files\xampp\htdocs\display.php on line 36
what’s wrong?
you have way too many e-mails so your script exceeds maximum time defined in php.ini trying to download them, strict your imap_search($inbox,’ALL’); to lets say imap_search($inbox,’UNSEEN’); and try again ;)
which PHP imap script should be the best one for me to install?
http://www.phpkode.com/scripts/tag/imap/ PHP IMAP based client
i’ve tried the mootools simple accordion code above and it didn’t work. Can you help me how to use it inside php? I put the script inside head tag, is it true? something like this..
save http://davidwalsh.name/dw-content/mootools-1.2.2.js in your script’s directory
+ link it in your head section OFC :)
Hi David, I have used you code for fetching gmail inbox in my php application. But i am facing a problem. when I executing the file in browser it taking a very long time to execute. please give me suggestion. Thanks
hi we are getting this error by above code
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX
can you tell me solution for this
This was working for me at one point, and now I get the following error.
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /hermes/bosweb/web090/b906/ipw.175gcom1/public_html/awaitingreply.php on line 8
Cannot connect to Gmail: Too many login failures
i tried changing my gmail password, etc. curious how you would reset gmail or grant permission to this application.
Me too. Would love to get this sorted
I did have this problem too but in my case the solution was wrong password, I confused passwords with another account and it seemed correct ;X
Hi I am using your gmail code. But I am getting error. See below
http://knocktechsolutions.com/easy/gmail.php
How to fix this error.
Karthik
Couldn’t open stream {pop.gmail.com:995/pop3/ssl}INBOX in /home/knock123/public_html/easy/gmail.php on line 22
Cannot connect to Gmail: Certificate failure for pop.gmail.com: unable to get local issuer certificate: /C=US/O=Google Inc/CN=Google Internet Authority
———————-
pop.gmail.com:995/pop3/ssl ?
try using imap {imap.gmail.com:993/imap/ssl}INBOX
Your self-portrait made me think I was hallucinating at 230 am… I didn’t realize it had a mouseover effect but I kept seeing tiny little dots flying around every so often.
how to get X-GM-THRID in gmail via imap php?
http://code.google.com/intl/ru-RU/apis/gmail/imap/
Tried this script and it does not work anymore.
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/mtharmal/pesiplex/condoclicks/test/gmail.php on line 9
Cannot connect to Gmail: Certificate failure for imap.gmail.com: unable to get local issuer certificate: /C=US/O=Google Inc/CN=Google Internet Authority
Notice: Unknown: Certificate failure for imap.gmail.com: unable to get local issuer certificate: /C=US/O=Google Inc/CN=Google Internet Authority (errflg=2) in Unknown on line 0
Looks like SSL might be an issue here…
Nikke
I am hosting the example to my site http://www.mysite.com and its not working saying that “Connection failed to open stream”
But when i tried to run localhost machine its working fine
Can anybody give a fine solution to the problem as many are facing the same problem.
That was what I was looking for. I will right now try it. Thanks for sharing.
I am getting the :
Cannot connect to Gmail: Certificate failure for imap.gmail.com: unable to get local issuer certificate: /C=US/O=Google Inc/CN=Google Internet Authority
Did someone find the fix?
thx in advance.
for all the people having issues with certificate… I found the solution.
I set novalidate-cert in my servceflags and problem solved!
[code]
$config[‘service_flags’] = ‘/imap/ssl/novalidate-cert’;
[/code]
So in this piece of code it’s:
[code]
$hostname = ‘{imap.gmail.com:993/imap/ssl/novalidate-cert’}INBOX’;
[/code]
It works great. Now I need to get the attachment out of the email and save it on the server. This seems to be a little bit more difficult.
I’m wondering as to how can I use this code with processmaker so that the case is automatically created the moment processmaker receives mail with specific subject.
Thanx
You really should moo that PHP trash to node.js. JavaScript is now officially a trendy language. PHP is the worst language ever conceived.
I meant “move” instead of “moo”…
why i can’t open this message body:
/* output the email body */
$output.= ''.$message.'';
}
are someone here knows about mootools-1.2.2.js ?
i has link it like this but no reaction
please i need little help here..
why i can’t open this message body:
/* output the email body */ $output.= '
'.$message.'
'; }
are someone here knows about mootools-1.2.2.js ?
i has link it like this
but no reaction please i need little help here..
Great script, but i couldn’t get it to work, third time trying and I’m getting this error a long load time,
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/xxxxxxx/public_html/phpmail/display.php on line 28
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection timed out
Hi,
This is very nice to see, but I also can’ t get it work with the mootools.
Are you willing to upload a working zip here so we can see what goes wrong ?
That would help a lot !!
Thanks.
MatBoy
Hi,
This script is great, but I also have the mootools issue which makes that the dropdowns are not working.
Are you willing to upload a simple working example so we can see what goes wrong as more people have this ?
Keep up the good work!
Thanks,
MatBoy
PLEASE I NEED HELP I GET:
Parse error: syntax error, unexpected $end in /homepages/26/d94605010/htdocs/lz/writecodeonline.com/php/index.php(98) : eval()’d code on line 34
Parse error: syntax error, unexpected $end in /homepages/26/d94605010/htdocs/lz/writecodeonline.com/php/index.php(98) : eval()’d code on line 34
PLEASE I NEED HELP!
this worked right out of the box!
david walsh has helped me yet again…. the first time was his rather brilliant interface to tinyurl!
@deepak
try <?php instd of <?
@deepak try
<?php
instead of<?
How to add email forwarding to other email address using your code.
All I get when I run the script is:
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /Applications/XAMPP/xamppfiles/htdocs/mail.php on line 24
Cannot connect to Gmail: Can’t open mailbox {imap.gmail.com:993/imap/ssl}INBOX: invalid remote specification
I’m using localhost. Would that be the problem?
Can someone help me?
Thanks.
hi,
great code, but i get
Maximum execution time of 30 seconds exceeded
Can we increase a time. I have 3700 mail in my inbox,
Hi David,
Is there any way to fetch mail from sent box as well as inbox.
I have use your sample code to retrieve the mail from Gmail and it works awesome.But now i want to implement the system where i can search for particular mail id and it will be presented to me.and this email id might come from the inbox as well as the Sent mail.
I implemented the search also but all the time i need to see the mails in inbox as well as sent items,
Hi Sid,
To get the emails from sent items in gmail, use the host name like this.
{imap.gmail.com:993/imap/ssl}[Gmail]/Sent Mail
This should work.
Thank,
Muhammad
I tried this code wamp server local system is working perfectly but hosting this script it show error like
Can’t connect to gmail-pop.l.google.com,995: Connection refused
Did someone find the fix?
Thank u
Hello David,
I Used Your Script and with detail reading about php Imap m able to use it accordingly
but m still unable to fetch the spam mail
any idea how to do it ?
Whenever i try to connect with gmail server it give me fatel error !
i had try everthing to get my mails from gmail !
please help me to solve this mistake
this is my code ………
Maximum execution time of 30 seconds exceeded in C:\xampp\htdocs\mymail\mail.php on line 49
Thank you very much Dave!!! I really appreciate your effort in sharing your knowledge!!!
Hi tried running your script and I get this error. Kindly help I have tried everything and nothing seems to work.
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/ssl/novalidate-cert} in/home/spifiel1/public_html/email.php on line 8
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection timed out
hi,
I wuld like to connnect to the hosting email of my website. I am able to connect to gmail but i want to connect to my website email. I have cpanel and port used is 110. getting error on imap open function. imap host i am using
for anyone getting errors for imap. if you are on a mac and using mamp. mamp does not have imap in it yet. cehck this page http://forum.mamp.info/viewtopic.php?f=4&t=9422
Hi David Walsh
I am getting this error..
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/coimbato/public_html/cp/mailling.php on line 31
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection refused
i uploaded it and tested..but receiving the same…
Can’t sure if it related to SELinux, but i had selinux enabled and got following message:
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /var/www/html/imap.php on line 34
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Permission denied
after disable related selinux permission by
setsebool -P httpd_can_network_connect=1
script work well
(googled said that /var/log/message or /var/log/httpd/error_log should had selinux warning, but i can’t find it at CentOS6 x64)
Fatal error: Call to undefined function imap_open() in C:\wamp\www\prog\proj.php on line 33
i am using wamp server,any suggestion how to remove it??pls answer asap..
title . '';
echo 'You have ' . $xml->fullcount . ' ' . $xml->tagline . '';
foreach ($xml->entry as $child) {
echo '' . $child->title . '';
echo '' . $child->author->name . '<' . $child->author->email . '>';
echo '' . $child->modified . '';
echo '' . $child->summary . ' link['href'] . '" target="_blank">Read more.. .';
}
?>
Very useful mail-handling script, thank you.
I would like to make a system similar to that outlook. could you help me? your script is not working well.
Hi,
For those who hav got a “invalid remote …” error whith MAMP (Mac computer) trying to connect to a SSL IMAP server as Gmail, I have found the solution here, and… it really works for me :
http://www.vargatron.com/2009/03/imap-ssl-with-mamp/comment-page-1/#comment-152
hey give me ful code :( i dont get it correctly…how i include mootools???
Hi Shareef
You can see the example code of david walsh that i used.
example code of david walsh by jorge caraballo
It works perfect in my own domain and i share it. You can try it using your own mail server settings.
Good luck.
Best Regards.
David,
While i am using this code some of the mails are skipped.Rest of the mails are downloaded as well.Any suggestion…
Hello everyone,
I am getting this error. From the above posts i tried to rectify, by changing INBOX to SENT MAIL and so on..but still giving me the same error. Does anyone else has face the same problem. if so please do share with me how to rectify it.
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in C:\xampp\htdocs\email\index1.php on line 32
Cannot connect to yourdomain.com: Too many login failures
Fatal error: Maximum execution time of 60 seconds exceeded in C:\xampp\htdocs\email\index1.php on line 51
getting this error whenever i try to run it..
Hi,
Is there any way to figure out the size of the gmail account using API, prior to downloading the emails?
Thanks,
-Mark
Wow, i’ve been looking for such simple code for long time. And your live demo made me believe again, that accessing GMail via PHP can actually work. :-)
I am wondering if there are any special requirements (php-imap client version or so) since I never get connected with imap_open on my environment. all ports opened. latest php release.
wbr
Jan
Thanks, this was most helpful !
I am trying to run this script on my server but it shows nothing can you send me the original script please
Hi David,
I am getting numbers of mails but it didn’t display mails and throwing below error
“Warning: imap_fetchbody() [function.imap-fetchbody]: Bad message number line 24”
I can’t get why its throwing this error?
Hi David,
I am getting number of mails but didn’t get any subject or other stuff even it throws below Warning
Warning: imap_fetchbody() [function.imap-fetchbody]: Bad message number on line 24
and below is my code
$hostname = “{imap.gmail.com:993/imap/ssl}INBOX”;
$username = “username”;
$password = “password”;
$inbox = imap_open($hostname,$username,$password);
$emails = imap_search($inbox,’ALL’);
if($emails)
{
$output = ”;
rsort($emails);
foreach($emails as $email_numbers)
{
echo $email_numbers;
$overview = imap_fetch_overview($inbox,$email_number,0);
$message = imap_fetchbody($inbox,$email_number,2);
$output .= ”;
$output .= ”.$overview[0]->subject.”;
$output .= ”.$overview[0]->from.”;
/* $output .= ”.$overview[0]->date.”;*/
$output .= ”;
}
echo $output;
}
imap_close($inbox);
hi David,
i have used ur code. But it prints these following php lines instead of output:
##
I hope I will get quick response. Thanks in advance.
can any one give me code for the following program using IMAP—–>
A GUI in which username and password along with the message content to be searched is asked and you have to dispaly message content of user who is logging currently to gmail and whose search message content is matched wt messages in inbox as well as in spam folder if any plz help..???
I have recently found a class on this site http://thetutlage.com/post=TUT182 , seems nice and promising ..
i am getting this error can any one help me out ( Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,995: Network is unreachable ). i have changed the port number to 993 still it isn’t working.
please its an request
Please help me
How to change the UNSEEN message to SEEN message.
Kindly give me a solution
I also tried something but im getting error something like this
Notice: Unknown: Can’t connect to gmail-imap.l.google.com,143: Timed out (errflg=2) in Unknown on line 0
Got a solution thanks.
your a hero…… seriously this has saved me hours!!!!
I am running it on localhost. on vertrigo server. but this error occured.
Fatal error: Call to undefined function imap_open() in C:\Program Files (x86)\VertrigoServ\www\atmail\gmail.php on line 35
can anyone help me in this??
How i can run this server. please tell me steps
Really nice, you save me a lot of time.
I managed to get the script to pull in the email messages but I cannot seem to expand the dropdowns. I am using the same mootools. Any way we can get a working example? thank you
Hey
am getting problem
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/realvalu/public_html/mail/mail.php on line 59
Cannot connect to Gmail: Too many login failures
Hi guys! Iam wondering how to mark the message as READ. Any tip?
Thanks!
Too good. You helped me alooooooooooot
Very good script and briliant
liked it a lot
is there any possibility to see/read deleted email from trash folder?
i have some important email deleted and by mistakes i click on “delete forever”.
but there are very important email also gone.
any idea to read that emails from the account.
(i am using gmail).
thank you in advance if anyone can help me.
Try to use this function to avoid encoding errors when displaying titles
Try to use this function to avoid encoding problem displayed like ?ISO-8859-1?Q?, etc.
To those who have reported a “Too many login failures” error:
1. Open your gmail account, go to settings -> Forwarding and POP, and make sure IMAP is enabled.
2. Your account might need a captcha to login due to multiple failed login attempts. To solve this, login to your gmail account and visit this link: https://accounts.google.com/UnlockCaptcha
3. Lastly, change
imap_last_error()
toprint_r(imap_errors())
. This shows the exact error.Hey does anyone know of a free webhost who doesnt block port 993. Thanks
i m facing this problem….
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}All in /home/floodair/public_html/own_email.php on line 8
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection timed out
while working fine on localhost WAMP server. I m using it here….
http://www.floodair.com/own_email.php
I m facing this error on my live site while working fine on localhost WAMP server… any idea??
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}All in /home/floodair/public_html/own_email.php on line 8
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection timed out
My page is on:
http://www.floodair.com/own_email.php
Very usefull. The script however needs some modification. The headers need to be encoded. You can do like this
http://php.net/manual/de/function.imap-mime-header-decode.php
Help! I dont understand how to execute this php code. Im not too tech savy. Did you upload it to a site you have ftp access to and then hit the file location with your browser?
did you put it into some other software to run it?
I dont know how to implement this
@stephex i have developed the combined code for fetching the gmail and also integrated it with attachment reader
Contact me for details
@david
Cool script definitely. i made some changes acc to requirement
the problem is
the code runs smooth on localhost
but it gives cannot cannot to gmail error when i upload it on my web server
I read through the thread
found out others also had the same problem
can anybody share the solution ?
Thanks
Ok so now i got it worked
thr was a problem from the hosting side
now i am facing problem in deleting the mail
seems like expunge doesn’t work on inbox any more
any clue to how get that work ?
It does not work with my GMail account… But works great with my Microsoft Exchange account ! Thank you David Walsh ! Now I can automate my announces ! :)
I got error like this
Cannot connect to Gmail: Certificate failure for imap.gmail.com: unable to get local issuer certificate: /C=US/O=Google Inc/CN=Google Internet Authority
Why?? kindly need your advise to solve it.
I am using Hostgator to hosting it
Rgds
/PH
Great article
(I would recommend using: quoted_printable_decode and HTML purity on the message output)
Once in a while I get some encoded text in the subject line. For example…
Congrats! You’re invited to start selling for free, Paul.
was returned as…
=?UTF-8?Q?Congrats!=20You=E2=80=99re=20invited=20to=20start=20selling=20for=20free,=20Paul.?=
I’ve tried using the following with various combinations of parameters. Some returned the original input text while others returned nothing either with or without an error.
iconv_mime_decode($overview[0]->subject, 0, “ISO8859-1”)
echo iconv(“utf-8”, “windows-1250//TRANSLIT”, $overview[0]->subject)
utf8_decode(imap_utf8($overview[0]->subject))
The problem appears to be encoding, but I’m at a loss as to the solution. Any suggestions???
Hi
I am able to retrieve the mail using your script but only the subject and when I click the subject it is not displaying the email body. How can view the mail message as well.
@mukesh:
I know that if you change the 2 to 1 in the line below, you’ll be able to see all messages. I can’t get the accordion to work properly either.
$message = imap_fetchbody($inbox,$email_number,2);
Just wanted to say thanks, useful code. If you’re still reading the comments, you should use strip_tags to delete eventual , tags and keep only useful tags.
Awesome code dude !!!
Actually the above code fine on my local machine but when i put it on server it shows “The connection was reset”…
Any solution…what might be the cause..php imap is installed on it also
On my server it doesn’t right! What is wrong???
Does anyone have this working properly? I use David’s demo email info and get an error: Cannot connect to Gmail: Too many login failures
I receive this on one of my gmail accounts, but not on the other. On the account that works, the accordion does not work. All emails are open entirely.
SOLUTION: Google now has a 2-step verification process for added security as an option. If you have 2-step verification set to ON, you’ll need to go to Account>Security>2-step verification>Edit Then log into your account again. and set up the password for an application. Use this password in the php file instead of you Gmail account password.
Message output encoding is definitely a problem.
I guess I was looking for something like Horde or Squirrelmail without the delete or send functions.
Any ideas???
Change:
to fix the encoding issue and allow images to show up.
3 years later and this post is still valuable! First I’d like to thank with all the help!
Second, I am having a odd issue with this that I could not figure out. Hoping you can help!
So my function works perfectly — I can forward an email from an account on Outlook (account#1) to my gmail and read it no problem through this function.
The issue is when I copy/paste the SAME email (the .msg file) from Outlook (account#1) to my Outlook Gmail with IMAP setup (account#2) the function returns nothing for the body. The rest (seen, subject, from, date) is seen.
The only difference is that my first attempt, my gmail account is the only one that receives this email, where as when I copy/paste the email it is sent to 100 plus people. I would hope this would not break it?
Please let me know if you have any idea.
Hey David ,
Thanks for this nice explanation and its working fine for me .
Now I am working on exploding public certificate from emails message, do you have any idea about this .
Your reply is highly appreciated .
Thanks
Hi nice post with the help of this post i managed to make the gmail inbox reader code.
Now i can read my inbox in a php page.
http://zkforum.com/retrieve-your-gmail-emails-using-php-and-imap/
With this code you can correct the Subject displaying
So, change the PHP var:
Hello, for gmail dosnt work, someome works fine with gmail????.
And for hotmail??
Thanks.
Thanks for this great tutorial. This is going to be a critical piece for a system I am building to track inbound calls to a 1-800 number I have. I receive an e-mail for each incoming call and have been basically manually inputting this information into a simple database. Now, with this, I can setup a cron job to get and parse the e-mails straight into the database. This is going to be a huge time saver for us.
Thanks again.
i m a begginer…i m using localhost.Can anyone tell me all the setups required before running this code. i m getting this error
imap_open(): Couldn’t open stream {localhost:143/imap/ssl}INBOX in C:\New folder\htdocs\facebook\email.php on line 10
Cannot connect to Gmail: Can’t connect to dell,143: Refused
Notice: Unknown: Can’t connect to dell,143: Refused (errflg=1) in Unknown on line 0
Notice: Unknown: Can’t connect to dell,143: Refused (errflg=2) in Unknown on line 0
Hi anubhav. Do you have an email server running in your localhost already, using imap port 143?
heelo some error slove but 1 left.
please help me.
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /home/cottonha/public_html/mail/index.php on line 21
Cannot connect to Gmail: Can’t connect to gmail-imap.l.google.com,993: Connection timed out
Hello dharmesh
I have never been able to connect to gmail. I got the same error like you.
So, i redirect the emails from gmail to another email server (not gmail).
I don’t have any issue with that other email server . I can connect without any problem.
By the way, i use bluehost services for this (email server)
Best regards.
@David
I am getting the below error can you please suggest something
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /var/www/html/paid/imap2.php on line 11
Cannot connect to Gmail: No such host as imap.gmail.com
Notice: Unknown: No such host as imap.gmail.com (errflg=2) in Unknown on line 0
I have used the exact php code from your post.
if you are having trouble with the body of your message not showing then their is a simple solution : just find
this line in your code and replace 2 with 1 just like
Now your email body contents showing you !! Fixed
Instead of disabling the security system with
{imap.gmail.com:993/ssl/novalidate-cert}
you should ADD the certificate to your trusted ones!
look here, how to do it:
http://blog.donovan-jimenez.com/2011/03/adding-new-trusted-certificate-on.html
I used this article’s example to scrobble together a 2-way email communication script where the web app is the middleman.
Cool thing about this simple example script is that the incoming emails are marked as ‘read’ or ‘unread’- and that gives me reason to forward only the unread messages to the right people. Look ma–No logic!
Save my hide again, Dave- thanks!
Chinese zip and csv files not working. What are changes have to do ?
This still works? I mean Google is kinda preventing hacking when using this technique :/
Hola Martín Cisneros. Supongo que hablas español por tu nombre. Por eso escribo en español. Yo estuve intentandolo con Gmail pero no me funcionó. Ya no recuerdo cual era el error, pero con Gmail no me funcionó. Lo que si recuerdo es que parecía ser un tema de seguridad con Gmail lo que me impedía conectarme a Gmail y obtener los emails. Lo que hice para mi aplicación fue utilizar un servidor de correos distinto, uno contratado por mi que vino incluido con el servicio de hosting que contraté. Con dicho servidor de correo, funcionó perfecto. Mi aplicación funciona perfecto. Se conecta, obtiene los emails, etc. Saludos.
How can you check or scan then save locally if there is an attachment on each email.
hello,
I get this error
Warning: imap_open() [function.imap-open]: Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in /public_html/gmail.php on line 176
Cannot connect to Gmail: Certificate failure for imap.gmail.com: unable to get local issuer certificate: /C=US/O=GeoTrust Inc./CN=GeoTrust Global CA
I used your script and i get this error : Cannot connect to Gmail: [CLOSED] IMAP connection broken (server response).
I have configured IMAP(for my email adress), and added the imap extension.
It simply dont wanna work for me !! Please HELP
I used your script and i get this error : Cannot connect to Gmail: [CLOSED] IMAP connection broken (server response).
I have configured your email address oly am working in my local
I am getting this error:
I am a beginner and I’m facing this problem. Please help me.
Warning: imap_open(): Couldn’t open stream {imap.gmail.com:993/imap/ssl}INBOX in C:\xampp\htdocs\EmailTesting\Main.php on line 27
Cannot connect to Gmail: Host not found (#11002): imap.gmail.com
Notice: Unknown: Host not found (#11002): imap.gmail.com (errflg=2) in Unknown on line 0
I get an error:
TLS/SSL failure for imap.gmail.com: SSL negotiation failed
i an error that points to the subject:
Notice: Undefined property: stdClass::$subject in C:\wamp\www\ManagementReport\Tracking\access.normal\E-mail\Email_inbox.php on line 39
Cannot connect to Gmail: Certificate failure for imap.gmail.com: Unexpected SSPI or certificate error 80096004
Hello, I am facing error while implementing your code:
I get this error. I am on GoDaddy shared hosting. I changed to php 5.6 and the IMAP was already checked.
Any idea of what the problem would be?
Thanks,
Rich
We need to do few things from the gmail side while connecting
1. Login to your gmail account, Under Settings -> Forwarding and POP/IMAP -> Enable Imap.
2. Go to https://www.google.com/settings/security/lesssecureapps and select “Turn On”
3. Go to: https://accounts.google.com/b/0/DisplayUnlockCaptcha and enable access.
Hi David,
A very useful article.
Needed you guidance for a bit complex issue I’m facing.
I’m trying to migrate my existing system to GSuite for which I’m making the up-gradation.
I’m using a code-igniter environment whereby IMAP is being used as library. I’m using this to make connection
Using this I’m facing a
php_network_getaddresses
error as the Host name ( {imap.gmail.com:993/imap/ssl}INBOX’) is not getting resolved.Any kind help will be highly appreciable.
Thanks
You’re a genius ;)
Hi,
I’m receiving this error in the code-igniter environment as I’m trying to connect using IMAP library.
The code runs excellent on dry run but when integrated into I’m receiving this error.
Any help / suggestion will be very greatful.
Thanks.
Nalin.
Modifying a little bit of this code fragment, I managed to decode some messages, but those coded to 7bit nothing, are still irregular.
Hi@carlos,I want to save the email attachment into a local file.so,pls give me the code for this.thank you
Better approach to view message would be changing
to this:
Hi guys ! I’m try to get email contents from gmail and store the data in my database . I have the code but I don’t know hot run it !
can someone help ?
am doing filtered mail
and i want to work on mail notification for over due task using below code but
not getting any mail notification any
there is some packages that can do this like
https://packagist.org/packages/ddeboer/imap
https://packagist.org/packages/clivern/imap
Hi David,
This is a very useful article.
I want to make reply mail for particular mail thread and also using filter. Please suggest.