Upload Photos to Flickr with PHP
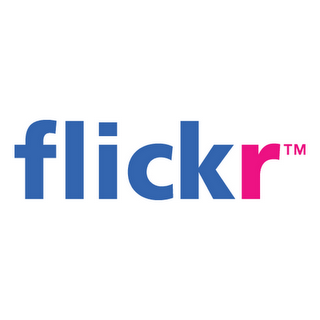
I have a bit of an obsession with uploading photos to different services thanks to Instagram. Instagram's iPhone app allows me to take photos and quickly filter them; once photo tinkering is complete, I can upload the photo to Instagram, Twitter, Facebook, and Flickr. This process made me wonder what it would take to upload photos to Flickr using PHP. This post details how you can authenticate and upload photos to Flickr using PHP with phpFlickr.
Step 1: Flickr Application Key Creation
Just as with every other API usage, you need to go to Flickr to sign up for an API key. Put together a good description and title, but one key is that you set the callback/redirect URL to the example page (or at least I did).
Step 2: Grab phpFlickr
phpFlickr is a suggested PHP library by Flickr. The library is not perfect (there seems to be a few long-standing redirect issues) but it does well as an all-purpose Flickr API interface.
Step 3: Configuring and Authentication
It's easiest to understand the process by looking at the code:
// Start the session since phpFlickr uses it but does not start it itself session_start(); // Require the phpFlickr API require_once('phpFlickr-3.1/phpFlickr.php'); // Create new phpFlickr object: new phpFlickr('[API Key]','[API Secret]') $flickr = new phpFlickr('[API KEY]','[API SECRET]', true); // Authenticate; need the "IF" statement or an infinite redirect will occur if(empty($_GET['frob'])) { $flickr->auth('write'); // redirects if none; write access to upload a photo } else { // Get the FROB token, refresh the page; without a refresh, there will be "Invalid FROB" error $flickr->auth_getToken($_GET['frob']); header('Location: flickr.php'); exit(); }
After starting the session and requiring the phpFlickr API library, an instance of phpFlickr
needs to be created, providing it the API key and API secret. With the instance created, an if
statement will be employed to react to a frob
parameter from Flickr. If no frob
is provided, the auth
method should be called, which either confirms authentication or redirects the user to the Flickr site for sign in. If a frob
is provided, the authentication token is set and the page needs to be refreshed (I'm not sure why the redirect needs to take place, but it was the only way to ensure authentication worked gracefully).
Step 4: Uploading a File
Uploading an image to Flickr is actually much easier with phpFlickr than authenticating. Uploading a file is as easy as one function call:
// Send an image sync_upload(photo, title, desc, tags) // The returned value is an ID which represents the photo $result = $flickr->sync_upload('logo.png', $_POST['title'], $_POST['description'], 'david walsh, php, mootools, dojo, javascript, css');
The sync_upload
method allows many parameters, but the image, title, description, and tags are the most prominent. There is also an async_upload
with may also be used.
phpFlickr also allows simple read access so that you may create a slideshow of photos, tags, etc., so don't think that phpFlickr is just for uploading!
My first attempt to create an independent PHP/Flickr script failed due to the need for OpenAuth. phpFlickr does a great job in managing the entire process, and the documentation is decent for getting started. I ran into a few problems with "too much redirection" (fixed by the "if" statement I added) and an "invalid frob" error (cured by the additional redirect) errors but beside those, phpFlickr is the right choice!
Excellent, thanks for the example, i was looking for this php code for a long time.
David I’m a newbie with PHP, and I was looking for this code for some time now. Thanks for the example.
It’d be a great help if you could guide me trough the Authentication Flow.
Thanks in advance :)
Thanks, David. I was searching for something similar for a while.
I have i TestWeb, it run on my local host Apache web server.
I use this example and i run my web at URL “localhost/Flickr_API-sample/upload.php”
I use right the key an secret that id received from my Flicker Account.
I get an error “Oops! Flickr can’t find a valid callback URL.
An external application has requested your login credentials and permission to perform certain actions on your behalf, but has failed to include all the required data.
You don’t really need to know what this means, except that you can’t use the application until this problem is fixed. (It’s a third-party problem, not a Flickr problem.)
There are lots of applications using the Flickr API. If you are curious about this, visit the Flickr Services page to see more examples of cool stuff. Otherwise, you might like to head to your home page…”
Please help me for this problem.
Thank very much.
Hey you need to set a callback url that is in the authentication flow in the edit app from where you created the app..
Not clear tutorial.
You have not given the content of all the related files..
Blankly you have given the upload code without any connection with the authorization..
What is the content of flickr.php??
What does this bit mean? $flickr->
I am getting error unexpected “&”
Very good.
Muchas Gracias David…..
Script genial y facil de entender.
Can you provide me a code of .net to upload files to flickr and return the picture id?
The Flickr API returned the following error: #95 – SSL is required
I’m also getting same Error. when uploading Images
May be upload images not sign in yahoo account?
I am looking solution for it.
And upload via URL?
You server not support SSL or Socket
i get this error when trying to upload
The Flickr API returned the following error: #98 – Invalid auth token
is there anything i miss ??
I receive the same error as Albar. Any solution or help?