Camera and Video Control with HTML5
The method for getting access to camera was initially navigator.getUserMedianavigator.mediaDevices.getUserMedia
.
Browser vendors have recently ruled that getUserMedia
should only work on https:
protocol. You'll need a SSL certificate for this API to work.
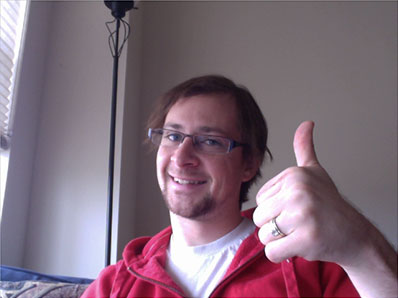
Client-side APIs on mobile and desktop devices are quickly providing the same APIs. Of course our mobile devices got access to some of these APIs first, but those APIs are slowly making their way to the desktop. One of those APIs is the getUserMedia API, providing developers access to the user's camera. Let me show you how to get simple camera access from within your browser!
The HTML
Please read my note about the HTML structure below:
<!-- Ideally these elements aren't created until it's confirmed that the client supports video/camera, but for the sake of illustrating the elements involved, they are created with markup (not JavaScript) --> <video id="video" width="640" height="480" autoplay></video> <button id="snap">Snap Photo</button> <canvas id="canvas" width="640" height="480"></canvas>
Each of these elements should be created once confirmation of camera support is confirmed, but for the sake of this tutorial, I wanted to show you what the elements look like with basic HTML. Do note that the dimensions we're working with are 640x480.
The JavaScript
Since the HTML elements above are already created, the JavaScript portion will look smaller than you think:
// Grab elements, create settings, etc. var video = document.getElementById('video'); // Get access to the camera! if(navigator.mediaDevices && navigator.mediaDevices.getUserMedia) { // Not adding `{ audio: true }` since we only want video now navigator.mediaDevices.getUserMedia({ video: true }).then(function(stream) { //video.src = window.URL.createObjectURL(stream); video.srcObject = stream; video.play(); }); } /* Legacy code below: getUserMedia else if(navigator.getUserMedia) { // Standard navigator.getUserMedia({ video: true }, function(stream) { video.src = stream; video.play(); }, errBack); } else if(navigator.webkitGetUserMedia) { // WebKit-prefixed navigator.webkitGetUserMedia({ video: true }, function(stream){ video.src = window.webkitURL.createObjectURL(stream); video.play(); }, errBack); } else if(navigator.mozGetUserMedia) { // Mozilla-prefixed navigator.mozGetUserMedia({ video: true }, function(stream){ video.srcObject = stream; video.play(); }, errBack); } */
Once it's been established that the browser supports navigator.mediaDevices.getUserMedia
, a simple method sets the video
element's src
to the user's live camera/webcam. Calling the play
method of the video then starts the element's live streaming video connection. That's all that's required to connect your camera to the browser!
Taking a photo is only marginally more difficult. Simply add a click listener to a generic button and and draw an image from video!
// Elements for taking the snapshot var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); var video = document.getElementById('video'); // Trigger photo take document.getElementById("snap").addEventListener("click", function() { context.drawImage(video, 0, 0, 640, 480); });
Of course you could add some sexy image filters and make a billion dollars...but I'll save that for another post. At minimum you could convert the canvas snapshot to an image though! I'll talk about canvas image filters in the future...
Being able to access the camera within the browser without using third party software is an incredible advancement. Paired with canvas and a bit of JavaScript, the camera has become quickly and easily accessible. Not only it the camera accessible, but since canvas is ultra-flexible, we'll be able to add sexy Instagram-style image filters in the future. For now, however, simply accessing the camera in our browser moves us miles ahead. Have fun taking images within your browser!
Post inspired by I see you getUserMedia; provided a great starting point for this post.
My friend, you are an inexhaustible source of source code, all more useful to each other! I follow you for a long time and I appreciate all the effort you put into your research and development! I say a big thank you!
How would I go about recording video for say about 20 seconds?
Hi Daivd,
This is really nice. we dont need to use flash anymore for this.
Thanks for your effort.
Hello David,
This article is great. I will use your tutorial to do many videos.
How do we do to take the video and send it to a web server?
Do you have any good tutorial to suggest?
Thank you very much
Eddy
Hi Daivd, This is really nice. we dont need to use flash anymore for this.
Thanks for your effort.
Hi Daivd, This is really nice. we dont need to use flash anymore for this.
Thanks for your effort.
It works with Chrome Version 23.0.1271.64 as well, no need for Canary.
and Opera 12.10. Thank you, David, for this
Chrome 22.0.1229.94 m and Opera 12.10. Thank you, David, for this post.
Can we add other feature liks adding some butter to apply the effects (color filter, fish-eye lens effect) to the snap photo?
can you demo this exactly with the audio element instead of video?
i’m trying to :
1 – access user’s microphone
2 – record something
3 – upon completion of audio recording have it display (with controls) under the recording area
4 – and here’s the hard part – record again (and again) and have them show up under each other with the ability to toggle/play/mute each track
5 – voila! an HTML5 audio multitracker!
6 – i understand that getting them to play in sync will require some work – but – a man can dream…!
Hi David, I want to know if it’s possible to access an external camera – i.e a broadcast quality camera? I’m looking to maybe develop some interactive products for live events, using broadcast camera(s) with maybe HTML5 /Canvas. Would be great to get your advice on this.
Paul.
@Paul
You should be able to access whatever camera the user has selected as the default in their operating system, be it a laptop internal camera, a FireWire or USB webcam, a digital video camera over FireWire, or a broadcast quality camera.
Perhaps the better questions you should be asking are, “Are there limits to the supported resolution and framerate?” and “Is it possible to provide for in-browser selection of one or more of multiple available cameras?”
As far as I can tell, 1) the limits are in the user’s hardware’s ability to process and render video at acceptable framerates and 2) it is not currently possible to provide for in-browser camera selection. So, as long as the OS recognizes your broadcast quality camera as a proper video input, you should be able to use it with this technology. My advice: try it. Get something like the camera you’ll be using, hook it up, and test it out on the demo page linked to in this article.
@Paul
I take #2 back: it looks like Chromium supports in-browser camera selection. I just tested it and it works perfectly in Chromium/Chrome as of 23.x
could these stream be sent to server or saved or record on local file?
At the very least, you could take screenshots at intervals and send them to the server with WebSockets or basic AJAX.
When i am trying to execute this code in my webpage it shows error in console i.e “Video capture error: 1” what is this error???
I got the same error. Please help me
Hy! I have webcam streaming site with red5 rtmp server. It possible stream audio and wideo to rtmp with html5?
@Dhananjay
It means permission error. Try making sure no other app is using the camera, and that your page is being served by a server like nodejs or whatever.
Hi David.
I do appreciate ur effort on source code and the beatiful demo!!!
the demo can be well performed in chrome version 23. as well as the source code in WAMP server.
i am now preparing my theis for a topic which may use ur source code. sure I will note in the theis the code is ur artwork.
best regard
Wang , a chinese students, one of ur beneficiary
Can we add some butter to this? I like popcorn and butter.
Hi David,
is it possible to access my mobile phone’s camera to stream the video on the page?
Hi David,
I have a similar question like Celu: Is is now possible (e.g. in webkit browsers) to take snapshops on mobile phones (without resorting to Phonegap)?
I recommend that you check out the web camera API, although web RTC should also be available (in Firefox and Chrome for Android). Neither API is available on other mobile browser though.
Hello friend,
I need your help as soon as possible.
When I click the button, it should initiate an event to capture an image with preview and transfer it to the server.
very lightweight and cool example !
One more vote for a response to Celu! Thanks. :D
I want to be able to take high-resolution images from the admin panel of the Magento eCommerce system. This is so I can avoid having to save pictures to disk, fathom out what I called them and then attach them to products. I think what you have here will do the job, massively streamlining the process.
However, how do I capture megapixels instead of VGA?
The backend form on Magento can be slow, ugly and lacking a preview, however, it will have to be functional. Can I declare the canvas object to be off-screen so that I have a simple ‘take photo’ button?
thanks. good code. this run
good stuff code is working fine
@Theodore have you found a way to save megapixels? I need the same thing for a CRM and HR system.
I found the way for saving megapixels. You don’t need to display your canvas to the end user, just setup your camera view
and set your canvas like . This way the canvas does not take a huge part of the screen, if you want to display the photo taken you can return the image object to another DIV after saving it through AJAX call.
The only problem is that you have to know your camera’s capabilities, because you can’t adjust the canvas size according to the camera’s capability automagically, or I just didn’t try enough.
Hi, I use js get the canvas iamge and send image to server like this:
Your browser does not support the HTML5 canvas tag.
But why the image’s clarity is very poor? use the native app can get picture very good…
Hello Jay,
This article is great. You have sent the video to a server. Can we start the video recording automatically when the page loads, or with a JS code like:
myVideoCamera.startRecording()
How do we do to take the video and send it to a web server?
Do you have any good tutorial to suggest?
I want to get video from two camera in one computer
Look at the section “Selecting a media source” in https://html5rocks.com/en/tutorials/getusermedia/intro/
How to stop webcam, thank you.
Same!! How to stop the web cam, the glowing light besides freaks the person out!
https://developer.mozilla.org/en-US/docs/Web/API/MediaStreamTrack/stop
If this does not work, try adding track.enabled = false as well
Hi thanx for the code.But if you can please suggest how we can use it in other browsers i-e firefox,internet explorer?
Firefox works! IE is still a little behind but the team has started a little work on
getUserMedia()
.I tried to capture video from webcam and upload it into server,
below URL working with local storage. I want to capture and upload to the server. can you give me your suggestion, I am stuck with almost a week.
Sample : http://html5-demos.appspot.com/static/getusermedia/record-user-webm.html
Hi,
How can I bind the display with a button click please? I don’t want the display starting at page open…
Thanks
google chrome not allow to access webcam
Please help me i want to develops video conference site please help me….
Hi,
Please help me .
How to close camera which is opened in browser? Can I use javascript or jquery ?Give your suggest and source code.
Thanks
Sir, i am getting error- HTTP “Content-Type” of “text/html” is not supported. Load of media resource http://localhost/project/ failed. I am using angular.js framework.
I also did the same experiment few months back . Its so great to get access the user’s hardware using front end code . Here is my experiment link http://99mobileworld.com/take-your-picture-using-your-webcam-by-web-rtc . I applied a bit css to make frames around it
How can i stop the camera taking feed continously using the getusermedia??
Why I am getting following error:
“Video capture error: 1 ”
Please suggest me.
it looks really good… I have a small question, will this work in IE or only for Chrome
I do not understand! i want a clean code! to copy pase and undertand
how can i use getusermedia api without using canvas?
I connected two USB webcam to my local computer ,I tried to connect both of them and display the image from both camera in same time . But no luck ,
Do you have any suggestion ?
I m getting nothing on chrome and my chrome version is 29.0.1547.57. please help me
How would it be possible to send the image to the server ? After the user takes the snapshot send it to the server ?
Check out this post:
http://davidwalsh.name/convert-canvas-image
Get the image data with the `Convert Canvas to an Image with JavaScript` code and then send that data via an AJAX POST call.
I used the same code u referred above..page shows as blank, and it is not even showing any error message like browser supports getusermedia or not
Do you know any solution for iOS? The demo works fine with Chrome on Android 4.2.2 but dosn’t work with either Chrome or Safari on iOS :(
hi, i add the html and the javascript part into one file, and open it in the web browser but nothing happens.
please explain how to make it work.
It’s explained in this article.
Hi David!
I followed your tutorial of “Camera and Video Control with html5”, but my
test didn’t work!
Everything works, but after to click on “Snap Photo”, nothing happens! No photo appears in the page.
Do you have an email I can send my codes?
Here is the link to my codes:
https://github.com/GugaSevero/html5_media
I’m waiting for your replay.
Thank you so much for your attention.
hello David
i want to open mobile camera in to php or html page from where i can take snap and upload it in mysql DB.
this same application is working in my laptop but when i open it in my phone its not working.
so can you plz send me a code how to do this
Hi David,
This code works beautifully for me on a pc, but I want to be able to use this same kind of method on an android tablet using the back camera. How do I do this??
Hi,
Thanks for the code. Actually I need to connect my PC with USB hub which connected with four cameras and I want to display what the camera captured. Please help me I need it as soon if you can
Hi Dave,
This is a great tutorial. Do you have an example on flipping the video horizontally, say onclick event? I have looked this up and all points to
scale(-1, 1);
but this doesn’t seem to work on when used just before thecontext.drawImage(videoElement, 0, 0, 640, 480);
nothing happens. Any suggestions?hey david, great is there any way to make this work on firefox in any way?
otherwise, is there a way to provide – so to say – a fallback image in case this is not working?
thanks in advance!
Updated to work in Firefox! :)
HTML5 captured video is just playing for 30 seconds although it has been captured for more than 2 mins.
Any thoughts???
Thanks for your help in Advance.
sir,
This coding works well. but i can’t get video from mobile. please mobile script.
Hi, this code is working very well on form load but i need it to be done on button click event.Any suggestion?
Thanks in advance!
Parabéns… Excelente conteúdo.
Hi Sir, I have captured video for more than 1 minute (without audio,because
getUserMedia
does not support audio with video) but when I am going to play the video it runs only for 32 seconds. Can You solve this problem.Thanks for your help in Advance.
Hi, it is a brilliant post, thanks a lot!!!
I’d like to make a question though…
I tried it and works fine for me in a computer browser, but does not on ipad, phone, etc, the browser does not even show the (url wants to use your camera allow or deny),
do I need to do that using a input with capture=camera yes or yes in these devices?
thanks in advance and thanks for the article!!
Hi.
This code is very good. I tested the code in a html site opened in a Android Cell Phone. I have one question about this, the code invokes the front camera of the cell. I needs to invoke the main camera. How can I get this? Any ideas?
Thanks a lot!
Ho João,
I have the same problem with the front camera of the cell. I also want to use the main camera. Did you found a solution aleady for this feature.
Thanks a lot!
Hi,
Excellent work! quite straightforward and clear code. I am trying to acquire images from user’s scanner. It is possible to do that starting from this code?
thnaks in advance!
Good job. Would you put how to do the same, but with a mobile camera? Thanks
Great job. Can we use the same code in jquery mobile if yes what will be change? I am new to web development. I really need it.
Thanks you david. But i want to ask something. I use this for accessing mobile camera. how to change the dafault camera? i need to use the main camera instead of rear camera. Thank you in advance.
Good day Magnificent Work!! but I’m having a hard time implementing your code can anyone give me link to download please..
Hi David, very nice example !!!
One question tho, is it possible to control the camera’s flash ? like toggle on/off, or possibly the camera’s focus point ?
Well this would have been so useful when I made my motion detector with getUserMedia :D http://motion-detector.nikhazy-dizajn.hu/
This is an easy and good tutorial, I had hard time to get it work back than.
I have no time to make it better, but it is so good to see you made article about getUserMedia. I bookmark this for the future.
Hello David. I just want to ask how can i change dynamically the resolution of snap photo in a scenario like responsive website?
how can i dynamically change resolution here? i already make my video and canvas responsive depending on the web browser size.
Hellow! Is there any way to capture video and audio (audio is very interested) on devices with iOS (iPhone, iPad)?
There are in fact no support nor GetUserMedia or flash. So…
Hi,
Reading mozilla docs I found a solution for firefox
do you have any idea how it work on safari ???
There seems to be some part missing, because the code from this page does not do anything. No errors, nothing. I tried to take the code from the demo page (that works when opened on your site) and open it standalone and it doesn’t work either. Is there a server side code or some external Javascript that supposed to initiate the video? Thanks.
Just checked this in Firefox Nightly and Chrome — what browser is this not working in?
It work find but only on your demo page. If I just take the code and the HTML above to a stand along HTML page – nothing works in any browser. “video.play()” never happens. That’s why I’m asking – is there any piece of code missing in your explanations above? Any external Javascript that you didn’t mention?
My bad – it does work in FF, but not in Chrome or IE.
Update: It looks like Chrome and IE need the page with this code to be loaded from a server, not an html in a file system. That’s very odd, it’s not documented anywhere.
Hello David,
Thanks for the article. Really simple and useful.
Like Cathy, Joao and Reynan have asked, how can we invoke the rear camera on mobile phones for this project?
Thank you very much.
Yes. Check out this link: http://www.html5rocks.com/en/tutorials/getusermedia/intro/
Can’t get it to work in Firefox 33.0.1..even with your code update. Any clues?
Is it possible to stop the video recording? A function of any sort? That would make this easier to use.
Hello everybody . Its save the captured image and turn it in binary.
Great!!! Tested and working.
Hey @David,
Thanks for writing such an amazing content.I got some errors like
navigator.webkitGetUserMedia
is undefined function.So i google about javascript webcam and found a solution i.e. WebcamJS plugin so i think why it should not be shared with other,so i just wrote a article and i hope that it will help others .
Here is the link http://mycodingtricks.com/javascript/webcam-api/
Can you post the styling for the button? I have everything working except the button.Thanks!
Awesome piece of work. i was looking for this and thought it would be a nightmare to achieve. This totally convinced me to proceed with my outstanding work.
Hello, nice tutorial, I want to know if there’s a way to know if the user denies share their camera, right now if that happens just left a blank image, but there’s no error or notification about it.
There’s a way to know if the access to the camera was denied or just ignored by user?
There is a command zoom for this code ? Scale -1, maybe.
Thanks.
and also it works on mozila fire fox 38.0.1.
thanks for this great tut.
Hey, nice features.
Could you also teach us how to crop fetched screenshot?
What can do with canon eos ?
Hi, It’s really good example. Thanks for sharing the information. It’s working fine on Chrome & FF but it’s not working on ipad safari & IE9+. Can you please provide solution for working it on ipad safari & IE9? Thanks in Advance!
Is there a way to share photos on instagram?
Hi ,
In Nexus6 with android version 6,the browser is not responding.Do you have any clue on this?
Thanks,
Srilatha.K
WOw, thank’s bro. Just copy and paste this magic code and voila! It’s capturing my face through my web cam.
Wow it’s work perfectly, I can take my picture on website :D
Hi ,
In Nexus5 with android version 5 its not working.
Thanks,
Ahesan
Hi,
I have a Sony Digital CamRecorder, output FIREWIRE to my PC. I can see the image properly in Firefox, but in Chrome. In Chrome, it appears as all black.
Any idea?
Thanks.
is there a way without ssl certificate?
Please tell me how to close camera or how to stop webcam
how to remove the recording symbol like red dot on the browser tab
Hi,
I am using the same thing, but i need image resolution 2560 x 1440.
But the image is getting stretched, and not clear.
So how to get clear image and prevent it from getting stretched.
Thanks
Swati
Hello, i write to ask if is possible make a photo of the video enought a botton but with a change of status. For example when the webcam recognize the face i have the status = “Found” and the webcam make a photo.
Hi. Im new on this and I may need some help. Do you have some file of source code or that’s all? It’s not working for me, so I don’t know if I’m skipping something. Thanks :)
Forget it, it’s working! Thanks
Thanks a lot! Is there any way to online view either front- or back-camera if on a cellphone? I’m gonna use live camera view as a background for my html-based social network-app “Brave”, and I believe that the users’d rather like to see their own faces than the ground in the background…
Thanks. It works fine!
Hello ,
This article is great. Now let’s see what’s on the Java Server side.
I am using tomcat anc I have the following exception according to the FILETYPE.
GRAVE: “Servlet.service()” pour la servlet UploadServlet a généré une exception
java.lang.IllegalArgumentException: Incorrect input type!
at javax.imageio.ImageReader.setInput(Unknown Source)
at com.sun.imageio.plugins.png.PNGImageReader.setInput(Unknown Source)
at javax.imageio.ImageReader.setInput(Unknown Source)
at com.delicieuse.UploadServlet.doPost(UploadServlet.java:92)
Here is my code: with the line that has the exception. If you are a Java developer or an HTML5 developer, you could tell be what is the FILE FORMAT. Please.
It seems to be a PNG encoded as a B64. But I can’t make it work.
public static String convertStreamToString(java.io.InputStream is) {
java.util.Scanner s = new java.util.Scanner(is);
return s.hasNext() ? s.next() : “”;
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Part file = request.getPart(“file”);
String filename = getFilename(file);
InputStream filecontent = file.getInputStream();
File outputFile = File.createTempFile(“Img”, “”, new File(“C:\\Temp\\”));
String fileContentStr = convertStreamToString(filecontent);
String base64Image = fileContentStr.split(“,”)[1];
byte[] imageBytes = javax.xml.bind.DatatypeConverter.parseBase64Binary(base64Image);
BufferedImage bigImage = ImageIO.read(new ByteArrayInputStream(imageBytes));
ImageReader reader = ImageIO.getImageReadersByMIMEType(“image/png”).next();
// Configure the input on the ImageReader
ImageInputStream bigInputStream = ImageIO.createImageInputStream(imageBytes);
// THIS LINE RETURNS AN ERROR
reader.setInput(new ByteArrayInputStream(imageBytes));
// Decode the image
BufferedImage image = reader.read(0);
ByteArrayOutputStream os = new ByteArrayOutputStream();
ImageIO.write(image, “jpeg”, os);
InputStream is = new ByteArrayInputStream(os.toByteArray());
ChannelTools.copy(is, outputFile);
long size = file.getSize();
String retMessage = “File ” + filename + ” successfully uploaded. “;
response.setContentType(“text/plain”);
response.setCharacterEncoding(“UTF-8”);
response.getWriter().write(retMessage);
}
Thank you very much
Hello ,
This article is great. Now let’s see what’s on the Java Server side.
I am using tomcat anc I have the following exception according to the FILETYPE.
GRAVE: “Servlet.service()” pour la servlet UploadServlet a généré une exception
java.lang.IllegalArgumentException: Incorrect input type!
at javax.imageio.ImageReader.setInput(Unknown Source)
at com.sun.imageio.plugins.png.PNGImageReader.setInput(Unknown Source)
at javax.imageio.ImageReader.setInput(Unknown Source)
at com.delicieuse.UploadServlet.doPost(UploadServlet.java:92)
Here is my code: with the line that has the exception. If you are a Java developer or an HTML5 developer, you could tell be what is the FILE FORMAT. Please.
It seems to be a PNG encoded as a B64. But I can’t make it work.
public static String convertStreamToString(java.io.InputStream is) {
java.util.Scanner s = new java.util.Scanner(is);
return s.hasNext() ? s.next() : “”;
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Part file = request.getPart(“file”);
String filename = getFilename(file);
InputStream filecontent = file.getInputStream();
File outputFile = File.createTempFile(“Img”, “”, new File(“C:\\Temp\\”));
String fileContentStr = convertStreamToString(filecontent);
String base64Image = fileContentStr.split(“,”)[1];
byte[] imageBytes = javax.xml.bind.DatatypeConverter.parseBase64Binary(base64Image);
BufferedImage bigImage = ImageIO.read(new ByteArrayInputStream(imageBytes));
ImageReader reader = ImageIO.getImageReadersByMIMEType(“image/png”).next();
// Configure the input on the ImageReader
ImageInputStream bigInputStream = ImageIO.createImageInputStream(imageBytes);
// THIS LINE RETURNS AN ERROR
reader.setInput(new ByteArrayInputStream(imageBytes));
// Decode the image
BufferedImage image = reader.read(0);
ByteArrayOutputStream os = new ByteArrayOutputStream();
ImageIO.write(image, “jpeg”, os);
InputStream is = new ByteArrayInputStream(os.toByteArray());
ChannelTools.copy(is, outputFile);
long size = file.getSize();
String retMessage = “File ” + filename + ” successfully uploaded. “;
response.setContentType(“text/plain”);
response.setCharacterEncoding(“UTF-8”);
response.getWriter().write(retMessage);
}
Thank you very much
Hi.
Is this working on Safari?
Can you provide an example code?
If you can provide a source code for taking a snapshot and recording a video on Safari, thanks.
Hello dol and everyone,
1 – I finally solve my problem with filetypes.
The PNG image sent by the browser is now correctly taken into account on the Java side.
I did not need to use: reader.setInput(new ByteArrayInputStream(imageBytes)); and I removed this code.
2 – I would also like to have a code that works on SAFARI
I seems it can not work on Safari, but if it is possible, it would be very usefull
Don’t work in safari? can’t load webcam.
Hello david i tried your code but it is giving me this error in the console could you please help me out
Uncaught (in promise) DOMException: The play() request was interrupted by a call to pause().
When i recorder video, how to add text to video rendered?? In
navigator.mediaDevices.getUserMedia
of course.Thanks!
Hey, can you specify whether or not this software can automatically Scan a document in the same way that it snaps a picture. (To avoid having to Scan the document manually into the system).
Are we able to control which camera stream gets returned by
getUserMedia
on laptops with multiple cameras (i.e. Surface Pro 4)?Hi Rodd,
Checking in to see if you ever heard or found an answer to your question here?
Any pointers are highly appreciated.
Thank you.
Hi,
How would you tie this in with a form so that the picture gets submitted along with the other form data?
Hi David
Thanx a lot for great tutorial it`s working fine for me but
After taking snap how to upload it in mysql DB.so can you plz send me a code how to do this thanx in advance
Just a note :
is being deprecated, since it locks the source/device from the MediaStream is originating.
The correct way is
Hi David,
Can I use for two webcam, one for capture my face, one for capture my ID card.
Please advise.
Regards,
Suwandi
Hi David,
I have tested your code on my browsers . . Its working all good on all . . But Why this same code doesn’t work on others devices like Iphone, Ipad etc . . . Is there any fix to resolve this.
Please advice….
Regards,
Sumit
Hi David,
I am able to connect to webcam but my camera is not visible in my web browser. Could you please let me know if I need to do any settings? Its showing the web page is accessing camera but I can’t see my camera on my webpage.
Regards,
Kishore
Heavily simplified. Hats off….
The WebRTC approach restricts you to the low-resolution video stream (often 640×480=0.2Mpix) stream. If you need high-resolution pictures you need to employ the Media Capture API.
You can find a working fiddle for high-resolution pictures with auto-upload in my fiddle: https://jsfiddle.net/rhsb0fqo/
Be aware this API is only supported in Mobile Browsers. If you need it to work for desktop browsers, then you need WebRTC as a fallback.
how would you capture the video and save the video into say post on word press. say if i use this to capture the video from my page then have the video appear below in its own section? giving me the abilities to have a feed of all my recorded videos from page? ad does the get media access mobile device camera? any help would be appreciated I have been to maybe 200 pages and wrote some code with getusermedia that works but I can figure out how to send the capture and place it in a section on my page. and be able to create a new instances of video that will just keep adding above or below it?
Hello David your Camera Demo doesnt work on Chrome or Canary.Any solutions yet?
https://davidwalsh.name/demo/camera.php
Hi david!
I try to convert it into APK using website2apk, Its only show the play logo can’t access the camera. Do you have solutions?
Hi, this is a great achievement and it works fine on my Android Phone running Chrome.
I found this article while looking for a way to start the flashlight on my phone via a web browser.
I wonder if that as well can be achieved?
Hi David
How can I stop the webcam by using a click function, is that possible ?
Thank you! You saved my life and a lot of time
Can you please mention how its works on ipad and iphone
we apply demo code, camra working on laptop and android phone but not working in ipad and iphone
Hi.
One little change I had to make
Replaced
with
Works in both FF and Chrome
Cheers
Hi Manny,
I am very surprised that there a no repsonses on your suggestion to use “video.srcObject = stream;” since that was exactly what got my application working again in both Chrome and FF. Edge was the only browser that did not have the problem, but Chrome and FF suddenly stopped showing the camera-stream.
Since I really did not have any clue on what the cause of this issue was, I was very happy that I found your comment since it solved my problem!
Thanks a lot!!
Hey Daivd,
Thanks for this code, amazing quick solutions.
Can you help me in how to save image into database using php
I found the camera function excellent, but it is possible that it activates the camera of the normal cell phone and not the frontal one?
Change code
mediaConfig
:is this working fine to access main camera?
This code was run.
Change:
With:
How to open mobile back camera, It opening from camera only
hi,all i use ios try run the Demo but it not work anyone could give me some suggestion? thanks
Can I use rear camera of mobile using this API
i can’t to get camera access from chrome browser to live streaming on my mobile. What is the reason. Please tell me about exact code of accessing the mobile camera from browser
Hi David, thanks for your coding , 2nd question is how to switch to front camera video audio and capture
Hello,
Thanks for your post.
Is it available to broadcast multi-webcam?
Hi,
Thank you ver well for your tuto. It’s very simple an helpfull.
So, I have a question, How can we detect if webcam is conected or no before capturing a picture ?
Thank you.
Hi – interesting – if I understand correctly we can take the captured photo and do something with it (e.g. fire it up to a server). Can we also delete (or prevent) the image from remaining on the phone/device?
thanks for any clarity you can provide !
thanks
JO
How do i add a count down timer to the function. So as to allow the user to properly stay in position for a picture?
I am getting:
on this line:
Hello
How can I start and stop a button for this code? In fact, what should be done to stop the camera and save the photo in a separate file?
Thank you for your help
I need to be able to add overlay to the video frame so that they know where to have face to take picture. Looking to end up with a round picture with face in middle.
Cannot seem to be able to overlay on the video frame, can you help?
Please, I need the complete HTML code of a camera that works without internet connection. I do not know enough about HTML, CSS, Java Script so I ask you for the complete code, ready to use, I need it. Thank you very much in advance. I would prefer that you reply to my email.
Hi david,
This works for all browsers except IE11 as
mediadevices.getusermedia()
is not supported in IE11. Any option for this?Windows Camera App error code 0xA00F4244
How do i stop video(web camera) ?
Hi,
How would I get the image url?