Sexy Album Art with MooTools or jQuery
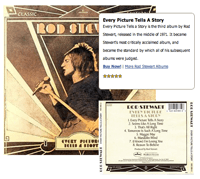
The way that album information displays is usually insanely boring. Music is supposed to be fun and moving, right? Luckily MooTools and jQuery allow us to communicate that creativity on the web.
The XHTML
<div id="album"> <div id="album-front"></div> <div id="album-back"></div> <div id="album-desc"> <h3>Every Picture Tells A Story</h3> <p> Every Picture Tells a Story is the third album by Rod Stewart, released in the middle of 1971. It became Stewart's most critically acclaimed album, and became the standard by which all of his subsequent albums were judged.<br /> <a href="javascript:;" style="font-weight:bold;">Buy Now!</a> | <a href="javascript:;">More Rod Stewart Albums</a> <br /><img src="everypicture-stars.gif" alt="5 Stars!" style="margin-top:10px;" /> </p> </div> </div>
A few structure DIVs and the album information.
The CSS
#album { width:500px; position:relative; } #album-front { width:400px; height:393px; background:url(everypicture-front.png) 0 0 no-repeat; cursor:pointer; position:absolute; top:0; left:0; z-index:1; } #album-back { display:none; width:250px; height:194px; position:absolute; top:250px; left:250px; background:url(everypicture-back.png) 0 0 no-repeat; z-index:3; } #album-desc { display:none; font-size:10px; font-family:tahoma; border:1px solid #7B7057; padding:10px; width:230px; position:absolute; top:20px; left:250px; z-index:3; -webkit-border-radius:8px; -moz-border-radius:8px; border-radius:8px; background:#fff; }
The CSS is used mostly for positioning and the imagery. I chose to incorporate the album images as background images -- you could use IMG tags if you want.
The MooTools JavaScript
(function($) { window.addEvent('domready',function() { //settings var fades = $$('#album-back,#album-desc'); //init fades.setStyles({ opacity: 0, display: 'block' }); //hover version $('album').addEvents({ mouseenter: function() { fades.fade('in'); }, mouseleave: function() { fades.fade('out'); } }); }); })(document.id);
Simple fading in and fading out -- nothing more.
The jQuery JavaScript
$(document).ready(function() { //settings var fades = $('#album-back,#album-desc'); //hover version $('#album').hover(function() { fades.fadeIn(250); }, function() { fades.fadeOut(250); }); });
I think this is a great way to add some dynamism to an otherwise boring display. Rod Stewart FTW!
AWSUM article !! :)
I have to tell that moo transition is smooother than jquery..
That tends to be the case, kburn.
Very nice, thank you!
That was a scary insight into your music tastes David!
Hi David,
Nice stuff! Just one remark, you could use
fades.fade('hide')
instead ofAnd now I wonder, isn’t the default duration of moo 500ms? You probably should make them the same, y?
Gracias por el código y la idea.
Thanks for sharing. Came in really handy.
if i hover many time, it will play non-stop and feel like not good but if i use .stop.fadeIt(250) method and hover many time , it opacity will reduce and eventually wont show, it like disappear, not sure if there any solution, can share?
Thank.
Nice, but how to make description div to apear on ALBUM div or near it? i hate absolute possitions, on every page it’s diferrent so how to make it stick with parent div?