Create a Quick MooTools Slideshow with Preloading Images
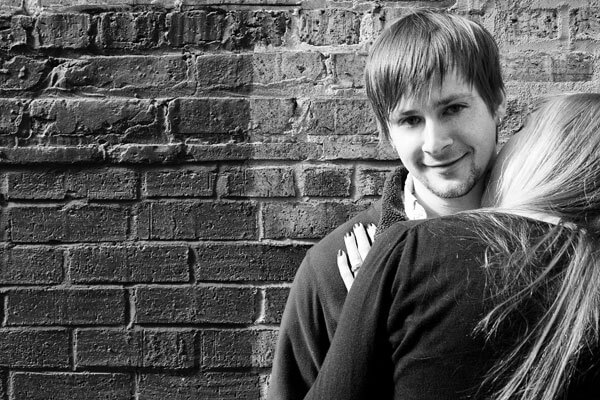
I've been creating a lot of slideshow posts lately. Why, you ask? Because they help me get chicks. A quick formula for you:
var numChicks = $$('.slideshow').length; //simple!
The following code snippet will show you how to create a simple slideshow with MooTools; the script will also preload the images and feature a progress message. Why preload images? They make the slideshow more elegant and you can avoid an onLoad mess. Oh, and chicks...loads and loads of chicks.
The HTML
<div id="slideshow-holder"> <div id="progress"></div> </div>
Basically just two DIVs which will hold content.
The CSS
#slideshow-holder { width:600px; height:400px; background:url(spinner.gif) center center no-repeat #fff; position:relative; } #progress { position:absolute; width:100%; text-align:center; color:#999; top:225px; }
The image holder is given set dimensions, starts with a background spinner, and its position set to relative; the images will all be positioned absolutely. The progress holder is set right below the spinner.
The MooTools JavaScript
window.addEvent('domready',function() { /* preloading */ var imagesDir = 'epics/'; var images = ['2.jpg','3.jpg','1.jpg','4.jpg','5.jpg']; var holder = $('slideshow-holder'); images.each(function(img,i){ images[i] = imagesDir + '' + img; }); //add dir to images var progressTemplate = 'Loading image {x} of ' + images.length; var updateProgress = function(num) { progress.set('text',progressTemplate.replace('{x}',num)); }; var progress = $('progress'); updateProgress('text','0'); var loader = new Asset.images(images, { onProgress: function(c,index) { updateProgress('text',index + 1); }, onComplete: function() { var slides = []; /* put images into page */ images.each(function(im) { slides.push(new Element('img',{ src:im, width: 600, height: 400, styles: { opacity:0, top:0, left:0, position:'absolute', 'z-index': 10 } }).inject(holder)); holder.setStyle('background','url(logo.png) center 80px no-repeat'); }); var showInterval = 5000; var index = 0; progress.set('text','Images loaded. MooTools FTW.'); (function() {slides[index].tween('opacity',1); }).delay(1000); var start = function() { (function() { holder.setStyle('background',''); slides[index].fade(0); ++index; index = (slides[index] ? index : 0); slides[index].fade(1); }).periodical(showInterval); }; /* start the show */ start(); } }); });
The first set of variable declarations represent basic settings for the preloader: images, preload-message-updating, etc. We pass our Asset.images instance an array of images. When each image loads, we update the status message. When every image has loaded, we remove the status message and start the slideshow. That's it!
Of course the above could be turned into the class....I'm just slightly lazy...Feel free to turn it into a class and share with everyone!
Doesn’t that suggest they’re only interested in the length of your slideshows, rather than the number of slideshow posts you make? :P
@Addy Osmani: Having a sizable slideshow doesn’t hurt. Maybe I should have used PHP:
As long as you remember to protect your
$$('.slideshow')
with curly brackets I think you should be fine.You’d hate for some ‘child’ elements to randomly start appearing unexpectedly around your page ;)
Dude, nice photos..! I mean, they are really good!
Especially the purple one!
Back to chicks – redirect guys to Christina Ricci posts so only chicks stay around ;)
I see that you use
this kinda syntax quite a lot.
I mean
(function(){}).xyz()
type of code.Does it have a some real advantage, apart from making code a lil complex to read.
@Nitin: I used that because the “.delay” method is a Function method, thus I need to wrap the “tween” directive in a function.
You just wanted and excuse to show off ur engagement photos!!
Showoff!! LOL
Great job and nice Photos
I would have thought that you could just tell chicks you were a mooTools guru, but slide shows work too!
Nice one!
Any easy way to make the slideshow randomize the pictures so that it will randomize the order on load?
Nice one!
Any easy way to make the slideshow randomize the pictures so that it will randomize the order on load?
Hello David,
i found an issue in your script:
and what kind of mo are you using.
with my original one mootools-1.2.4-core-yc.js from the moo-side
i get the error Asset is not defined.
@hamburger:
Asset is part of mootools.more, go there http://mootools.net/more and download the js with Asset if you need this one only
@David:
I was doing a slideshow with gradient lightbox, and wanted to integrate some ready done mootools slideshow, so I went with this one. Did some refactoring to put it in a class and it works great! Thanks a lot.
My slideshow: http://standupweb.net/mootools/gradientLightbox.php
hello, thanks for you. cool jquery
Hi there,
Excellent slideshow but just wondering if anybody found a way of randomizing the images?
Thanks Gav
hi guys…
anyone know of a way to add images using an external xml file?
thanks
donb
I love this script. However, it doesn’t seem to work on firefox 2 for some reason. unfortunately the project I’m working needs to be compatible with such antiquities.
Hi David
I don’t sure about my issue. It relate to use duration options instead delay function.
What’s diffirence ? performance ? speed ?
How would you use this to click through the images, instead of having them auto-cycle? (i.e. click on image 1 to have it load image 2)
What a cool slideshow!
I was wondering if anyone has been able to add a hyperlink for each image. It would help me a lot.
Thanks!
thank you. i like this slider.
Hi David
Your Diapo is using the Asset.images of Mootools , and I have a question about that considering this following code coming from MooWheel ( here http://labs.unwieldy.net/moowheel/ )
We need here to activate the code
this.parent()(wheelData, ct, options);
at the end of the OnCompleteInspite of the hack with
arguments.callee._parent_
(seems depreciated now !)we don't reach the method of the parent class MooWheel of MooWheel.Remote
How do you think we can do it now ?
Really cool script, g;ad I read the comments, couldn’t get it to work until I included more.js :)
Every day I amaze your detailed examples. You’re perfect. Greetings from Serbia ;)
Your script looks like just what I need… but I can’t get it to work :-(
As nearly as I can tell, it’s not a path issue for the pics. I don’t know if I need Mootools 1.2.4 loaded… I don’t have it, and know nothing about it. Do I need that? Thanx!
Ooooops… never mind, I got it figured out. I just needed to walk away for a few minutes :-)
Desperately need sleep…
Hi, Im new in this page I’d like to know do I have to download something special to make it work? I put the code and I have the .js funtion file but It doesn’t work, can somebody help me ? thanks!