Create a Simple News Scroller Using Dojo
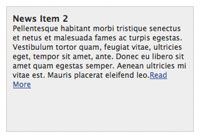
My journey into Dojo JavaScript has been exciting and I'm continuing to learn more as I port MooTools scripts to Dojo. My latest experiment is porting a simple new scroller from MooTools to Dojo. The code is very similar!
The HTML
<div id="news-feed"> <ul> <li><strong style="font-size:14px;">News Item 1</strong><br />Pellentesque habitant morbi...<a href="#">Read More</a></li> <li><strong style="font-size:14px;">News Item 2</strong><br />Pellentesque habitant morbi...<a href="/news/2">Read More</a></li> <!-- more.... --> </ul> </div>
The news items are placed into list items. The UL will be the element that's animated.
The CSS
#news-feed { height:200px; width:300px; overflow:hidden; position:relative; border:1px solid #ccc; background:#eee; } #news-feed ul { position:absolute; top:0; left:0; list-style-type:none; padding:0; margin:0; } #news-feed ul li { min-height:180px; font-size:12px; margin:0; padding:10px; overflow:hidden; }
The absolute positioning is essential to proper animation. Unlike my MooTools example, this example no longer requires a fixed height for each news item. I did add a minimum height so only one item shows up within the scroller window at a time.
The Dojo JavaScript
dojo.require('dojo.NodeList-fx'); dojo.addOnLoad(function() { /* settings */ var list = dojo.query('#news-feed ul'), items = list.query("li"), showDuration = 3000, scrollDuration = 500, scrollTopDuration = 200, index = 0, interval; /* movement */ var start = function() { interval = setInterval(move,showDuration); }; var stop = function() { if(interval) clearInterval(interval); }; var reset = function() { list.anim({ top: 0}, scrollTopDuration, null, start); }; /* action! */ var move = function() { list.anim({ top: (0 - (dojo.coords(items[++index]).t)) }, scrollDuration, null, function(){ if(index == items.length - 1) { index = 0-1; stop(); setTimeout(reset,showDuration); } }); }; /* stop and start during mouseenter, mouseleave */ list.onmouseenter(stop).onmouseleave(start); /* go! */ start(); });
This is where I have the epic description...but the above code (at least for MooTools users) should look familiar. The logic is exactly the same but the code uses dojo.* methods instead of MooTools' Fx, $$, $, and Element.Dimensions methods.
The MooTools JavaScript
window.addEvent('domready',function() { /* settings */ var list = $('news-feed').getFirst('ul'); var items = list.getElements('li'); var showDuration = 3000; var scrollDuration = 500; var index = 0; var height = items[0].getSize().y; /* action func */ var move = function() { list.set('tween',{ duration: scrollDuration, onComplete: function() { if(index == items.length - 1) { index = 0 - 1; list.scrollTo(0,0); } } }).tween('top',0 - (++index * height)); }; /* go! */ window.addEvent('load',function() { move.periodical(showDuration); }); });
The above code was taken from my original post. Take a moment to compare the Dojo and MooTools code.
What do you think? Three Dojo posts in -- what are your thoughts about Dojo to this point?
Hi David,
I’d be interested to see a post from you about the two frameworks – especially the merits & problems of each one.
Very much enjoying this series so far :)
@Jack Franklin: Check out Christoph’s talk to learn a bit more about MooTools: http://davidwalsh.name/mootools-video
Firstly, great article! However, there appears to be a small problem with the script. If the
onmouseenter
event is fired whilst on the last list item, the setTimeout function will already have been queued from the lastmove()
as it i the last in the list. This means that thestop()
function appears not to work. It does stop, only to be restarted by the setTimeout from the last move. Once restarted, things become a little more strange and the events are fired more quickly, almost as if we now have 2 intervals operating independently. Please feel free to contact me if my ramblings are unclear.Browser: Google Chrome 9.0.597.98
Hey, I’ve found removing the
stop()
andsetTimeout()
from the last item callback fixes the issue.Regards
why should i need dojo while I have found an Excellent News Scroller using jQuery and it made my Day !
eeehhhaaaa !
Check this out :
http://codecanyon.net/item/wp-easy-news-scroller/4063270
“WP Easy News Scroller” Plugin is a jquery based wordpress plugin which is used to show the wordpress news or relevant posts with various sliding effects and sixteen (16) different Themes. It can be integrated in the sidebar or footer area on your Blog or Website. WP Easy News Scroller Plugin adds latest news with Title, Image and Description. One can easily install and setup the plugin into the WordPress site.