Input Incrementer and Decrementer with MooTools
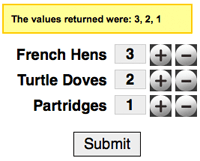
Chris Coyier's CSS-Tricks blog is everything mine isn't. Chris' blog is rock star popular, mine is not. Chris prefers jQuery, I prefer MooTools. Chris does posts with practical solutions, I do posts about stupid video-game like effects. If I want to become a rock star like Chris, I'll need to calm it down a bit and put together something you can all use! Today's post will show you how to add incrementing and decrementing functions to input elements on any form.
The CSS
label { font: bold 20px Helvetica, sans-serif; display: block; float: left; text-align: right; padding: 5px 10px 0 0; width: 140px; } input[type=text] { float: left; width: 40px; font: bold 20px Helvetica, sans-serif; padding: 3px 0 0 0; text-align: center; } form div { overflow: hidden; margin: 0 0 5px 0; } .button { margin: 0 0 0 5px; text-indent: -9999px; cursor: pointer; width: 29px; height: 29px; float: left; text-align: center; background: url(increment-buttons.png) no-repeat; } .dec { background-position: 0 -29px; } #submit { margin: 15px 0 0 95px; font: 20px Helvetica, sans-serif; padding: 5px 10px 3px 10px; border: 1px solid black; background: #eee; } #submit:hover { background: #ccc; }
The most important part of the CSS here is making sure the increment/decrement elements are properly floated and formatted.
The MooTools JavaScript
window.addEvent('domready',function() { $$('form div').each(function(div) { var input = div.getFirst('input'); var upButton = new Element('div',{ text: '+', 'class': 'button', events: { click: function() { input.value = input.value.toInt() + 1; } } }).inject(div); var downButton = new Element('div',{ text: '-', 'class': 'button dec', events: { click:function() { var val = input.value.toInt(); if(val != 0) { input.value = val - 1; } } } }).inject(div); }); });
The first thing we do is grab all of the DIV elements within our form. For each DIV in the form, we inject two DIV elements which serve as the incrementers and decrementers. The newly created incrementer and decrementer have a "click" event assigned to each to which will manipulate the input value.
What I love about this idea (originally Chris Coyier's) is that users without JavaScript don't see the incrementers (since the click events wouldn't work if they did) and the don't interrupt the normal flow of the form.
The jQuery JavaScript
Want to see how to accomplish this feat with jQuery? Check out Chris Coyier's CSS-Tricks blog!
Hopefully this post is a step in the direction of becoming a rock star blogger. Some, however, would say that I've already got the look.
eheh, handy but too late for my needs…
i did have to do this only 4-5 days ago – but by relation. 4 input boxes, all numeric to increment/decrement but with the sum of the values of inputs 2,3,4 to remain less than or equal to the value of input 1. ended up with a piece of code that could be quite useful (including attachable +/- controls etc) – if i could figure how to refactor it in a way that can be reused …
btw, toInt on value “” results in a NaN so trap when users have deleted the values by hand
The test in the minus function should be val >= 0, because with != , if I type -1 in the input and press -, it keeps going down. I know it deos not really matter since the user can keep typing whatever they want, unless you make the input readonly
Anyway for the minus button function, you optimize it a little with Math.max :
I am just nitpicking. You can also set a maximum value as well with the plus button using Math.min
oops, in my previous comment, I meant val >= 1 , not val >= 0
not bad, but those damn ugly + and – buttons gave me such a fright :0
Lol, design fail by Mootools. Requiring quotes around class ftl
@Darkimmortal: I guess…”class” is a reserved word. The alternative would be what? “klass”. I prefer quotes. Also note that JSON requires quotes on everything for this reason.
Don’t worry about being like css-tricks (or any other blog/author), you’re doing just fine on your own.
@cancelbubble: I was just having a little fun! :)
What about holding down a button to increase the value? Also it should get progressively faster