Digg-Style Dynamic Share Widget Using MooTools
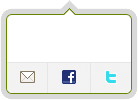
I've always seen Digg as a very progressive website. Digg uses experimental, ajaxified methods for comments and mission-critical functions. One nice touch Digg has added to their website is their hover share widget. Here's how to implement that functionality on your site using MooTools.
The XHTML
<!-- My Custom Story Formatting --> <h2>My Story 1</h2> <p> Mauris amet eu Vestibulum feugiat eget, est. senectus semper. tempor Pellentesque Aenean ante. quam egestas. ultricies fames sit ultricies sit placerat et ac Donec leo. morbi habitant mi eleifend tortor netus turpis libero et amet, tristique malesuada egestas quam, vitae, vitae. Mauris amet eu Vestibulum feugiat eget, est. senectus semper. tempor Pellentesque Aenean ante. quam egestas. ultricies fames sit ultricies sit placerat et ac Donec leo. morbi habitant mi eleifend tortor netus turpis libero et amet, tristique malesuada egestas quam, vitae, vitae. </p> <!-- Share Widget -- Don't Change --> <div class="share-storylist"> <a class="tool share" href="https://davidwalsh.name/demo/digg-share.php?My%20Story%201">Share!</a> <div class="share-hover"> <input type="text" value="https://davidwalsh.name/demo/digg-share.php?My%20Story%201" class="share-diggbar-url" readonly> <ul class="share-actions"> <li class="twitter"> <a href="http://twitter.com/home?status=My%20Story%201:%20https://davidwalsh.name/demo/digg-share.php?My%20Story%201" target="_blank">twitter</a> </li> <li class="email"> <a href="mailto:?subject=My%20Story%201:%20https://davidwalsh.name/demo/digg-share.php?My%20Story%201">email</a> </li> <li class="facebook"> <a href="http://www.facebook.com/sharer.php?u=https://davidwalsh.name/demo/digg-share.php?My%20Story%201" target="_blank">facebook</a> </li> </ul> </div> </div>
You can display the article or post however you'd like but the Digg share widget should closely follow what I've used above, which comes directly from Digg.
The CSS
/* Storylist Share */ .share-storylist { position:relative; float:left; } .share-hover { background:url(storylist-share.png) no-repeat; width:138px; height:106px; position:absolute; top:19px; left:-35px !important; z-index:100; display:none; } .share-diggbar-url { position:absolute; top:24px; left:14px; padding:5px; text-decoration:none; background:#fff; width:98px; border:1px solid #c6c6c6; -moz-border-radius:4px; -webkit-border-radius:4px; } .share-hover span { font-size:85%; font-weight:normal; color:#9ab9d5; display:block; margin-top:-1px; } ul.share actions, ul.share-actions li { list-style:none; } ul.share-actions li a { text-indent:-999px; width:40px; height:26px; position:absolute; top:63px;} .share-actions li.facebook a { left:48px; width:42px;} .share-actions li.email a { left:7px;} .share-actions li.twitter a {left:91px; } /* Storylist Share Email Box */ #share-dialog { display:none; } .share-email a.email-suggestion { background:#edf7e6 url(/img/lightbox-email-apps.png) 460px 7px no-repeat; display:block; margin:-16px -17px 15px; padding:15px 15px 15px 65px; font-size:1.15em; font-weight:bold; text-decoration:none; color:#64a715; border-bottom:1px solid #daecb0; } .share-email a.email-suggestion:hover { color:#000033; } .share-email label { float:left !important; display:block; position:absolute; color:#777; } .share-email input[type="text"] { padding:5px; -moz-border-radius:3px !important; -webkit-border-radius:3px !important; color:#777; margin:0 0 10px 0; width:490px; margin-left:50px; border:1px solid #ccc; font-size:1.1em; } .share-email textarea { width:530px; padding:10px; height:12em; margin:10px 0 5px 0; font-size:100%; background:#fffdea; border:1px solid #dcd069; color:#39340b; } .share-test-email .dialog-tray { text-align:left; }
This is the exact CSS from Digg -- nothing's been changed.
The MooTools JavaScript
(function($){ window.addEvent('domready',function() { $$('a.share').each(function(a){ //containers var storyList = a.getParent(); var shareHover = storyList.getElements('div.share-hover')[0]; shareHover.set('opacity',0); //show/hide a.addEvent('mouseenter',function() { shareHover.setStyle('display','block').fade('in'); }); shareHover.addEvent('mouseleave',function(){ shareHover.fade('out'); }); storyList.addEvent('mouseleave',function() { shareHover.fade('out'); }); }); }); })(document.id);
The JavaScript is a piece of cake using MooTools. Simple show and hide.
One way to improve your website is to check out what the large sites are doing. Feel like you have a lame sharing widget of your own? Duplicate what Digg has done!
You need to set the default opacity to 0 so it fades-in the first time you hover the link.
https://www.sites.google.com/site/gornjamoracainfo/
nice!
interestingly i also found a jquery version :)
Create a Digg-style post sharing tool with jQuery
I updated the code a bit to do minor cleanup and implement Oskar’s suggestion.
Absolutely brilliant! as usual David an excellent post, i admire your work greatly. All the best :)
lovely! thnx
Awesome post, I always enjoy reading these and especially like that you use MooTools.
nice one. may be add
a.stop();
to prevent link on the share texte.great ,u can not believe how much time i spend to find this!
Hi, a great one and already loved by a lot of our cms-customers. We implemented it in the BLOG-function an with the shop-products so everyone can share share share. Thanx David!
You can’t use target=”_blank” for XHTML. :) Please use:
onclick="window.open(this.href); return false;"
instead.On IE this results in nasty black borders during the fade effect because of PNG transparency issues. Is there any way to solve this?
any idea getting around this error ?
Error: uncaught exception: [Exception… “String contains an invalid character” code: “5” nsresult: “0x80530005 (NS_ERROR_DOM_INVALID_CHARACTER_ERR)” location: “http://onlineshopleader.com/bcv/media/system/js/mootools.js Line: 38”]
Sorry, forgot to mention the error above is from FF Error Console
this widget seems to work on mootool 1.2.3 version. i hv mootool 1.12 and couldn’t make it work. it works when i use mootool 1.2.3