Install Chrome Store Web Apps with JavaScript
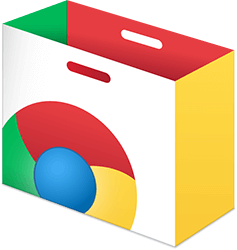
Being able to install Firefox OS web apps from any domain, not just the platform app store, is a giant step forward in mobile app marketing and freedom. Firefox OS allows installing apps from any or all domains, and it just so happens that the Chrome Web Store allows JavaScript-triggered app installation as well.
JavaScript Install Code
The chrome.webstore.install
method accepts three parameters, the install URL, the success callback, and the error callback:
/* Chrome installation */ var chromeInstallUrl = "path/to/chrome/app"; chrome.webstore.install(chromeInstallUrl, function() { // Success! }, function(err) { // Error :( });
The code above triggers installation of the Chrome web app at the given location. The obvious difference from Firefox OS app installation is that callbacks are included in the initial call here, instead of adding adding onsuccess and onerror methods to a resulting install object.