Mobile Touch Events in MooTools 1.3
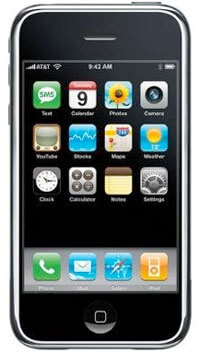
The release of MooTools 1.3 brings about a vast array of new functionality to the JavaScript framework. One big new addition to MooTools Core is the ability to detect mobile events: touchstart
, touchmove
, touchend
, touchcancel
, gesturestart
, gesturechange
, gestureend
, and orientationchange
. Let me show you how to listen for and react to each of these mobile events using the new MooTools touch/gesture functionality with the traditional MooTools event listener syntax!
The MooTools JavaScript
There are four different touch events that may be detected with MooTools: touchstart
, touchmove
, touchend
, and touchcancel
. There are three gesture events which may be detected: gesturestart
, gesturechange
, and gestureend
. You can even detect when the orientation changes with the orientationchange
event! You create mobile event listeners in the traditional MooTools fashion:
//add touchstart event to the body document.body.addEvent('touchstart',function(e) { //react to the touchstart however you'd like! }); //add touchmove event to the body document.body.addEvent('touchmove',function(e) { //react to the touchmove however you'd like! }); //add touchend event to the body document.body.addEvent('touchend',function(e) { //react to the touchend however you'd like! }); //add gesturestart event to the body document.body.addEvent('gesturestart',function(e) { //react to the gesturestart however you'd like! }); //add gesturechange event to the body document.body.addEvent('gesturechange',function(e) { //react to the gesturechange however you'd like! }); //add gestureend event to the body document.body.addEvent('gestureend',function(e) { //react to the gestureend however you'd like! }); //add orientationchange event to the body document.body.addEvent('orientationchange',function(e) { //react to the orientationchange however you'd like! });
The event object looks exactly as any other event object does. You can find the event properties list in the MooTools Event documentation. Here's a quick snippet to listen to all mobile events:
window.addEvent('domready',function() { ['touchstart','touchmove','touchend','touchcancel','gesturestart','gesturechange','gestureend','orientationchange'].each(function(ev) { document.body.addEvent(ev,function(e) { new Element('li',{ html: ev }).inject('mobileEventList','top'); }); }); });
Whenever a mobile event is triggered, a new list item with the event name will be placed at the top of an UL element with the id mobileEventList
.
Where Did You Find the Event Names?
A quick peek at the Element.Event
code provides you a list of every event type MooTools natively supports:
Element.NativeEvents = { click: 2, dblclick: 2, mouseup: 2, mousedown: 2, contextmenu: 2, //mouse buttons mousewheel: 2, DOMMouseScroll: 2, //mouse wheel mouseover: 2, mouseout: 2, mousemove: 2, selectstart: 2, selectend: 2, //mouse movement keydown: 2, keypress: 2, keyup: 2, //keyboard orientationchange: 2, // mobile touchstart: 2, touchmove: 2, touchend: 2, touchcancel: 2, // touch gesturestart: 2, gesturechange: 2, gestureend: 2, // gesture focus: 2, blur: 2, change: 2, reset: 2, select: 2, submit: 2, //form elements load: 2, unload: 1, beforeunload: 2, resize: 1, move: 1, DOMContentLoaded: 1, readystatechange: 1, //window error: 1, abort: 1, scroll: 1 //misc };
As you can see, the new touch and gesture events are listed with the traditional browser events!
MooTools Mobile
Obviously you cannot easily build a mobile application from simple and gesture touch events as shown above. How do you know which direction the user swiped? How can you detect if the user pinched? Have no fear: MooTools Core Developer Christoph Pojer has created MooTools Mobile, a set of MooTools classes providing you more information about touches, swipes, pinches, and mobile browser features! Look for future blog posts from Christoph on this blog about MooTools Mobile and how you can use it on your mobile website!
Thanks, good info. I’ll play around with this later.
Too bad it doesn’t work on my crappy palm pre (like most mobile javascript frameworks). :-/
How do you test this if you don’t have the correct (or any) mobile device? I like the concept…
I’d say mootools needs to fully support mobile web apps development. what I mean by fully support is having something like jquery-mobile.
Saying mootools is a great framework that is generic and allows mobile development is not enough. if the mootools team wants people to develop mobile web apps with mootools there needs to be a customized set of components/classes etc for that in order to gain audience. I thought mootouch was coming but it looks like its not going to happen. (they had a momentum scrolling demo that blew jqtouch away at the time…)
just my opinion.
How do I add this to my moomenu? I’m not very good with Javescript, but i want my site to be supported by mobile devices.
Hello,
With MooTools Mobile is the touchhold example a good starting point for drag and drop functionality? Just wondering if Christoph’s mobile library can handle drag & drop?
Thanks,
Joshk
In Mootools Mobile events are properly working in windows phone 7?