Create a Simple News Scroller Using MooTools, Part I: The Basics
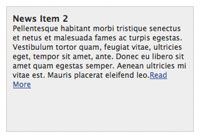
News scroller have been around forever on the internet. Why? Because they're usually classy and effective. Over the next few weeks, we'll be taking a simple scroller and making it into a flexible, portable class. We have to crawl before we walk though; let's make a simple news scroller using MooTools.
The HTML
<div id="news-feed"> <ul> <li><strong style="font-size:14px;">News Item 1</strong><br />Pellentesque habitant morbi...<a href="#">Read More</a></li> <li><strong style="font-size:14px;">News Item 2</strong><br />Pellentesque habitant morbi...<a href="/news/2">Read More</a></li> <!-- more.... --> </ul> </div>
The HTML part is fairly simple: a list with numerous list items (news items) wrapped in a single DIV.
The CSS
#news-feed { height:200px; width:300px; overflow:hidden; position:relative; border:1px solid #ccc; background:#eee; } #news-feed ul { position:absolute; top:0; left:0; list-style-type:none; padding:0; margin:0; } #news-feed ul li { height:180px; font-size:12px; margin:0; padding:10px; overflow:hidden; }
Getting the CSS correct is very important for our simple scroller. The wrapper DIV must be positioned relative and the UL must be positioned absolutely. Each LI's height + padding/margin/border must be the same height as the UL itself.
The MooTools JavaScript
window.addEvent('domready',function() { /* settings */ var list = $('news-feed').getFirst('ul'); var items = list.getElements('li'); var showDuration = 3000; var scrollDuration = 500; var index = 0; var height = items[0].getSize().y; /* action func */ var move = function() { list.set('tween',{ duration: scrollDuration, onComplete: function() { if(index == items.length - 1) { index = 0 - 1; list.scrollTo(0,0); } } }).tween('top',0 - (++index * height)); }; /* go! */ window.addEvent('load',function() { move.periodical(showDuration); }); });
The settings will be placed at the top of the code block, as always. Once the settings are defined, a function is created that scrolls from the current news item to the next news item. Once the scroller reaches the last news item, it scrolls back to the first one. Lastly, when the page has loaded, the directive to start the news scroller is given.
Creating a basic scroller is super simple. Look forward to future posts expanding the capabilities of our scroller, including creating a class and adding events.
Continue, David… ;)
It suggest you stop the event when mouseover the item ^_^ , other than that… beautiful!!! hehe
Can you please write up a JQuery equivalent?
One thing I dislike about looping carousel scrollers like this is the big scroll it makes when it goes from Item 9 back to Item 1. I suspect that it might be possible to circumvent that scroll by duplicating Item 1 next to Item 9, and then when it switches to the duplicated Item 1 (with a normal scroll), you replace the position with the original Item 1 (no animation). Seem possible?
@Jay:
Take a look at an article I wrote some time ago. This hasn’t the big scroll at the end of the list…
http://youngdutchdesign.com/mootools-12-cvnewsticker-class
@Gabriel: Will keep in mind for the next post.
@Brian Lang: Possibly.
@Jay: Yep, I’ll add that to the list.
@David and Jay
You might achieve that by cloning the UL, so you have 2 UL’s in the DIV
Then, you can scroll nicely from 9 to 1… when you’re at #1 again, you put the first UL after the cloned one, and so on.
@Arian: Continued cloning sounds inefficient. Should be able to just do a setStyle.
@David
I didn’t meant continued cloning, but clone the UL once, and use setStyle, .inject(el,’after’) or something like you already mentioned
I wish you see the jquery cycle? because its the same is and very easy
thanks david!
can you make another demo when the direction is down, left, or right?
thanks.
Nice work David!! Amazing like always. Just one thing.. dont work on IE8.
I been looking for a rotator effect, like a wheel. Exist something like that in mootools?? (sorry my bad english)
Thanks again!
Works for me in IE8. What problem are you seeing?
Now its working.. sometimes when I refresh the web, dont work or suddenly start… well.. I think could be the “amazing” browser.
And about the wheel efect? some idea???
Thanks!!!!
Yes, I got the same with you, I must refresh the web , it will works, but sometimes it doesnt work or suddenly start In IE 8 and IE 9.But it alway works in other browsers.
As always great job. Can you give me hint how to add mouse over stop the event.
Is there any way to make it scroll on horizontal way, or the other way around like vertical? I guess this might be great for a featured content scroller. Thanks for sharing!
I M just a graphic designer with not too much programming knowledge, how this works? is this a simple copy paste that I can use in my dreamweaver? is that easy?
Thanks a lot David
Cesar
@cesar delgado: its MooTools.
Good job!! I’m trying to implement this efect in a website, but I’would like that news where continuously scrolling in circles instead of going up to the first item. Also, it’s would be a good idea that the efect stop on mouseover. Sorry for mistakes, but I’m not English!.
Thanks a lot.
Hi, David nice work
but I tried many times to copy your code it still does not work in IE8
:~(
Hey David,
I would second tuxfede’s requests of a “circular” scroller and a pause with onMouseEnter.
If I figure it out, I’ll post it here!
Thanks,
Chris
The script don’t works!
See here and help me:
http://andreahomepage.altervista.org/slide-news/index.php
It doesn’t work… look and hel me, please!
http://andreahomepage.altervista.org/slide-news/
@Andrea: your link works for me… try to crtl+f5
Yep, i change thed js code with the dojo!! thank again :)
Hi David
Having some problems with this one, it doesnt seem to work in IE 8, it just does nothing, thats the code from this page and mootools 1.2.4,
works in chrome etc
really like the no nonsence approach of this and any help would be appreciated :)
thanks in advance
James
Hi David,
Testing this on a joomla site and I’m getting a “String contains an invalid character” code: “5” error? I’m guessing this is something to do with my mootools version (1.12) but I may be wrong?
Also hate to be demanding with all the help on here but I was wondering how would I make the news items fade one to the next rather than slide?
Thanks
Gary
Hi David,
could you try to open the url (http://davidwalsh.name/dw-content/news-scroller.php) In IE8, Maybe something could be found. It doesnt work utill you refresh the web.
How can I pause on MouseOver?
what does it take to implement the fade instead of the scroolTo
I have found a nice News Scroller using jQuery and it made my Day ! eeehhhaaaa !
Check this below:
http://codecanyon.net/item/wp-easy-news-scroller/4063270
“WP Easy News Scroller” Plugin is a jquery based wordpress plugin which is used to show the wordpress news or relevant posts with various sliding effects and sixteen (16) different Themes. It can be integrated in the sidebar or footer area on your Blog or Website. WP Easy News Scroller Plugin adds latest news with Title, Image and Description. One can easily install and setup the plugin into the WordPress site.