Get a URL’s Reddit Score Using PHP and JSON
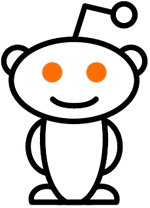
Since we can see Digg turning more into a funny-pic-and-vid site each day, I've turned my attention to Reddit. Reddit just seems more controlled and programmer-friendly. Since I have respect for Reddit I thought I'd put together a quick tutorial on how you can retrieve a URL's Reddit score using PHP.
The PHP
<?php /* settings */ $url = 'https://davidwalsh.name/9-signs-not-to-hire-that-web-guy'; $reddit_url = 'http://www.reddit.com/api/info.{format}?url='.$url; $format = 'json'; //use XML if you'd like...JSON FTW! $score = $ups = $downs = 0; //initialize /* action */ $content = get_url(str_replace('{format}',$format,$reddit_url)); //again, can be xml or json if($content) { if($format == 'json') { $json = json_decode($content,true); foreach($json['data']['children'] as $child) { // we want all children for this example $ups+= (int) $child['data']['ups']; $downs+= (int) $child['data']['downs']; //$score+= (int) $child['data']['score']; //if you just want to grab the score directly } $score = $ups - $downs; } } /* output */ echo "Ups: $ups<br />"; //21 echo "Downs: $downs<br />"; //8 echo "Score: $score<br />"; //13 /* utility function: go get it! */ function get_url($url) { $ch = curl_init(); curl_setopt($ch,CURLOPT_URL,$url); curl_setopt($ch,CURLOPT_RETURNTRANSFER,1); curl_setopt($ch,CURLOPT_CONNECTTIMEOUT,1); $content = curl_exec($ch); curl_close($ch); return $content; } ?>
Parsing the JSON is simple using json_encode with the value of true to make turn the JSON into an associate array. My example shows how you can grab the number of "ups" and "downs" -- not just the score. As with every API/web service, I highly recommend caching the result of your request.
David, this doesn’t have anything to do with the article (which I’m sure is good but my JSON knowledge is null)… but man that top bar that follows is really funky. In my opinion, it’s distracting to the viewer who just scrolled a little and it follows but also makes it blurry up top. Now when you mouse over it, it’s great and really functional… but I don’t know if it needs to follow me as I scroll down. It reminds me of a frame but it’s even more distracting because of the aforementioned blur.
But of course that’s just me. I also loved the old bottom nav that had little icons next to AJAX, PHP, etc. Like Stewie from Family Guy once said, “I don’t like change!” … especially when it’s a distraction to the main content. But I’m sure there’ll be a lot more iterations of the site (since I’m assuming this is your playground for cool new features… and as I say that, a little cartoon David Walsh popsup as I highlight a word on the page…) so I look forward to what you come up with next =)
very good, thanks David
You know your script wont inject itself?
@Chris the Developer: Ummm, come again? Looks good to me.
I just means that you could save on the str_replace by using string variable substitution supported by php…
$a = ‘foo’;
$b = “hello {$a}”;
David,
could you provide a workaround because of the great topbar??? I really like it!
@Chris the Developer: Oh. I like my mini templating system. A minor detail/preference, in my opinion.
‘http://www.reddit.com/api/info.{format}?url={url}’ :P
@Chris the Developer: Damnit, you got me! :)
reddit reddit reddit. oh david, you’re such a whore.
@Al: Thank you, sir.
urlencode($url) before appending it to the reddit url, or it will break on URLs that contain query string parameters of their own, among other things.
Just to add my 2 cents, it could also be done like so.
but as you stated before, it’s personal preference and I like coming here for tips. ;) Keep up the good articles!
If I use the format as XML, it doesn’t show me the score or ups & down. Any help !
And how to insert a new post on reddit? Some one have a script?
With this code I get score of http://google.com at 28 score.
But if I check from https://www.reddit.com/buttonlite.js?i=0&url=http://google.com it is showing 128 score.
Why is it difference?