iPad Detection Using JavaScript or PHP
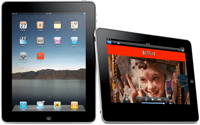
The hottest device out there right now seems to be the iPad. iPad this, iPad that, iPod your mom. I'm underwhelmed with the device but that doesn't mean I shouldn't try to account for such devices on the websites I create. In Apple's developer tip sheet they provide the iPad's user agent string:
Mozilla/5.0 (iPad; U; CPU OS 3_2 like Mac OS X; en-us) AppleWebKit/531.21.10 (KHTML, like Gecko) Version/4.0.4 Mobile/7B334b Safari/531.21.10
Given that string we can create a few code snippets to determine if the user is being a smug, iPad-using bastard.
The JavaScript
// For use within normal web clients var isiPad = navigator.userAgent.match(/iPad/i) != null; // For use within iPad developer UIWebView // Thanks to Andrew Hedges! var ua = navigator.userAgent; var isiPad = /iPad/i.test(ua) || /iPhone OS 3_1_2/i.test(ua) || /iPhone OS 3_2_2/i.test(ua);
A quick String.match regular expression test can check for the presence of "iPad" in the user agent string.
The PHP
$isiPad = (bool) strpos($_SERVER['HTTP_USER_AGENT'],'iPad');
This time we look for the position of "iPad" in the user agent string.
The .htaccess
RewriteCond %{HTTP_USER_AGENT} ^.*iPad.*$ RewriteRule ^(.*)$ http://ipad.yourdomain.com [R=301]
Using some logic from Drew Douglass' excellent mobile redirection post, we can redirect users to a mobile version of your website if you so desire.
So what would you the above tests for? You may want to redirect iPad users to a different version of your website. You may want to implement different styles to your standard website if your user is surfing on an iPad.
Nice tutorial. Did you get an iPad? I also created a similar tutorial
http://cardonadesigns.com/wordpress/2010/04/04/using-php-to-detect-the-ipad-user-agent/
I just wrote a similar post, and referred your post.
As I described the technique in the post, it’s better to apply different CSS to iPad visitors rather than redirecting them to a special version of your website (when it meets your requirements). This way all you need to do is manage different CSS files.
http://jquery-howto.blogspot.com/2010/10/javascript-to-detect-ipad-visitors.html
I respectfully disagree. Especially if your site has flash, more than a one column layout, or really bandwidth intensive content.
Standard web and mobile web are completely different beasts. Simple css tweaks may not be enough.
I applaud the idea of keeping maintenance easy but I think anyone who is even thinking of doing a mobile site, will have to prioritize which content to show.
The cruddy thing about the iPad user agent string is that it has the word “mobile” in it. So if your detection script is looking for “mobile” already, it may also be sending iPad users to your iphone/mobile optimized site. Apple offered up a way to test though -> http://developer.apple.com/safari/library/technotes/tn2010/tn2262.html
Yes but you could employ logic in both cases which does and does not find the string “iPad”.
Great! Now I can redirect/block iPad users!
@Rob: That’s what I was thinking! Thanks David.
@Rob: That’s what I was thinking! Thanks David.
I just use this code:
What if I need a different landing site for the ipad, I try this and it doesn´t work
if((navigator.userAgent.match(/iPhone/i)) || (navigator.userAgent.match(/iPod/i)))
{
location.replace(“iphone/index.html”);
}
if((navigator.userAgent.match(/iPad/i)))
{
location.replace(“ipad/index.html”);
}
else
{
location.replace(“index_frames.html”);
}
Thanks
Maybe try location.href I’ve never used location.replace.
@Connor Crosby: that is in a javascript
Another, and often better option is media queries in CSS3
http://www.w3.org/TR/css3-mediaqueries/
You can deliver CSS based on device characteristics, such as viewport width, and orientation (so you can have different CSS for portrait and landscape)
http://developer.apple.com/safari/library/documentation/AppleApplications/Reference/SafariWebContent/OptimizingforSafarioniPhone/OptimizingforSafarioniPhone.html
Let them eat Flash
Welcome to the old web !
…
“Site optimised for IE 5.5”
But where is the code to make the iPad melt once detected? :p
@Noetic: you could start with js “while……..” =x
Very nice tutorial, tnx man!
V.
I was a little stuck. I’m looking to do something similar, but really just target the viewport for the iPhone/iPod Touch and iPad. Here’s what I came up with so far but doesn’t seem to work. Any suggestions?
Easy to do redirecting in PHP:
If you just want to redirect iPhone users:
If you also want to bounce iPad users, use this instead:
Here’s what the session variable “fullsite” is for:
I always use a link in the mobile site that would allow the user to go to the full site if he wishes; the link goes to a page I call “fullsite.php,” and which includes this code:
This sets the session variable and then sends them back to the full site’s home page, and prevents the PHP from bouncing them continually back into the mobile site.
Note that the session variable will stay set until they close their browser.
Hey ! Thanks for the turorial !
I’m a newbie, and I wanna do something I guess is easy, but can’t find a solution.
What I wanna do is to display a certain element (a div) if the user is on his computer, but display another one instead if he is on his iPad.
What should I write, and where ????
Please help me !
Charles
This post is featured on 40 iPad tools, tips for designer
Perfect! thank you David!
Hi! Thanks for the article.
I wrote a free open source set of APIs for web designers to detect mobile visitors. The APIs are very light weight, easy to implement, and easily customized. They’re available for JavaScript, PHP, Java, and ASP.NET. Check out http://www.mobileesp.org
Nice article, thanks.
Also nice site and article on iPad site dev Garmahis. Particularly enjoyed the link to page flipping versus scrolling. :)
Thank you very much for this page! I probably owe you a cut of the small fee I earned last night solving someone’s emergency iPod detection crisis. And you’re funny! (lots of people can code. Funny, not so much.)
Did you know that its not possible to copypaste you hillarious quotes when using An ipad to reading you site?
“determine if the user is being a smug, iPad-using bastard”
Genius!
Hate the bastards myself. It’s the iPhone all over again: “You didn’t invent it, you bought it, anyone can buy a bloody iPad”
Sorry for posting again in such quick succession, but I’ve just found a very natty PHP class that handles all browser user agents, including the iPad. Thought you might be interested:
http://www.phpclasses.org/package/6369-PHP-Detect-the-type-of-browser-accessing-the-site.html
Promise I’m not spamming!
Nice Article. The regexp works great for single device detections. If you’re after detecting device classes like Tablets or other mobile browsers check out http://www.handsetdetection.com (disclaimer : I work there).
Cheers
Richard
Awesome tutorial! Saved me for iPad in my use
Nice way to check, but I am waiting to buy iPad.
thank you for info
As of iOS 3.2 (which is iPad-only), the user agent string no longer contains ‘iPad’. Rather, the device identifies itself as ‘iPhone’, so the above solution does not work. The way I’m detecting iPads in JavaScript–at least until I come across a better way–is with the following expression:
/iPad/i.test(ua) || /iPhone OS 3_1_2/i.test(ua) || /iPhone OS 3_2_2/i.test(ua)
Sorry, the ‘ua’ in that line of code should be navigator.userAgent
When is/was iOS 3.2 released? I just tested on my iPad, which has its iOS up to date, and the user agent included “iPad”.
http://davidwalsh.name/dw-content/detect-ipad-new.php
I’m seeing iPad in the UA for Safari on iPad as well. I think the case I was seeing was an embedded UIWebView inside an iPad app. Most web developers won’t have to worry about that case, I suppose, but it is pretty vital for native app developers using web views.
Awesome, thanks Andrew. I updated my article. Cheers!
Laughed my ass off on the ‘iPad this, iPad that, iPad your mom’. hahaha! Made my day!!!
To those having issues like “too many redirections” there’s a solution, here’s the link of StackOverflow where this guy helped me figure out a easy solution to this!
http://stackoverflow.com/questions/5131158/ipad-iphone-browser-sniffer-with-mod-rewrite-and-redirection-too-many-redirecti
Hey, becareful with this line:
PHP’s
strpos()
can return0
, meaning that the$needle
is at position 0 (zero) of the string. So if you do like that,$isiPad
can returnFALSE
where the correct value isTRUE
.To resolve that, do this:
:)
That is true in general, but in the case of the user-agent string for iPad it never puts iPad at the start of the string…
Palomita para tí, awesome tutorial, simply! efficient! Thanks a lot.
Thanks for the code snippets David…! Thanks for Andrew too.
Surely there must be a better way than using userAgent…
At the end of March 2011, there are literally HUNDREDS of possible values for the userAgent, all of which have to be manually mapped as “true” or “false” as to whether they are mobile devices.
In the coming months and years, that number could easily become THOUSANDS!!!
This is a regression to web-dev practices of the 1990s.
As developers, we cannot rely on such tactics…
Simply including such a file means users have to download SEVERAL THOUSAND ADDITIONAL BYTES just to determine if they are mobile or not.
Maybe we should be identifying screen resolutions or something “simpler”…
@Blunt Brother – someone already commented on this – see CSS3 media queries. I agree with you by the way – user agent sniffing everything is a big regression. We’d just about, almost FINALLY got Microsoft to fix IE and the desktop browser space is better than it’s ever been for web developers, so now everyone wants to bend over for mobile devices? Insane.
How about for ipad 2?
Awesome post that I was looking for. Although a suggestion : the code with black background is hard to read i had to copy it and read it .
Thanks again
Anyone here had experience detecting iPad then providing a specific version of a video within the page to only thos ipad users, allowing for stack overflow to take care of other safari, ff, and ie users. The reason i need to do this is to overcome ipad 2’s gamma problem when playing video. I need to provide a gamma adjusted version of the video just to ipad users (preferably just ipad2 but i dont think the user agent string is that specific?,
Ideally just JS, and Im no JS wizard.
Any help would be greatly appreciated.
Just wanted to share this, since it pertains to the topic.
Our hero image once clicked opens a pop-up/shadowbox image viewer (imaging provided by Adobe Scene 7) on our company website, but (non-Flash-i)Pads don’t dig it. We use Miva Merchant as a part of our backend. The following code, using Miva Script, allows us to detect iPads and reveal a compatible viewer alternately:
-------- MOBILE USER AGENT --------
-------- MOBILE S7 VIEWER --------
-------- MOBILE ENLARGE VIEWS BUTTON --------
-------- NORMAL USER AGENT --------
-------- PRODUCT IMAGE --------
-------- ENLARGE VIEWS BUTTON --------
Essentially, if the site detects a mobile device, show the alternative viewer. Else, reveal the normal one.
Thanks for the insight thus far.
A fourth method are ESI tags like:
http://esi-examples.akamai.com/viewsource/ad.html
This is required when using a server side templating system that renders first before JavaScript
the php code is not working anymore.. :( help anyone?
This post is missing what’s by far the best check for an iPad (or iPhone) in JavaScript:
navigator.platform === 'iPad' || navigator.platform === 'iPhone'
The best part is that the platform property can’t be easily spoofed. Some Android devices, for example, will allow users to change the user agent string to match that of an iPad, but changing it won’t affect the value of navigator.platform.
You can do it in PHP:
http://sjevsejev.blogspot.com/2012/05/php-mobile-detect-class-ipad-iphone.html
I advise you check out http://wurfl.io/
In a nutshell, if you import a tiny JS file:
you will be left with a JSON object that looks like:
(that’s assuming you are using a Nexus 7, of course) and you will be able to do things like:
if(WURFL.form_factor == “Tablet”){
//dostuff();
}
The service also goes out of its way to detect specific iPad models.
I know this is an old post but it turns up near the top of a google search for php detecting ipad or something like that. Anyway I just wanted to note that your casting the result of strpos to a boolean is a bad idea. This is because strpos can return a 0 position which simply means the string you are looking for is at the beginning of the string you are searching. When you then cast this to a boolean you get false, implying that the string didn’t contain the search term when in fact it did. The best practice use of strpos to check for the presence of a search term would in fact be
This works because a treble character equality (or inequality) test checks both the type and the value.
navigator.userAgent.match(/iPad/i) not work with Ipad Pro
Since iOS 13 the iPad sends out the exact same User Agent string as desktop Safari, so one has to use hacky client-side solutions to determine if it’s an iPad – the old solutions just apply until and including iOS 12!