Sexy Opacity Animation with MooTools or jQuery
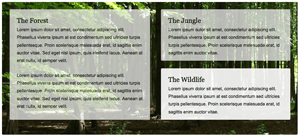
A big part of the sexiness that is Apple software is Apple's use of opacity. Like seemingly every other Apple user interface technique, it needs to be ported to the web (</fanboy>). I've put together an example of a sexy opacity animation technique which you may implement in jQuery or MooTools.
The XHTML
<div id="forest-slot-1" class="opacity"> <h2>The Forest</h2> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus viverra ipsum at est condimentum sed ultricies turpis pellentesque. Proin scelerisque malesuada erat, id sagittis enim auctor vitae. Sed eget nisl ipsum, quis eleifend lacus. Aenean at erat nulla, id semper velit.</p> </div> <div id="forest-slot-2" class="opacity"> <h2>The Jungle</h2> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus viverra ipsum at est condimentum sed ultricies turpis pellentesque. Proin scelerisque malesuada erat, id sagittis enim auctor vitae.</p> </div> <div id="forest-slot-3" class="opacity"> <h2>The Wildlife</h2> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus viverra ipsum at est condimentum sed ultricies turpis pellentesque. Proin scelerisque malesuada erat, id sagittis enim auctor vitae.</p> </div>
There's nothing too special about the HTML above; the awesomeness comes with CSS.
The CSS
/* all css is specific to THIS example */ #forest { width:800px; height:360px; position:relative; background:url(forest.jpg) 0 bottom no-repeat; -webkit-border-radius:3px; -moz-border-radius:3px; border-radius:3px; } .opacity { background:#fff; -webkit-border-radius:3px; -moz-border-radius:3px; border-radius:3px; padding:20px; } #forest-slot-1 { height:260px; position:absolute; top:20px; left:20px; width:340px; } #forest-slot-2 { height:100px; position:absolute; top:20px; right:20px; width:310px; } #forest-slot-3 { height:100px; position:absolute; top:180px; right:20px; width:310px; }
To enhance the design of my content block, I'm adding a colorful background image behind where the content blocks will display. To increase the sexiness, I've added rounded corners to the DIV elements.
The MooTools JavaScript
(function($){ window.addEvent('domready',function() { //element collection and settings var opacity = 0.7, toOpacity = 0.9; //set opacity ASAP and events $$('div.opacity').set('opacity',opacity).addEvents({ mouseenter: function() { this.tween('opacity',toOpacity); }, mouseleave: function() { this.tween('opacity',opacity); } }); }); })(document.id);
The structure for both the jQuery version and MooTools version is the same. Once the DOM is ready, we immediately set the opacity of the child elements to the lighter opacity. We then add mouseenter
and mouseleave
events to the child elements that animate the opacity.
The jQuery JavaScript
$(document).ready(function() { //settings var opacity = 0.7, toOpacity = 0.9, duration = 250; //set opacity ASAP and events $('.opacity').css('opacity',opacity).hover(function() { $(this).fadeTo(duration,toOpacity); }, function() { $(this).fadeTo(duration,opacity); } ); });
Bring the sexy back with jQuery or MooTools and element opacity animation!
Oh, I like this … very nice!
The only downside that I can see about this method is that all children of the div.opacity elements will have the opacity applied to it. I think it would probably be better (in some situations) to have stack two div’s like so:
Then for the opacity effect, use what you already have. Then in addition, place the content of
div.class
on top of div.opacity by setting the positioning to relative and then offsetting the top spacing the height ofdiv.opacity
. It would be cool to have a javascript snippet that finds the height of div.opacity and then applies it to the offset ofdiv.content
.Just sharing…I ran into this exact situation recently.
Simple but nice. I have a question though – why is the domready event handler in the Mootools version wrapped in:
@Jeffrey Schrab: Check this out: http://mootools.net/blog/2009/06/22/the-dollar-safe-mode/
Interesting side effect: If you move over two of the divs fast back and forth in the jQuery example nothing happens, in mootools a chain is build and executed. I’d prefer the second.
@Patrick: You got the two mixed up, in the mootools example, nothing happens, but in the jQuery example a chain is built up… I gotta say I prefer the mootools method.
He needs to add
.stop()
commands before he animates the opacity. That way it will stop all queued animations before it starts a new one, preventing a buildup.That’s a really nice effect, I like it! Thanks David.
@Patrick && @Adriaan
Mootools uses link:cancel, but jQuery uses something like link:chain like Adriaan correctly says.
@Patrick && @Adriaan && @Alex: You can achieve the same effect as Mootools using jQuery. The only thing u need to do is add
stop()
before calling an animation method. This will stop any animation running before firing a new one.Here is the code with this issue fixed:
Or in CSS3 only :
@Jeferson Koslowski: Nice one!
Nice one!
But i got some questions on your example:
1. why do you use tween(‘opacity’, opacity) ? wouldn’t fade(opacity) be easier?
2. do both animations have the same duration?
it would be better to compare if this were so, at this time it seems to me that the jQuery one is faster and for this the mootools example appears to be (maybe it just is) so much smoother, so comparing this two demos for me the mootools (or should i say smoothtools ) example is a clear winner.
good and very useful!
10x
@derschreckliche: I agree with u on the second question. Looks like Mootools has a default of 500ms and the jQuery example is using a duration of 250ms. Thats why Mootools seems smoother than jQuery on this example.
tnx for doing these in both mootools and JQuery! It is much appreciated!!
Not working in Firefox 3.0 or 3.5 / OS X 10.4 (although it works in Safari 4, same OS)
Works for me.
Hahaha! Good but Not Sexy
You can see a similar effect, though not quite as subtle, on my site at http://pulseblogger.com
Maybe I could get some feedback?
@Patrick DeVivo: Looks good, but that right ad bar bouncing around ensures that I’ll never come back again :) Seriously, stop that thing from moving. I know that I can click to station it, but why make the default annoying? If anything, get rid of the bounce and have it scroll down.
@David Walsh : OK, good point. I have had some complaints about it. The only reason i have it followsing your scrolling is to occupy that otherwise empty “sidebar space” on the right side of the site. I will get rid of the bouncing and hopefully you will come back again :)
@Michael: it’s called “dollar safe mode” – used to be able to use mootools with other frameworks.
look here:
http://mootools.net/blog/2009/06/22/the-dollar-safe-mode/
If you mouse in and out fast a few times the animation keeps going on and off even if the mouse is out.
Always remember to stop animations! :D
Nice tutorial.
been a useful effect by using basical knowledge of jquery/mootools
Great tutorial. Thanks!
Thanks for this great tutorial. Really simple and the final outcome is superb!
Pretty neat effect and I have used a similar one in the past!
This does not work in Internet Explorer for me. FF looks great.
when mouse over and read
can it stay effect 0.9?